JavaScript for Beginners: Interactive Web Development Essentials
Last Updated: June 5th 2024
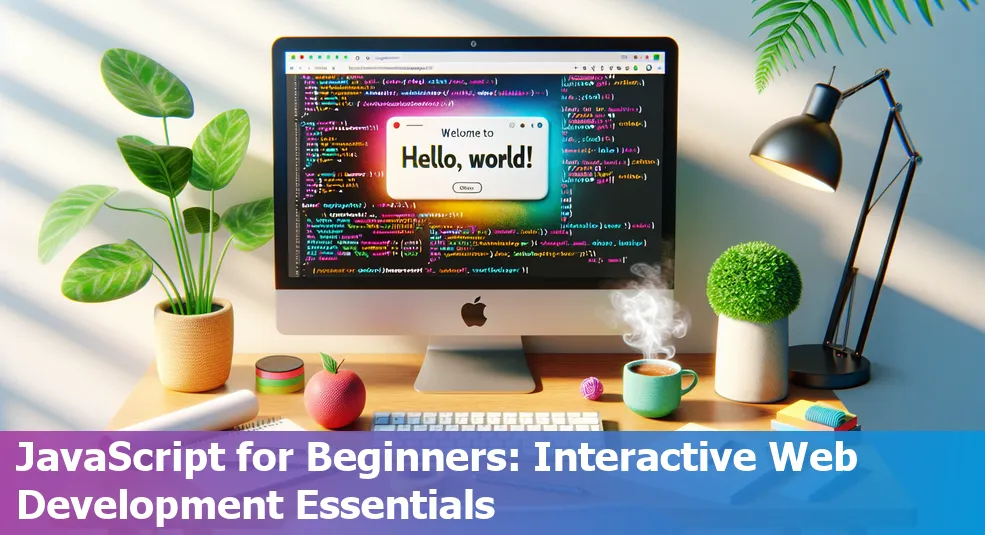
Too Long; Didn't Read:
JavaScript, powering 97.7% of websites, enables rich interfaces, real-time updates, SPAs, and user engagement, increasing interaction up to 70%. Master JavaScript's basic concepts, DOM manipulation, interactive implementations, frameworks like React and Vue.js, common pitfalls, and career opportunities for dynamic web development success.
Check this out! JavaScript is like the superhero of web programming.
It's the secret sauce that makes websites dope and interactive. Almost every website out there is using JavaScript to make things pop off the screen.
With JavaScript, you can do some seriously cool stuff like:
- Make slick interfaces with features like drag-and-drop and content that updates without reloading the page.
- Connect with APIs to pull in fresh data and make the user experience lit.
- Build single-page apps where the content loads dynamically without constantly refreshing the page.
- Validate forms on the fly, so you don't have to wait for the server to tell you if you messed up.
JavaScript isn't just about functionality, though.
It's a game-changer for keeping users engaged and hooked on your site. Sites with interactive JavaScript elements can see up to a 70% boost in user interaction, according to the Nielsen Norman Group.
Mozilla's JavaScript docs break it down – interactive sites are just more fun to use.
Whether you're learning the basics or diving deep into front-end web dev, JavaScript is the language you can't ignore.
It's the secret weapon for creating websites that people actually want to stick around and use.
Table of Contents
- Basic Concepts of JavaScript
- Implementing JavaScript for Interactive Web Development
- JavaScript Frameworks and Libraries
- Common JavaScript Pitfalls and How to Avoid Them
- Conclusion
- Frequently Asked Questions
Check out next:
Achieve Front-End Web Development Mastery by learning how to seamlessly integrate HTML, CSS, and JavaScript into your projects.
Basic Concepts of JavaScript
(Up)If you wanna be a true web dev badass, you gotta nail down the fundamentals of JavaScript. Peep this dope guide on JavaScript Fundamentals - it breaks down all the crucial stuff you need to know right from the get-go.
First off, you gotta wrap your head around the language's syntax and structure.
That's like the backbone of your JS coding game. Get cozy with variables (using var and let), operators, data types, and control structures. Tutorials from freeCodeCamp and MDN Docs will hook you up with the low-down on data types like undefined, null, boolean, string, symbol, number, and object - these are the building blocks for storing and manipulating data.
Control structures like if-else statements, for and while loops are like the traffic cops of your code, telling it where to go based on different conditions.
