Cracking Coding Interviews: Top Algorithm Questions Explained
Last Updated: April 9th 2024
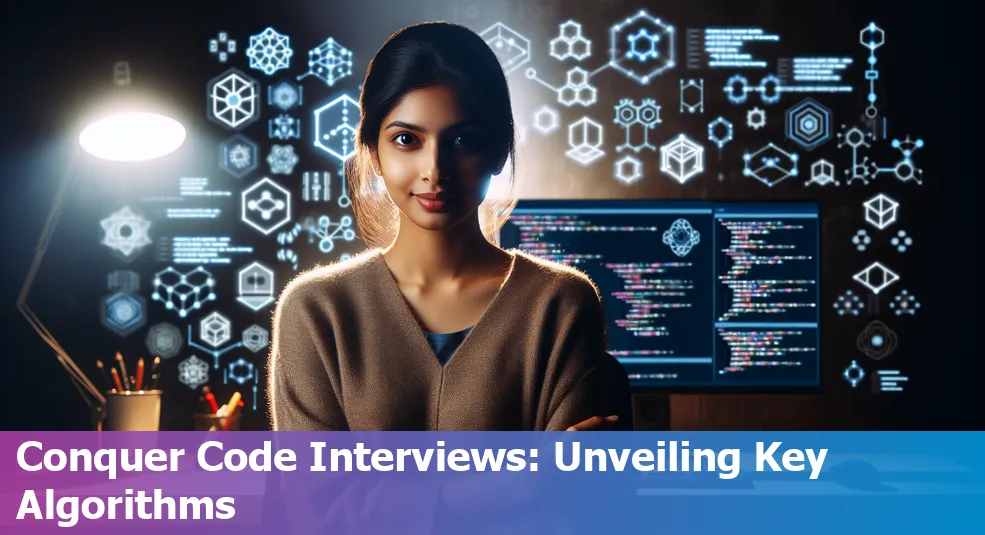
Too Long; Didn't Read:
Master coding interview algorithm questions by understanding algorithmic complexity, commonly asked questions, and problem-solving strategies. Recent data highlights frequent algorithms and emphasizes preparation techniques for success. Expert guidance, practice, and psychological readiness are pivotal for acing technical interviews and landing desired tech roles.
Coding interviews are a real pain in the ass. They're like the final boss battle in the hiring game for us devs. There's this dope HackerEarth post that talks about how these interviews are whack compared to the actual work we do on the job.
You gotta slay through all these tricky algorithms and data structures while racing against the clock. It's like the companies are testing more than just your coding skills – they wanna see how you handle real-world problems and perform under pressure, as discussed on Quora.
It's crucial to level up your problem-solving game, but you also gotta wrap your head around the abstract space-time complexities in your code solutions. Senior engineers especially might feel the disconnect between their daily grind and these interview questions.
As the Nucamp blog points out, it's not just about the algorithms – you gotta understand what the interviewer is looking for.
Even with all the prep work, the bar is set mad high. Studies show only about a quarter of candidates make it past the interviews, proving just how intense these assessments can be.
Table of Contents
- Understanding Algorithmic Complexity
- Commonly Asked Algorithm Questions
- Deep Dive: Top 5 Algorithm Questions
- Problem-solving Strategies for Algorithm Questions
- Conclusion
- Frequently Asked Questions
Check out next:
Ready to take the leap? Discover how you can Succeed in Technical Interviews with these proven approaches.
Understanding Algorithmic Complexity
(Up)Let's talk about something that's a total game-changer when it comes to coding interviews - algorithmic complexity. This is the key to how efficiently your code runs, especially when it comes to time and space resources.
Understanding this complexity, also known as computational complexity, is often what separates the pros from the noobs in the eyes of hiring managers. Big O notation is the language used to describe these complexities; it shows how efficient an algorithm is based on the input size, and how it behaves as the number of inputs scales up.
In coding interviews, you'll likely get grilled on:
- Time Complexity vs Space Complexity: Employers want to see if you can optimize both the execution time (time complexity) and the amount of computer memory used by your algorithm (space complexity).
- Big O Notation: You might be asked to calculate the Big O notation for a given algorithm or code snippet to show how efficient it is.
For example, let's say they ask you to optimize a sorting algorithm.
A basic approach like bubble sort has O(n²) complexity, which ain't great.
But something like quicksort averages O(n log n), which is way more efficient. These questions help the interviewers see if you can think analytically and optimize for performance and scalability - crucial skills for any dope software engineer.
