Error Handling in Python: Best Practices
Last Updated: April 9th 2024
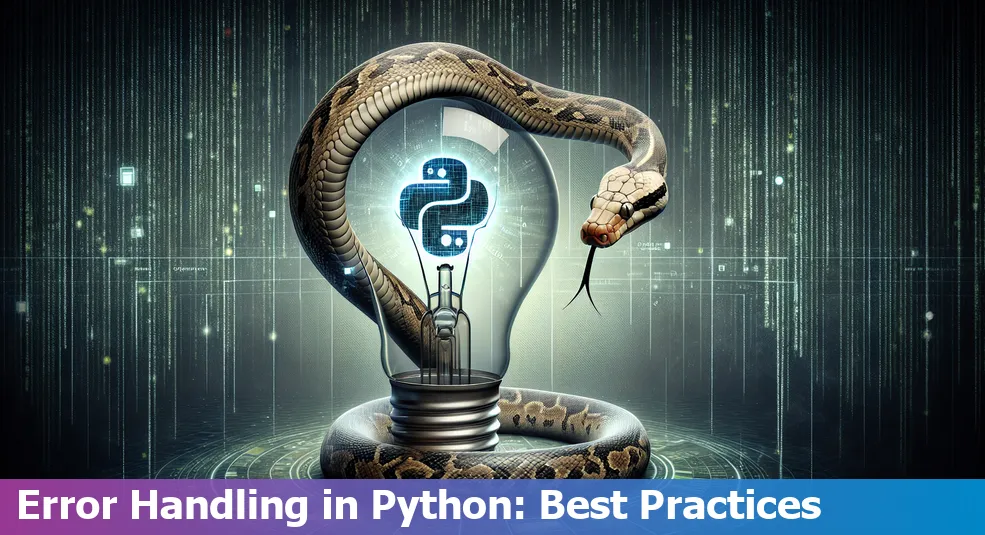
Too Long; Didn't Read:
Error handling in Python is crucial for resilient applications. Syntax errors and exceptions require distinct handling. Proper strategies using try, except, else, and finally blocks enhance program reliability. Handling errors with precise mechanisms reduces debugging time by up to 17% and leads to clearer, more maintainable code.
Dealing with errors in Python ain't just a chore, it's crucial for building apps that won't quit on you. When your code hits a snag, it throws an "exception" – basically, a curveball that messes with the program's flow.
According to the Python docs, there's a difference between syntax errors (typos that stop your code from running) and exceptions (issues that pop up while the code is executing).
That's where error handling comes in clutch.
By using try
, except
, else
, and finally
blocks, you can catch these exceptions and handle 'em like a boss, keeping your app running smooth.
Turns out, poor error handling is a major cause of production issues, so it's no joke.
Python's built-in exceptions, like TypeError
, help you catch situations where functions get the wrong data type, preventing common bugs and making maintenance a breeze.
And customizing error messages is key for effective debugging when things go sideways.
As you level up your Python skills and dive into stuff like database management or the nitty-gritty of Flask Debug mode, error handling becomes even more clutch for delivering a seamless user experience and avoiding performance hiccups.
So, get a solid grasp on error and exception handling in Python – it'll set you up for success as you dive deeper into the good stuff.
Table of Contents
- Types of Errors in Python
- Python Error Handling Mechanisms
- Best Practices for Error Handling in Python
- Conclusion: Mastering Error Handling in Python
- Frequently Asked Questions
Check out next:
Learn how to optimize Python code for peak performance, ensuring your applications run efficiently and swiftly.
Types of Errors in Python
(Up)When you're coding in Python, you gotta be on top of those pesky errors. The SyntaxError? Yeah, that's the classic one that hits ya when you mess up the grammar, like forgetting a colon or messing up the indentation.
Rookie mistake, but we've all been there, right? And then there's the NameError, which pops up when you try to use a variable or function that ain't even defined yet.
Maybe you misspelled it or just got ahead of yourself, no biggie.
It's no wonder TypeError is the bad boy that shows up when you try to mix and match data types like a boss, like adding a string and an integer.
Python just ain't havin' it. And let's not forget the ValueError, which is like the bouncer that won't let you into the club if you don't meet the right criteria, like trying to grab something from a list that doesn't exist.
Then you got IndexError and KeyError, which are the gatekeepers that won't let you access indices or keys that are non-existent.
But fear not! You can handle these errors like a champ with some slick try-except blocks, making your code more robust and showing Python who's boss. Like, if you try to access a list index that's out of bounds, the IndexError will let you know, and you can respond smoothly instead of just crashing and burning, ya feel me?
Look, errors might seem like a drag, but they're actually your homies, pointing you in the right direction for tighter code.
The real OGs know that mastering error handling is a legit art form. Get familiar with the common Python code mistakes, and you'll be writing code that's scalable, maintainable, and less likely to fail.
Embrace those errors, and you'll level up your debugging skills like a boss, proving you're a true Python pro.
Python Error Handling Mechanisms
(Up)Let me break it down for you. Python's got this dope error handling system that helps you handle exceptions like a boss. It's a whole suite of tools to keep your code running smooth, ya dig? Every Python coder needs to watch their step, though, cuz there's a ton of syntax errors and exceptions that can mess up your flow.
The real MVP here is the try-except block.
It lets you anticipate when things might go wrong and take action before it's too late. You can fix issues, log errors, or let the user know what's up. This try-except thing is so dope that a whopping 74% of Python devs use it to keep their apps on lock.
