Guide to Iterating through Python Data Structures
By Jenn Rogala
Last Updated: June 4th 2024
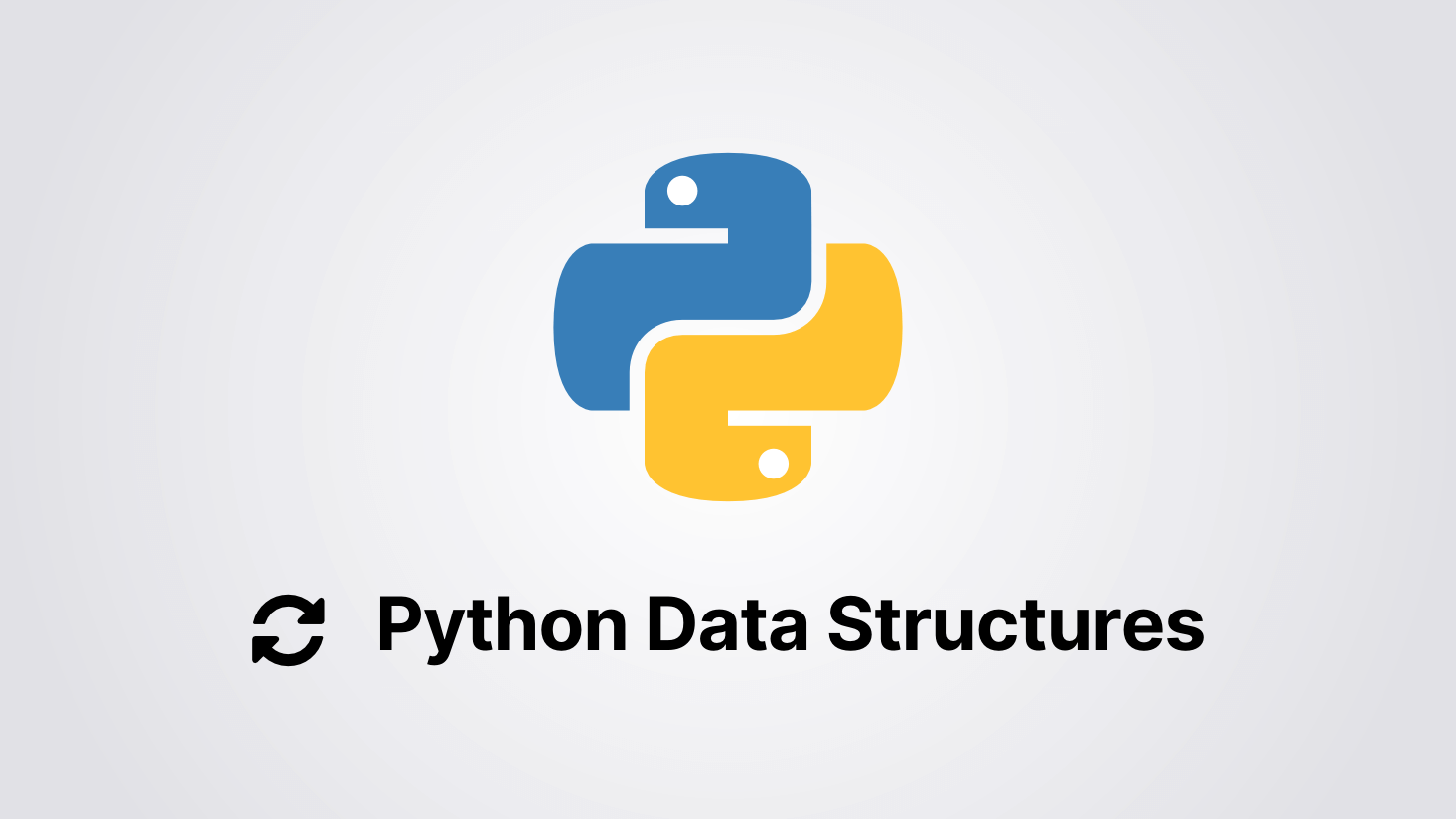
Iterating through Python Dictionaries, Tuples, Lists, Sets, and Strings.