A lot of newbies struggle with this, so pay close attention. Functions, including arrow functions and function expressions, are like the MVPs of code reusability and organization, helping you keep your codebase clean and tidy.
You gotta get your head around the Document Object Model (DOM) too.
This bad boy lets you interact with and manipulate web pages in real-time, making your web apps come alive.
Here's a quick rundown of the JS concepts you should have on lock:
- Syntax and Structure, including using "use strict"; to avoid common coding mistakes.
- Variables (var, let, const) and Data Types; the building blocks for data storage and manipulation.
- Operators (assignment, comparison, logical); the tools for performing operations on data.
- Control Structures (e.g., if/else, switch, loops); the traffic cops of your code.
- Functions (declarations, expressions, arrow functions); the MVPs of code reusability and organization.
- Events and Event Handlers; the keys to interactive web apps.
- Document Object Model (DOM) Manipulation; for dynamic web page changes.
"Master these pillars of JavaScript," says a web dev guru, "and you'll lay a solid foundation for complex web development tasks." Real talk - nail down the basics, and you'll be unstoppable in the world of interactive web development.
Implementing JavaScript for Interactive Web Development
(Up)Web dev is the shit these days, and JavaScript is the boss. Like, 95.2% of websites use it to make web pages come alive. If you're a beginner trying to get into the web interaction game with JavaScript, there are tons of practical examples and techniques to get your hands dirty.
From simple on-page effects to complex, dynamic apps, JavaScript is versatile AF. One popular technique is DOM Manipulation, where JavaScript dynamically changes the content and structure of a webpage based on what the user does.
Around 63% of JavaScript devs regularly manipulate the DOM to make things more interactive. Experts call this the manipulation of the Document Object Model (DOM), allowing for real-time content updates and interactive web pages.
Check out these common web interaction elements:
- Dropdown menus that extend when you click on them, thanks to JavaScript's ability to toggle display properties.
- Modal windows that pop up and make you make a choice or enter info.
- Validation forms that use JavaScript event handling to make sure the data you entered is legit before submitting.
Handling events is another crucial technique where JavaScript listens for user actions like clicks, hovers, or keystrokes.
On average, there are four event listeners per JavaScript-enhanced page. This allows users to control their browsing experience, which improves engagement.
Websites like Humboldt California and The St. Louis Browns use creative JavaScript implementations to create immersive and interactive user experiences.
Crafting interactive web elements like sliders, animated graphs, and dynamic content feeds is made possible with these techniques.
"JavaScript remains the backbone of web interactivity", understanding how to implement these cyphers into web design is vital for a developer's toolkit.
Using tools like D3.js can further enhance data-driven visualizations. At the end of the day, learning JavaScript isn't just about writing code; it's about unlocking the web's potential, one interactive element at a time.
JavaScript Frameworks and Libraries
(Up)JavaScript is still the boss of making websites lit in 2023. The holy trinity? React, Vue.js, and Angular.
React is the OG, with its virtual DOM and component-based flow, used by over 40% of devs. Vue.js is the chill, lightweight option that's flexible AF, loved by 15% of coders.
Angular, rocking that 30% adoption rate, is the enterprise MVP with its two-way data binding and slick material design components.
These tools make web dev a breeze with:
- Turbo-boosted Efficiency: Reusable code and pre-built functions = dev life on easy mode.
- Mind-blowing Interactivity: Reactive components and instant updates? User experiences just leveled up.
- Easy Maintenance: Modular code = updates and fixes without breaking a sweat.
They differ in their perks and learning curves:
Framework/Library | Interactivity Support | Learning Curve |
---|---|---|
React | High | Moderate |
Vue.js | High | Low to Moderate |
Angular | High | Considerable |
A tech lead from a web solutions company said, "Frameworks like React and Vue.js turn complex tasks into manageable challenges, boosting our productivity like crazy."
Bottom line? Mastering JavaScript frameworks and libraries ain't just following trends; it's a strategic move to match your project needs, ensuring efficiency, maintainability, and mind-blowing user interaction.
Common JavaScript Pitfalls and How to Avoid Them
(Up)Getting the hang of JavaScript can be a wild ride, but there are some common pitfalls that can trip you up if you're not careful. Turns out, silly mistakes like missing semicolons or brackets are responsible for a whopping 46% of JS errors that newbies face.
