Optimizing Python Code for Efficiency and Speed
Last Updated: June 5th 2024
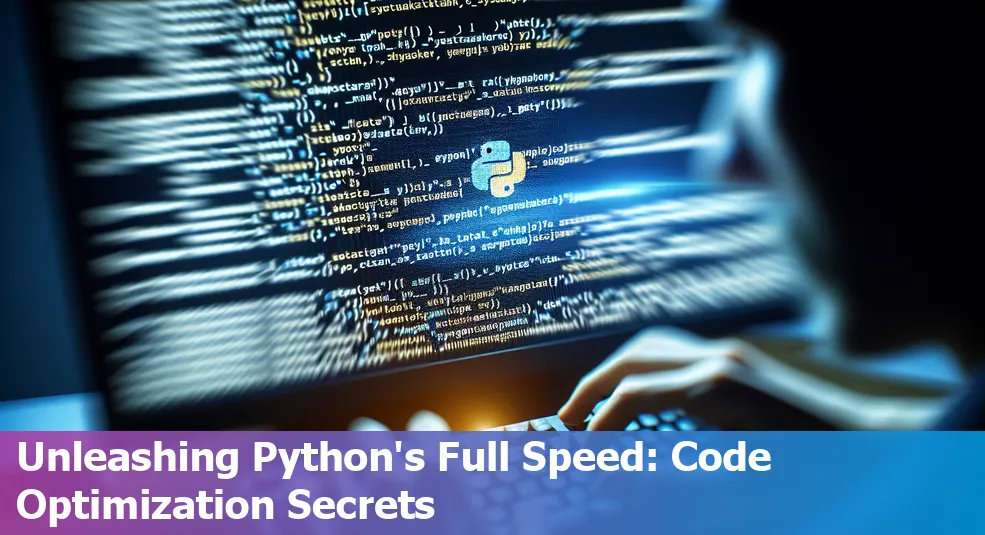
Too Long; Didn't Read:
Python's readability and versatility shine in web dev, data analysis, and AI. While criticized for speed, optimization techniques like just-in-time compilation and C extensions can rival compiled languages. Efficient Python coding leads to faster apps, reduced memory usage, scalability, and user satisfaction. Strategic optimization is key.
Let me break it down for you about Python - this language is fantastic! It's all about that clean code and massive library, making it a top choice in the programming world.
Whether you're building websites, crunching data, or diving into AI, Python's got your back.
But here's the real deal - Python shines when you need to move fast and keep that code fresh.
Its dynamic nature and powerful data structures make reusing and modularizing code a breeze. That said, Python can be a bit slower compared to languages like C, but don't underestimate it! With techniques like just-in-time compilation and C extensions, you can get Python running efficiently.
That's where Nucamp's guides on CI/CD best practices come in handy.
They'll show you how to unleash Python's full potential and optimize that performance. Whether you're automating tasks or diving deep into data analysis, Python's got the capability to get the job done if you approach it correctly.
Table of Contents
- The Importance of Optimizing Python Code
- Guidelines for Writing Efficient Python Code
- Optimizing Python IO Operations and Looping Constructs
- Python's Built-in Tools for Speed Optimization
- Using External Tools to Boost Python Performance
- Real-life Examples of Python Code Optimization
- Conclusion: Balancing Python Efficiency and Readability
- Frequently Asked Questions
Check out next:
Discover how Python in web development powers the internet, and choose the right framework between Flask and Django for your projects.
The Importance of Optimizing Python Code
(Up)Optimizing your Python code is crucial for making your apps run faster, especially since Python can be a bit sluggish due to its interpreted nature.
Getting your code optimized can lead to massive speed boosts, which is essential for time-sensitive tasks.
Studies show that with some smart optimization techniques, Python code can run up to 50% faster. For data analysis, where speed directly affects how quickly you can make decisions, optimization is a game-changer.
And when it comes to web apps, snappy response times are key to keeping users engaged, as our resources on speeding up Django apps highlight.
- Blazing fast execution: Focusing on efficiency means your apps will run way quicker.
- Less memory hogging: Optimization typically leads to leaner memory usage.
- Better scalability for your apps: Efficient code can handle more users as your app grows.
- Happier users with snappy response times: Fast feedback makes for a better user experience.
When it comes to Python runtime, proper optimization strategies can drastically cut the time it takes to run complex operations.
For instance, improving your algorithm's efficiency can turn a minutes-long process into a matter of seconds. Techniques like Peephole Optimization or runtime analysis can make a huge difference, turning sluggish apps into agile beasts.