Python is an open source, object-oriented programming language developed in 1991 by Guido van Rossum.
It's widely used for machine learning, server-side development, data visualization and much more.
Python's uncomplicated syntax resembles how we speak which makes it easy to understand.
For beginners who want to learn to code, Python programming is a great choice.
Python has 4 collection data structures: Dictionaries, Tuples, Lists, Sets.
We're also going to include strings in this guide since, in Python, each character in a string is indexed.
Each data structure can be used as an iterative value to step through, such as items in a list, characters in a string, keys in a dictionary etc.
We'll use Python For Loops to demonstrate the slight differences between each data structure.
The syntax isn't hard. It's remembering the differences in syntax between the data structures that can be challenging.
So, we'll walk through them.
But first let's quickly review the Python For Loop.
What is a For Loop?
A For Loop is a control statement used in programming languages to repeatedly execute a block of code until a specified condition is reached.
The condition can be something like - 'end the For Loop after executing the code 5 times', or - 'end the For Loop when a specified Boolean variable equals FALSE'.
In the following example, we use the Python built-in function 'range' and the Python keyword 'in'.
The range function takes 3 parameters:
- the starting value
- the ending value
- the increment value
And note the colon “:” at the end of the first statement of the For Loop.
for i in range(0, 10, 1): print(i)
This will print the numbers from 0 to 9. It will start with the variable i=0 and continue to increment the variable i by 1. It will continue to print the value of i until i=10, then the For Loop will end. It will not print the number 10.
There are several ways to control the flow of a For Loop, but we're going to narrow our focus to just the syntax of looping though the Python data structures.
Summary of Python data structures:
Data Structure | Description | Data Enclosed In... | Example |
---|---|---|---|
Dictionaries | collection of key-value pairs | {} curly braces | dict={“nm”:”Ed”, “age”:30, “mi”:”M”} |
Tuples | sequence of multiple values | () parentheses | tuple=(“two”, 1000, 27.5, {1,2,3}) |
Lists | sequence of multiple values | [] square brackets | List=[“two”, 1000, 27.5, {a,b}] |
Sets | collection of unique values | {} curly braces | set={“alpha”, 123, (true, false)} |
Strings | sequence of characters | “” or '' quotation marks | string=”abcdefg” or 'abcdefg' |
References for Methods available for each Python data structure
- Python methods for dictionaries
- Python methods for tuplies
- Python methods for lists
- Python methods for sets
- Python methods for strings
How to Iterate through Python Dictionaries
When using For Loops with Python dictionaries, you can iterate over the dictionary's keys, values, or both.
- The Dictionary college_mascots is enclosed in curly braces.
- The key is the college name. (e.g.; BC, Tulane)
- The value is the college mascot. (e.g.; Eagle, Pelican)
college_mascots = {“BC”: “Eagle”, “Tulane”: “Pelican”, “Louisville”: “Cardinal”, “Iowa”: ”Hawk”}
Use the 'keys' method to print the dictionary keys. (e.g.: college names)
for college in college_mascots.keys(): print(college)
Use the 'values' method to print the dictionary values. (e.g.: mascots)
for mascot in college_mascots.values(): print(mascot)
Option 1
Use the 'items' method to print the dictionary keys and values. (e.g.: Eagle is the mascot of BC)
for college, mascot in college_mascots.items(): print(mascot, “is the mascot of“, college)
Option 2 (produces same results as option 1)
- Uses the dictionary key to get the value.
- Also prints keys and values (e.g.: Eagle is the mascot of BC)
for college in college_mascots: print(college_mascots[college], “is the mascot of“, college
To reference a value in a dictionary by key, use bracket notation: college_mascots[“BC”].
Dictionaries are mutable. Note the use of square brackets when adding, deleting, or changing.