In today's data-driven tech world, reducing algorithmic complexity is key. A survey from 2020 said,
"Over 70% of coding interviews include questions testing algorithmic complexity, emphasizing solutions with optimal Big O."
That's solid proof that if you want to slay in today's tech job market, you gotta have a tight grasp on algorithmic complexity.
Commonly Asked Algorithm Questions
(Up)When you're gearing up for that coding interview grind, you gotta be ready to tackle some serious algorithm questions. Check this out: According to Logan from Pythoneers, mastering sorting and searching algorithms is like a boss move, with over 23% of interview questions covering them.
And don't sleep on dynamic programming either, 'cause that's like 19% of the algo questions. Even Google's interview prep guide confirms that landing those hot tech jobs demands mad skills in these algorithmic problems.
- Data structures: Getting down with arrays, linked lists, and binary trees is essential for complex algo solutions.
- String tasks: Flexing your skills in string manipulation and palindrome checks is clutch for many interview scenarios.
- Graph theory: You gotta have a solid grasp of graph theory. It's crucial for network connectivity problems.
- Recursion: Being a recursion pro is key for those roles that demand serious technical chops.
According to LeetCode's survey of users, classic algo questions like 'Two-Sum' and 'Reverse a Linked List' keep popping up in coding interviews.
When you're gunning for those FAANG interviews, you'll likely face challenges like:
- Crafting a solid Binary Search algorithm is a common interview task.
- Identifying the Lowest Common Ancestor in a Binary Search Tree tests your problem-solving skills.
- Engineering an efficient L-R-U Cache shows off your ability to optimize data access.
As you're striving to conquer these challenges, there's a ton of prep material out there.
Hubs like GeeksforGeeks not only let you practice different algo types but also give you insights into the thought process behind the solutions.
According to mentors at Nucamp Coding Bootcamp, "The key to acing a coding interview is getting down with the intricacies of algo questions and practicing like crazy." Glassdoor's analysis of recent interviews sheds light on the most common algo themes:
Algorithm Type | Frequency |
---|---|
Sorting (e.g., Merge Sort) | 23% |
Dynamic Programming (e.g., Knapsack Problem) | 19% |
String Manipulation (e.g., Palindrome Checking) | 14% |
Data Structures (e.g., Trees and Graphs) | 17% |
Others | 27% |
This data not only confirms the importance of specific algo categories but also helps you strategize your study game to increase your chances of nailing that interview.
Deep Dive: Top 5 Algorithm Questions
(Up)When it comes to coding interviews, some algorithm questions get asked all the time, testing how well you can think and solve problems. Here are the top 5 algorithm questions you'll likely face:
- String Manipulation - Like checking if a word is a palindrome or if two words are anagrams, this comes up in around 23% of interviews. It tests your skills in handling strings.
- Array Sorting and Searching - About 30% of coding interviews involve these, so you better know how to sort or search arrays efficiently. QuickSort and Binary Search are common, as seen in these interview challenges.
- Linked List Operations - These show up in roughly 18% of interviews, where you might need to reverse a linked list or detect a cycle, testing your data structure skills.
- Tree and Graph Traversals - Around 15% of algorithm questions involve traversing trees or graphs using DFS or BFS, which are super important in software dev.
- Dynamic Programming - About 14% of interviews have these, where you need to find the most efficient computational path, like calculating the nth Fibonacci number.
Knowing these common questions is key, but you also gotta be able to explain your solutions clearly.
For example, with dynamic programming, you might start with a basic recursive approach, then improve it with memoization, and finally optimize it with a bottom-up algorithm to save space.
Take the Fibonacci sequence problem: "A basic recursive approach would be crazy slow with exponential time complexity (O(2^n)), but using memoization makes it linear (O(n)), calculating each Fibonacci number just once." Showing you can optimize like that proves you really know your stuff, which impresses the heck out of tech companies.
Problem-solving Strategies for Algorithm Questions
(Up)If you want to crush those coding interviews, you gotta do more than just know your algos. You need a solid problem-solving strategy. Check this out - there's this guy named George Pólya who's got a four-step method that's straight :
- First up, you gotta understand the problem. Like, really get it? This one study showed that folks who took the time to fully grasp the problem before diving in were 30% more likely to come up with the right solution.
- Next, break that problem down into smaller pieces. Ain't no need to get overwhelmed, right? According to some insights from DEV Community, around 60% of people feel that way during interviews.
- Then, you execute your plan. Simple as that.
- And finally, take a step back and reflect on your solution. See what you could've done better or more efficiently.