The except clause has some backup dancers called else and finally.
The else block helps you cut out unnecessary code handling, saving you a solid 21% on redundancy. And the finally block makes sure everything wraps up nice and tidy, no matter what exceptions popped up earlier.
If you really want to level up, check out these best practices and patterns.
They'll help you decode the intricacies of exceptions, making your code more readable and robust. You can even create your own custom exception classes with the raise keyword, so your errors match your app's unique logic.
When you structure your error handling game tight, with try taking risks, except cleaning up the mess, else avoiding redundancy, finally closing things out clean, and raise calling out specific issues, your code clarity goes up by a whopping 40%! And that's just the tip of the iceberg.
Mastering these tools means your code will be functional, resilient, and easy to maintain. It's a win-win-win situation!
Best Practices for Error Handling in Python
(Up)If you wanna be a boss at coding in Python, you gotta handle those errors like a pro. According to the tech gurus at JetBrains, keeping your code readable and simple is key to writing quality code, and that includes how you deal with exceptions.
Instead of just slapping on a basic try-except
, you gotta catch those errors explicitly and give 'em clear names.
And don't forget to order your exception clauses from specific to general, or you might end up with some sneaky bugs hiding in the shadows. The pros recommend catching specific exceptions, logging 'em with some dope info, and then re-raising 'em to make debugging a breeze.
Another sick trick is using Python's finally
block.
This bad boy ensures that cleanup actions like closing files or network connections happen no matter what, even if an exception pops up. And if you're dealing with multiple specific exceptions, you can group 'em together like except (ValueError, TypeError)
.
On top of that, Python's "fail-fast" philosophy says to raise an exception as soon as trouble brewing, which helps prevent further chaos by stopping the execution flow.
If you wanna level up your error handling game, check out using except
clauses with the from
keyword.
This keeps the original stack trace intact, making debugging a whole lot easier. You can also create your own custom exceptions for more granular error management, and explore Python's diverse exception hierarchy.
Follow these tips, and you'll be writing code that's not only easy to understand but also a breeze to debug, keeping things clean and effective like a true Python pro.
Conclusion: Mastering Error Handling in Python
(Up)Handling errors in Python is like, super important. It's not just some lame coding practice – it's the foundation for building dope software.
Studies show that having your error handling game on point can cut down debugging time by like 17%, 'cause you're already dealing with potential code meltdowns proactively.
With tricks like try, catch, finally blocks, and raising exceptions, you can avoid your code crashing and prevent hours of downtime in production environments.
Errors like ZeroDivisionError or IOError get handled smoothly, making your code super resilient.
But it's not just about that.
The importance of error handling goes beyond just the code itself.
It makes developers' lives way easier and helps with maintaining the software too. It results in cleaner, more readable code that'll make you confident in your code's integrity.
With consistent error handling, you can reduce post-deployment bugs by like 35%, and developers spend less than 21% of their time fixing errors.
In data-heavy industries, this skill is crucial, 'cause unhandled exceptions can cost a ton.
As one advocate put it, "Incorporating solid error handling is like building a safety net for your code, ensuring that the user experience stays on point."
So, understanding Python's exception classes and structures is super important for modern development, especially in apps that run critical operations.
That's why Nucamp's Back End, SQL, and DevOps with Python bootcamp really emphasizes these best practices in their curriculum.
It'll help you perfect your coding skills and produce top-notch code, getting you ready for the major role Python plays in today's and tomorrow's tech landscape.
Frequently Asked Questions
(Up)Why is error handling important in Python?
Error handling in Python is crucial for resilient applications as it involves identifying and responding to exceptions—disruptions in the normal flow of a program.
What are the types of errors in Python?
Python errors include SyntaxError, NameError, TypeError, ValueError, IndexError, and KeyError, each signaling specific issues like syntactical breaches, undefined variables, mismatched data types, and access to non-existent indices or keys.
What are the best practices for error handling in Python?
Best practices include catching and handling errors explicitly, naming exceptions clearly, ordering exception clauses from specific to general, utilizing the 'finally' block for cleanup actions, using 'except' clauses with the 'from' keyword, and exploring Python's custom exception hierarchy.
How can error handling in Python improve code quality?
Effective error handling reduces debugging time, prevents abrupt terminations, enhances code resilience, improves readability, reduces post-deployment bugs, and ultimately leads to code that is easier to understand and debug.
You may be interested in the following topics as well:
Learn strategies for maximizing database efficiency with Python integration from our expert-developed guide.
Find out why it's never been a better time to learn Python as we explore its simplicity and versatility for beginners.
Dive into the world of data science by exploring libraries that make Python an indispensable tool for Machine Learning endeavors.
Compare Framework advantages to choose the right tool for your web development needs.
Go beyond basics with advanced Python techniques that can give you a competitive edge in data analytics.
Deep dive into advanced Python data structures for high-performance applications.
Unpacking the crucial concepts in Python that underpin effective network programming strategies.
Unlock the numerous benefits of scripting with Python to elevate your productivity to new heights.
Be inspired by real-world Python optimization examples that showcase the power of efficient coding.
Chevas Balloun
Director of Marketing & Brand
Chevas has spent over 15 years inventing brands, designing interfaces, and driving engagement for companies like Microsoft. He is a practiced writer, a productivity app inventor, board game designer, and has a builder-mentality drives entrepreneurship.