To speed up your Python code, some top tips include:
- Use built-in data structures: like sets and dictionaries for faster lookups, and minimize global variable usage.
- Implement list comprehensions: and generator expressions for concise and efficient looping, as recommended in high-performance tips.
- Leverage parallelism: when possible, to take advantage of multiple CPU cores for concurrent execution, bypassing Python's global interpreter lock (GIL) limitations.
At the end of the day, optimizing your Python code's performance can make or break a project's success.
As one senior dev at a major tech firm said,
"Efficient Python code isn't just about getting stuff done faster; it's about scaling your app efficiently and delivering a smooth user experience."
So, make sure to set aside time for refactoring and optimization during development to keep your Python app running like a well-oiled machine.
Guidelines for Writing Efficient Python Code
(Up)Writing Python code that's sleek and speedy is crucial if you wanna be a coding wizard. One key move is sticking to PEP 8 style guidelines, which make your code easier to read and higher quality.
First, you gotta profile that bad boy – tools like cProfile can sniff out those pesky bottlenecks, and visualizing the results gives you a heads up on where to optimize.
Python's got some built-in functions and libraries that are already turbo-charged, so use those whenever you can.
For instance, 'map()' can blaze through loops way faster. Same goes for list and dictionary comprehensions – not only do they look slick, but they'll also outrun traditional iterations.
Oh, and minimizing global variable access can give your code a serious speed boost, since local variables are the real MVPs.
Picking the right algorithms is a game-changer too.
Using the appropriate data structures and swapping out inefficient algorithms like bubble sort for quicksort can make your code fly. Knowing the time and space complexity of different approaches is clutch.
And let's not forget about writing clean, well-documented code following best practices – that'll make your code way more usable and maintainable in the long run.
But here's the real deal: keep it simple and readable.
As Python's creator Guido van Rossum said, "Code is read much more often than it is written." So, while you're optimizing for speed, don't forget to write self-documenting code with variable names that actually make sense and thorough function descriptions that follow PEP 8 guidelines.
That way, your Python scripts won't just be efficient but also easy to maintain and scale up over time.
Optimizing Python IO Operations and Looping Constructs
(Up)If you want your Python code to run like a champ, you gotta optimize those I/O operations and loops. That's the key to speed and efficiency.
First up, let's talk about handling data files.
It's crucial to do things right, like using buffered I/O methods with open()
and specifying a buffer size to reduce system calls. Or, you can leverage the power of io.BytesIO
for in-memory byte operations.
And if you're dealing with massive datasets, check out Delta Lake optimizations like compaction and data skipping to make reading and writing a breeze.
Now, when it comes to file handling, here are some tips:
- Close those files ASAP to free up resources and avoid wasting them.
- Use context managers, like the
with
statement, to automatically close files even if an error occurs. - Employ lazy loading with generators or
itertools
to process large files efficiently, as recommended in the Delta Lake docs for optimizing loops using maps.
Let's talk about looping like a pro:
- Use built-in functions instead of explicit loops, 'cause they're often implemented in C and way faster.
- Eliminate duplicate computations within loops and embrace list comprehension for speedier executions.
Now, to dodge those pesky I/O and looping bottlenecks, follow these steps:
- Minimize file operations inside loops and consider techniques like Z-ordering to optimize data access patterns.
- Use
.join()
on strings instead of concatenation loops to reduce time complexity, leveraging the immutable nature of strings in Python. - Adopt built-in functions and tools like PyPy for module-level optimizations, which can deliver more efficient execution paths than traditional loops.
As Susan Martin says, optimal performance comes from a higher level of abstraction in data management, not nitty-gritty I/O mechanics.
Follow this philosophy and unleash the full potential of Python's optimization libraries, including loop and I/O operations, to write high-performing Python applications that'll blow your mind!
Python's Built-in Tools for Speed Optimization
(Up)Python's got some dope tools to make your code blazing fast. One of the sick ones is cProfile, which is like a built-in spy that tells you how often your functions are called and how long they take to run.
Just hit up python -m cProfile script.py
and bam, you got all the juicy details on what's slowing your program down. With cProfile, devs have seen their code speed up by like 25% after optimizing the sluggish parts.
And if that's not enough, there's this KDnuggets article talking about other profilers like Yappi for when you're dealing with multi-threaded code.
Another dope tool is timeit, which lets you time different code snippets to see which ones are the quickest.
This Plain English tutorial shows you how to use it on small pieces of code.
And if you're doing a ton of calculations, you can use functools.lru_cache to cache the results, which can make your functions run up to 10x faster.
