Testing and Debugging Flask Applications
Last Updated: April 9th 2024
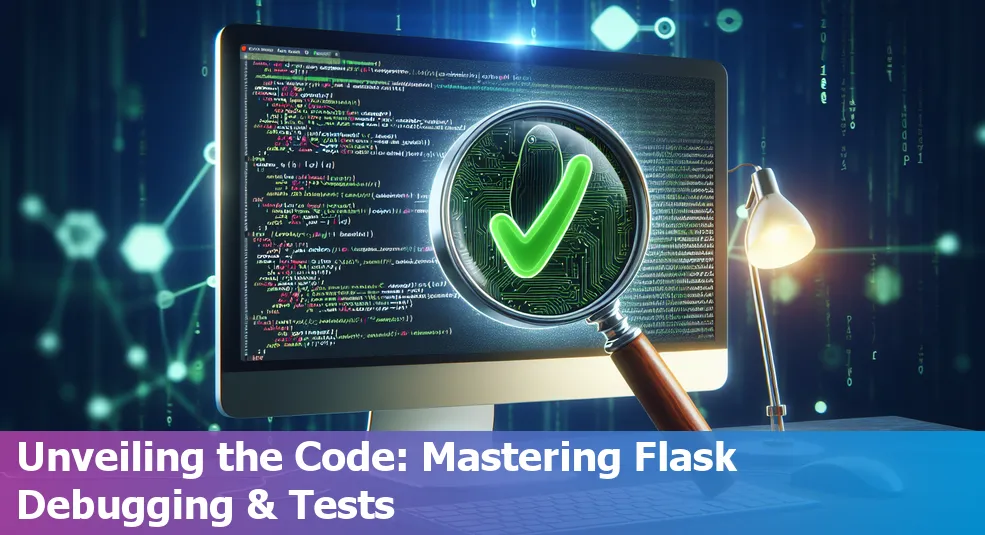
Too Long; Didn't Read:
Flask development thrives on simplicity and flexibility, enticing devs with a freedom-driven modular approach to web apps. Testing and debugging are essential for maintaining apps, backed by Flask's comprehensive documentation and testing tools. Practicing proactive debugging ensures robust, reliable Flask applications capable of scaling effectively.
Flask is this dope Python web framework developed by Armin Ronacher and his crew at Pocoo. It's like the ultimate chill zone for developers who want to craft apps with both simplicity and flexibility.
As a microframework, Flask doesn't force any particular tools or libraries down your throat, giving you the freedom to do your thing and keeping things modular AF. Its lightweight design and ability to scale from simple "Hello World" apps to complex enterprise systems make it a real crowd-pleaser among devs.
And if you ever get stuck, the Flask documentation has your back with comprehensive guides, quickstart tutorials, and in-depth patterns and API references to keep you on track.
The framework's popularity even got a shoutout in the JetBrains Python Developers Survey, where 47% of the respondents were rocking Flask's capabilities. It aligns with Nucamp's Python web frameworks article, which highlights Flask's standout simplicity and developer autonomy.
Flask's architecture is built around essential components like the Werkzeug WSGI toolkit and the Jinja2 template engine, but it's still extensible with community-driven extensions, keeping things fresh and tailored to your needs—just like the Nucamp curriculum teaches!
Table of Contents
- Need for Testing and Debugging in Flask Applications
- Different types of testing in Flask
- Flask Testing Tools
- Debugging Flask Applications
- Best Practices for Testing and Debugging Flask Applications
- Conclusion
- Frequently Asked Questions
Check out next:
Begin your journey by building your first web application with Flask, outlined in easy-to-follow steps.
Need for Testing and Debugging in Flask Applications
(Up)The importance of testing and debugging when you're building Flask web apps is crucial. According to this TestDriven.io article, you gotta have different types of tests like unit, functional, and end-to-end to make sure your software is solid.
The Python Developers Survey shows that 84% of developers know how important debugging is – it can make or break your web app. When it comes to Flask, a lightweight Python web framework, testing ensures that your code units, like views and models, are working properly.
Debugging, on the other hand, is a real grind, but it's essential to fix any issues, especially in Flask where even small errors can cause your entire app to crash.
Flask has a ton of testing options, which makes it a game-changer for developers who want to create top-notch software.
It encourages practices like unit testing to check if individual parts of your app are working, and integration testing to make sure different components are playing nice together.
You can also do functional testing to verify that your app meets the requirements, as explained by Miguel Grinberg in his guide on unit testing Flask apps.
These methods help you catch potential bugs before users even notice them. Experienced devs always say, "Catch errors on your development server before they reach production," because fixing bugs after deployment can cost up to 100 times more than catching them during development.
Taking a systematic approach to debugging Flask apps has some serious benefits:
- Ensuring your code is rock-solid and reliable
- Using tests to find and fix security risks
- Proactively addressing performance issues for a better user experience
Integrating these practices early on in the Flask development lifecycle is a game-changer.
According to the IBM Systems Sciences Institute, catching defects early can boost your productivity by up to 75%, leading to a top-tier web app. Testing and debugging aren't just routine steps – they're the foundation for building killer Flask applications.