You can add: college_mascots[“Marist”]=”Fox”
You can delete: del college_mascots[“Iowa”]
You can change: college_mascots[“Tulane”]=”Canary”
How to iterate through Python Tuples
When using For Loops with Python Tuples, you can iterate over the tuple's items.
Here's the Tuple my_tips - enclosed in parentheses
my_tips = (7, 5, 8, 6)
This code iterates over the items in the tuple and prints the sum of the tips. (e.g.: $26)
total=0 for x in my_tips: total += x print(f'${total}')
Use the built-in 'enumerate' function to convert the tuple to an object.
Prints the position along with the item of my_tips tuple. (e.g.: (0,7) the number 7 is in position 0)
for x in enumerate(my_tips, 0): print(x)
To reference an item in a tuple by index, use bracket notation: my_tips[0]
Tuples are immutable so you cannot change the value. You cannot execute my_tips[0]=9
How to iterate through Python Lists
When using For Loops with Python Lists, you can iterate over the list's items. Lists are similar to tuples. But lists us square brackets, and tuples use parentheses. And lists are mutable, where tuples are immutable. Also, both data structures allow duplicate items.
Here's the List my_groceries - enclosed in square brackets
my_groceries = [“milk”, “bread”, “eggs”]
This code iterates over the items in the list and prints items in the my_groceries list.
for food in my_groceries: print(food)
Use the built-in 'enumerate' function to convert the list to an object.
Prints the position along with the item of the my_groceries list (e.g.: (0, 'milk') milk is in position 0)
for food in enumerate(my_groceries, 0): print(food)
To reference an item in a list by index, use bracket notation: my_groceries[0]
You can add to the end of a list: my_groceries.append(“butter”)
You can delete from a list: my_groceries.pop(0) will remove “milk”
How to iterate through Python Sets
When using For Loops with Python Sets, you can iterate over the set's items.
But keep in mind that the loop will return different results each time since sets are unordered.
Here's the Set my_todo - enclosed in curly braces
my_todo = {'wash dishes', 'laundry', 'take out trash'}
This code iterates over the items in the set and prints items in my_todo set.
NOTE: Will not always print items in same order.
for task in my_todo: print(task)
Note that you can use the enumerate function to print the position of the items in a set, but since the order is not fixed, the positions may not be useful.
Sets are mutable so they can be changed using methods such as my_todo.add(“sweep”) and my_todo.discard(“laundry”).
How to iterate through Python Strings
When using For Loops with Python Strings, you can iterate over the string's characters.
Strings are similar to tuples and lists regarding For Loop syntax. Strings are immutable and allow duplicate characters.
Here's the String my_message - enclosed in quotation marks
my_message = 'Hello World'
This code iterates over the characters in the string and prints each character in the string my_message.
for char in my_message: print(char)
Use the built-in 'enumerate' function to convert the string to an object.
Prints the position along with the characters in the string (e.g.: (0, 'H') H is in position 0)
for char in enumerate(message, 0): print(char)
To specify a character in a string by index, use bracket notation: message[0]
Strings are immutable, you cannot use the pop or append methods.
Key Takeaways:
As stated earlier, Python syntax is clear and simple, and a great place to start learning to code.
But there are times when one character or symbol is wrong or missing in your code.
And you may spend a fair amount of time trying to figure out why your code isn't working.
Maybe you used a square bracket when you should have used a curly bracket.
Or maybe you're missing a comma, parenthesis, or a quotation mark.
And if you forget the syntax for anything, the answer is usually one search query away.
Or you can always reference a copy of the above table, or our Python cheat sheet.
The Python community is large, active, and supportive.
So don't hesitate to loop someone in for help.
Happy coding!
Interested in learning Python programming?
Jenn Rogala
Senior Writer
Jennifer Rogala has worked in the area of healthcare technology for 30 years. Most of her publications were on the topic of how medical technology can improve patient safety. It wasn't until she became a mother that she started writing stories for children. From their infancy her twin daughters loved books. Seeing the joy books gave to her children inspired Jennifer to attempt to create this joy herself and share it with others. Jennifer lives outside of Boston with her twin daughters, and calico cat.