To avoid these snafus, it's a good idea to keep your code looking fresh with consistent formatting and use tools that can catch these syntax slip-ups before they become a headache.
But syntax isn't the only thing that can throw you for a loop.
TypeErrors and misunderstanding the 'this' keyword can also be major speed bumps.
Learning how to debug like a pro is key. Using browser debugging tools and checking out resources like Frontend Masters' course on debugging can help you track down and squash those pesky bugs.
And let's not forget about dealing with JavaScript's asynchronous nature – that can lead to race conditions and unexpected behavior if you're not prepared. But don't sweat it, using try-catch-finally blocks can help prevent total meltdowns from unhandled errors.
To really level up your async game, you'll want to get cozy with concepts like callbacks, promises, and async/await.
Hands-on practice, like the interactive sessions in Nucamp's Front-end Web Development bootcamp, can be a game-changer for reducing async-related errors.
And don't be a stranger to online communities like Stack Overflow – checking out code snippets and asking questions can help you spot patterns and identify bugs like a pro.
And when you do run into an error message, take the time to really understand what it's trying to tell you – that's often the key to solving the problem.
So, if you stay on top of error prevention, keep your coding style consistent, and tap into the wealth of resources and community knowledge out there, you'll be well on your way to becoming a JavaScript wizard.
Building a solid foundation now will pay off big time when you're slaying it in the interactive web dev game later on.
Conclusion
(Up)In the ever-changing world of web dev, JavaScript is like that one friend who's always got your back. With over 97.7% of websites using it to make pages come alive, it's the real MVP. And if you're trying to get into coding, JavaScript is the way to go.
Stack Overflow has been calling it the top dog for a solid nine years now.
But JavaScript isn't just a one-trick pony. Once you've got the basics down, you can take your skills to the next level with Node.js on the server-side, or flex your muscles with heavy-hitters like React, Angular, and Vue.js.
These frameworks are like the superpowers of front-end development, and knowing JavaScript gives you a head start in mastering them. Here's why learning JavaScript is a solid move:
- Rapid Prototyping: With JavaScript's flexibility, you can turn your ideas into real prototypes in no time, which is clutch for leveling up your skills.
- Community and Resources: The dev community has your back with tons of resources to help you through the rough patches.
- Career Opportunities: Once you're a JavaScript pro, you can explore different career paths, from front-end to full-stack development and beyond.
JavaScript is the foundation of web development – it's like the glue that holds HTML (the skeleton) and interactivity (the dynamic flesh) together.
Mastering it isn't just a nice-to-have; it's a must-have for anyone trying to make it big in the tech world these days. So, if you're looking to level up your coding game, JavaScript should be your first stop.
Frequently Asked Questions
(Up)What percentage of websites use JavaScript?
JavaScript powers 97.7% of websites, enabling rich interfaces, real-time updates, SPAs, and user engagement.
How does JavaScript boost user interaction on websites?
Sites with JavaScript-derived interactivity can see up to a 70% increase in user engagement, enhancing the overall user experience.
What are some common JavaScript pitfalls for beginners?
Common JavaScript pitfalls for beginners include syntax errors, incorrect assumptions about 'this' keyword scope, and challenges with JavaScript's asynchronous nature.
Which JavaScript frameworks and libraries are popular for interactive web development?
Popular JavaScript frameworks and libraries like React, Vue.js, and Angular offer increased efficiency, enhanced interactivity, and better maintainability for web development projects.
What are the key concepts beginners should focus on when learning JavaScript?
Beginners should focus on mastering JavaScript syntax and structure, variables, data types, control structures, functions, events, and DOM manipulation for a strong foundation in interactive web development.
You may be interested in the following topics as well:
Explore the comprehensive browser developer tools that can help you polish and perfect your websites.
Dive into beginner-friendly design techniques that will help you start your first responsive website with ease.
Unlock the secrets of precise styling by using selectors to target and style specific page elements.
Discover the relevance of HTML in the modern web era and its evolution from a simple markup language to a foundation of web development.
Refine your web design skills by understanding CSS properties and values, which dictate the look and feel of a website.
Discover why mastering the importance of web development can be a game-changer for tech industry newcomers.
Chevas Balloun
Director of Marketing & Brand
Chevas has spent over 15 years inventing brands, designing interfaces, and driving engagement for companies like Microsoft. He is a practiced writer, a productivity app inventor, board game designer, and has a builder-mentality drives entrepreneurship.