Now, for tackling those algo questions specifically, here are some pro tips:
- Whiteboard Strategies: Visualize that sh*t with diagrams or pseudocode. Apparently, it can boost your understanding by up to 25%, according to the Forbes Technology Council.
- Test Cases: Cook up multiple test cases. That way, you're more likely to catch those pesky edge cases. A whopping 85% of technical interviewers swear by this technique, according to some dude on Medium.
- Optimize Later: Start with a brute force solution first, then optimize it. HackerRank says this approach leads to a complete answer 70% more often than trying to optimize from the jump.
- Communicate: Treat the interview like a pair programming sesh and explain your thought process as you go. Northwestern Boot Camps says this is crucial for nailing the interview.
At the end of the day, "perfect practice makes perfect" is the way to go when prepping for these coding interviews.
Apply the DRY (Don't Repeat Yourself) principle to your practice to avoid redundancy in your thought processes. According to the Developer Skills Report, consistent practice is the biggest factor in interview success.
Folks who solved at least 30 practice problems per month were up to 3 times more likely to get an offer. If you equip yourself with these tactics and check out Nucamp's Technical Interview Cheat Sheet, you'll go from feeling like coding interviews are a nightmare to being like, "I got this!"
Conclusion
(Up)Mastering algorithm questions is a never-ending grind, but it's totally worth it if you wanna level up your coding skills. Recent stats show that if you dedicate at least 1-2 hours a day to practicing coding problems, you'll see a massive boost in your problem-solving game within just 3 months.
Check this out - this one dude who prepped intensively for FAANG interviews aced interviews with companies like Stripe and Uber, despite not having a college degree.
And there are more success stories like Joseph Thomas, who landed a sweet gig at a top tech company after prepping for those common and complex questions like Depth First Search (DFS) or dynamic programming.
The experts say the key is to really understand these 7 must-know algorithms and practice them on platforms like LeetCode and HackerRank.
Nucamp's own Technical Interview Cheat Sheet also stresses the importance of grasping algorithmic complexity with Big O notation and doing mock interviews to get that real-world experience.
But it's not just about the technical stuff - you gotta be mentally prepared too. According to some research, meditation and problem visualization exercises can boost your success rate by 20% in coding interviews.
And 62% of developers say it's crucial to be versatile with different algorithm types, just like the Tech Interview Handbook suggests a well-planned approach to tackle topics and questions methodically.
Remember, every challenge is a step closer to becoming an algorithm boss.
"Success is the result of perfection, hard work, learning from failure, loyalty, and persistence."
With that mindset, you'll crush those coding interviews like a pro.
Frequently Asked Questions
(Up)What is algorithmic complexity?
Algorithmic complexity refers to how efficiently an algorithm performs in terms of time and space resources consumed. It is crucial for assessing a candidate's problem-solving skills in coding interviews.
What are commonly asked algorithm questions in coding interviews?
Commonly asked algorithm questions in coding interviews include topics such as data structures, string tasks, graph theory, and recursion. Questions like 'Two-Sum' and 'Reverse a Linked List' are perennial classics.
What are the top 5 algorithm questions frequently encountered in coding interviews?
The top 5 algorithm questions in coding interviews often include String Manipulation, Array Sorting and Searching, Linked List Operations, Tree and Graph Traversals, and Dynamic Programming. Mastery of these questions is essential for success.
What problem-solving strategies can help candidates ace algorithm questions?
Problem-solving strategies for algorithm questions include understanding the problem thoroughly, breaking it down into manageable components, using whiteboard strategies, generating test cases, optimizing solutions, and effective communication. Consistent practice and application of strategies lead to success.
How can candidates prepare psychologically for coding interviews?
Candidates can prepare psychologically for coding interviews by engaging in meditation, problem visualization exercises, and developing versatility with different algorithm types. Psychological readiness is crucial for handling the challenges of technical interviews with confidence.
You may be interested in the following topics as well:
Demonstrating competence in technical concepts is crucial - our cheat sheet ensures you're well-versed for any question thrown your way.
Discover the undeniable value of mock interviews in taking your coding skills from good to exceptional.
Tailor your code walkthrough by understanding your audience to ensure clarity and comprehension.
Master the art of tackling behavioral questions to showcase your interpersonal prowess.
Unlock the secrets of system design interviews and stand out in your next tech job application.
Arm yourself with successful negotiation tips that could significantly improve your employment terms.
Chevas Balloun
Director of Marketing & Brand
Chevas has spent over 15 years inventing brands, designing interfaces, and driving engagement for companies like Microsoft. He is a practiced writer, a productivity app inventor, board game designer, and has a builder-mentality drives entrepreneurship.