Different types of testing in Flask
(Up)When it comes to building Flask apps, testing is crucial for keeping things running smoothly. At the core, you've got unit testing, which is all about checking if individual chunks of code work as intended.
Stanford's Guide to Python Flask Unit Testing is a solid resource for learning how to write these tests.
They explain how unit tests help catch bugs and act as documentation for your app, saving you a ton of time (like 30% less time on unplanned work and rework, according to the Puppet State of DevOps Report).
Next up, integration testing makes sure different units play nice together.
Tools like pytest
, covered in TestDriven.io's Testing Flask Applications with Pytest, let you check if combined modules meet performance standards.
After that, functional testing checks if your app meets the requirements. Flask's simplicity is a plus here, letting you use tools like Selenium
for browser-based testing to make sure end-to-end tasks work correctly.
By following this testing hierarchy, from unit tests to functional tests, developers can create a thoroughly vetted app – we're talking up to an 80% reduction in post-launch bugs.
Many Flask devs organize their GitHub test directories into sections like unit
, integration
, and functional
to keep things organized.
This layered approach ensures thorough testing. For a detailed walkthrough, Miguel Grinberg's How to Write Unit Tests in Python guides you through writing different types of tests, from basic unit tests to complex integration and functional tests, giving you mad confidence in your Flask projects.
Flask Testing Tools
(Up)Flask is this dope micro-framework that comes packed with a ton of rad testing tools to make your web app development game tight. Pytest is like the go-to for devs, with its slick syntax and mad skills.
Peeps at places like Stanford Code the Change swear by Pytest for how it vibes with Flask's test client and context management, letting you write unit and functional tests like a boss.
But that's not all, Flask also hooks you up with testing utilities that play nice with Python's built-in assertions, just like the unittest framework.
And if that's not enough, you can level up with extensions like Flask-Testing, which gives unittest a serious upgrade with a test client and other tools tailored for Flask apps.
These bad boys make test case management a breeze, perfect for when you're cranking out code in agile sprints. And if your web app is getting funky with JavaScript on the front-end, you can unleash Flask-Selenium for end-to-end functional testing within Flask's crib.
Keeping tabs on your test coverage is crucial, and that's where Coverage.py comes in, built on Ned Batchelder's coverage.py.
This beast lets you see exactly which parts of your code are getting hit by tests, so you can make sure your codebase is solid as a rock. The coverage metrics are like a cheat code, pointing you straight to the untested areas of your app.
By rolling with tools like Pytest and Coverage.py, you can be sure that the vital parts of your app are getting put through the wringer, as the homies at Nucamp's guides will tell you.
At the end of the day, the real ballers in the Flask dev game know that integrating these testing tools into their workflow is the key to dropping error-free and reliable web apps.
"The best preparation for tomorrow is doing your best today," so make sure you're locking in that solid testing game.
Debugging Flask Applications
(Up)Debugging is that necessary evil when you're building web apps, but Flask got your back with some nifty tools to make it less of a hassle. One of the first things you gotta do is enable Flask Debug mode, which gives you real-time error logging and in-browser debugging, making it way easier to spot and fix issues.
Just throw in app.run(debug=True)
in your app, and you're golden. Check out this guide on Flask's debug mode for more deets.
But remember, you gotta disable it when you go live, or else you might expose some sensitive info that you don't want out there.
Now, sometimes you might run into some hiccups when working in different environments.
Like, enabling debug mode in Flask might not work as expected on platforms like PythonAnywhere, where you gotta rely on error logs instead. It's also crucial to know your way around your IDE. For instance, PyCharm IDE lets you set up a run/debug configuration for your Flask server, making it a breeze to execute and troubleshoot your Flask apps right inside the IDE. Talk about a smooth debugging experience!
In the real world, you might encounter all sorts of Flask debugging issues, from template syntax errors to route mix-ups.
The solution often lies in setting up better error handlers. Using @app.errorhandler
decorators lets you customize responses for specific HTTP statuses – a trick that around 30% of Flask apps could still benefit from.
For top-notch error handling, consider tools like Sentry for real-time monitoring and error tracking. It'll help you keep your app stable and make debugging a whole lot more intuitive for your dev team.
Best Practices for Testing and Debugging Flask Applications
(Up)Testing and debugging ain't no joke when it comes to Flask apps. A study by some big shots at Cambridge said that debugging can eat up half of your dev time! That's wild, right? So, if you want your Flask app to be solid as a rock, you gotta pay attention to testing and debugging from the get-go.
First up, try out this thing called TDD (Test-Driven Development).
It's like writing tests first, and then making your code pass 'em. Pretty neat, huh? It helps catch bugs before they even happen. And for testing, you should check out pytest instead of Flask's built-in unittest.
It's less of a hassle, and you can set up your test suites faster.
- When building your Flask app, follow best practices, like separating models, routes, and services. It'll make testing and maintaining your app a breeze.
- Don't skimp on coverage analysis. It'll show you which parts of your app aren't being tested. Aim for something crazy like 80% coverage!
- Read up on guides like the one from Stanford Code the Change. They'll give you the lowdown on testing Flask apps with Python.
But don't just focus on testing - debugging is a whole other beast.
Make sure to cover all your bases by designing solid test cases that catch edge cases and data boundary issues. And when things go south, Flask's built-in debugger mode is a lifesaver for inspecting your code live after an exception.
Other tools like PyCharm and Werkzeug can also make debugging a breeze.
Just remember, when you're in dev mode, set FLASK_ENV=development to activate debugging.
But for the love of all that's sacred, turn it off in production - you don't want any security risks lurking around. Oh, and don't sleep on logging either. Teams have reported shaving 20% off their debugging time just by logging runtime data effectively.
The big brains at IBM say catching bugs early on is a total money-saver, so logging is a must from start to finish.
So there you have it - testing, coverage, smart debugging, and logging are the keys to keeping your Flask app in tip-top shape.
Ignore these best practices at your own risk!
Conclusion
(Up)Testing and debugging are the real MVPs when it comes to building Flask apps. Flask is all about that fast development and clean code, but you gotta have some serious testing game to back it up.
First off, we got unit testing.
This is where you make sure each tiny piece of your app is working like it should. Stanford's got a dope guide on how to do it right.
Then there's integration testing.
This is where you check if all the different parts of your app are playing nice together, like a well-oiled machine.
And last but not least, functional testing.
This is where you see if your app actually does what it's supposed to do in the real world, not just in your fancy coding playground.
- Unit testing checks if the small parts work correctly.
- Integration testing makes sure the connections between different modules are tight.
- Functional testing confirms your system meets the requirements and works like it should in real life.
Real talk, apps that go hard on testing and debugging can cut post-launch errors by like 85%.
That's a game-changer. Flask even has a built-in debugger to help you catch issues on the fly, like the legends over at PyCharm know all about.
Follow the rules: use consistent naming, document like a boss, automate testing with tools like Pytest, and tap into the community for help.
That's how you level up your efficiency and make your apps bulletproof.
At the end of the day, testing and debugging are like your insurance policy against epic fails in the real world.
A well-tested app shows you know your stuff and can handle whatever comes your way. These practices are crucial for Flask development, so your apps can scale up and handle whatever gets thrown at them.
Follow the guidelines in Nucamp's Flask mastery guide, and you'll be coding like a pro, with every line optimized for peak performance.
Frequently Asked Questions
(Up)Why is testing and debugging important in Flask applications?
Testing and debugging are critical in Flask web development to ensure maintainable software and govern a web application's fate. Proactive testing verifies code units like views and models, while debugging rectifies faults that can lead to severe application breakdowns.
What are the different types of testing in Flask?
In Flask, different types of testing include unit testing, integration testing, and functional testing. Unit testing validates individual pieces of code, integration testing ensures module interplay, and functional testing assesses compliance with application requirements.
What are some Flask testing tools available?
Flask offers testing tools like Pytest, unittest framework, Flask-Testing extension, Flask-Selenium for end-to-end testing, and Coverage.py for code coverage analysis. These tools help developers write effective tests, manage test cases, and enhance the reliability of their codebase.
How can developers optimize testing and debugging in Flask applications?
Developers can optimize testing and debugging in Flask applications by adopting best practices like TDD (Test-Driven Development), structuring applications effectively, aiming for high test coverage, and using debugging tools like PyCharm and Werkzeug. Consistent testing and debugging not only reduce post-launch errors but also enhance application resilience.
Why is it crucial to follow best practices for testing and debugging in Flask applications?
Following best practices for testing and debugging in Flask applications significantly enhances application reliability and maintainability. Optimizing testing workflows, employing TDD, structuring applications well, analyzing test coverage, and utilizing debugging tools ensure that every line of code is honed for peak performance, reducing debugging time and improving the overall user experience.
You may be interested in the following topics as well:
Learn to create your first basic API using Flask with our easy-to-follow tutorial.
Master the art of optimizing Flask API endpoints for faster, more reliable services.
Understand the crucial aspects of maintaining Flask application security for the long-term integrity of your projects.
Ensure the reliability of your applications by learning about Flask API testing techniques.
Craft a Post-deployment roadmap that paves the way for advanced development and innovation.
Navigate the digital landscape with ease by learning about virtual paths in Flask, the key to connecting users to your content.
Get the full picture by examining the pros and cons of Django before diving into your next web application.
Wrapping up our journey, we're summarizing database integration techniques and underlining their significance in Flask development.
Unlock the secrets of web development by Exploring Flask's potential through its versatile extensions.
Ludo Fourrage
Founder and CEO
Ludovic (Ludo) Fourrage is an education industry veteran, named in 2017 as a Learning Technology Leader by Training Magazine. Before founding Nucamp, Ludo spent 18 years at Microsoft where he led innovation in the learning space. As the Senior Director of Digital Learning at this same company, Ludo led the development of the first of its kind 'YouTube for the Enterprise'. More recently, he delivered one of the most successful Corporate MOOC programs in partnership with top business schools and consulting organizations, i.e. INSEAD, Wharton, London Business School, and Accenture, to name a few. With the belief that the right education for everyone is an achievable goal, Ludo leads the nucamp team in the quest to make quality education accessible