To really crank up the speed, you gotta use Python's optimized built-in functions and libraries, write your loops with list comprehensions and generator expressions for better efficiency, and pick the right data structures like sets and dicts for quick access.
As one expert puts it, "Efficient Python code isn't just about using these tools, it's about knowing how to combine them in the right way for maximum impact." So if you want your code to fly, you gotta learn to wield these optimization resources like a pro.
Using External Tools to Boost Python Performance
(Up)If you're looking to crank up Python's speed, you gotta check out these sick tools and libraries. First off, Cython is a game-changer. It turns your Python code into C code, making it run way faster.
Users have seen some serious boosts, like anywhere from 30% to 50% quicker for computational tasks. It's like giving your Python a dose of pure adrenaline.
PyPy, an alternative Python interpreter, is a beast.
With its just-in-time compilation magic, it can make your code blaze up to 7.6 times faster than the regular CPython interpreter. It's like strapping a rocket to your code's back.
And for real-time insights, check out Coralogix's Python Logging Best Practices. They'll teach you how to log like a pro, giving you full transparency into your code's performance.
Now, if you wanna find those pesky bottlenecks slowing your code down, you gotta use profilers like Yappi and cProfile.
These bad boys will lay bare all the juicy details about your code's runtime, helping you pinpoint the inefficiencies and squash 'em. And let's not forget about NumPy and SciPy.
These libraries are like steroids for scientific computing, leveraging C and Fortran code to make your calculations fly. Even the folks on Reddit admit that Go can outrun Python in some scenarios, especially when calling C code.
So, you gotta pick the right tool for the job.
And last but not least, Numba is a straight-up beast. It translates your Python functions into optimized machine code at runtime, boosting your data processing speeds by up to 50%.
It's like giving your numerical algorithms a nitrous boost, making them scream through those calculations like a hot rod on the drag strip. With all these tools in your arsenal, Python's gonna be a serious contender in the performance game.
Real-life Examples of Python Code Optimization
(Up)In the tech world, optimizing Python code isn't just about making it faster, it's about keeping your systems scalable and easy to maintain, even when they get crazy complex.
Real-life examples from different industries prove this is the way to go.
Take Stackify for instance.
They stress how important it is to optimize Python, whether you're working on CPU-intensive tasks or managing memory usage. Instagram gave their backend a major boost by using Python's asyncio library, resulting in a 2x performance jump for CPU-bound workloads.
That's insane!
Dropbox built their own custom server-side compiler called Pyston, which made their code run around 30% faster. With over 500 million users, that's a game-changer.
Spotify, rocking 345 million active users, slashed their service latencies by fine-tuning their Python usage with CPython's ecosystem and C extensions.
But it's not just digital companies.
Even NASA used optimized Python code for their shuttle mission planning, resulting in faster computational executions that were critical for success.
Check out these real-life optimization wins:
- Instagram: Doubled performance with asyncio
- Dropbox: Pyston's 30% improvement in execution time
- Spotify: Reduced backend latencies by leveraging CPython and C extensions
- NASA: Accelerated mission algorithms via optimized Python code
According to industry experts, Python is the go-to choice for optimization in various sectors thanks to its versatility and rich ecosystem, including libraries like PuLP for linear programming (check out PuLP's documentation).
Leading companies often say that optimizing Python isn't just about individual gains, but part of a broader tech shift towards refining Python code for long-lasting performance and user satisfaction.
Conclusion: Balancing Python Efficiency and Readability
(Up)When it comes to Python coding, keeping things efficient and readable can be a real balancing act. While speed and efficiency are important, having code that's easy to understand and maintain is also very important.
Programmers spend a lot of time trying to figure out and tweak code, so readable Python can seriously boost productivity. Python's human-friendly syntax is its calling card, and keeping that vibe going while chasing performance gains is key.
The savvy devs out there got some tips for striking that sweet spot:
- Keep It Clear: Use descriptive variable and function names to make that purpose clear.
- Stay Consistent: Follow PEP 8, Python's official style guide, for a consistent, unified coding flow.
- Document That: Provide solid documentation to break down complex logic and keep it all straight.
- Refine and Shine: Refactor that code to eliminate redundancies and keep it DRY (Don't Repeat Yourself).
- Leverage the Goods: Utilize Python's built-in features and libraries for efficient common tasks.
But here's the real deal: when performance is a must, that efficiency-readability juggling act gets real.
Code optimized for speed might pack some complex algorithms that'll have future coders scratching their heads. On the flip side, ultra-readable code might not be as efficient.
It's all about finding that sweet spot between the two.
The Python Software Foundation and coding gurus out there agree: don't compromise either aspect, but consider the long-term benefits of code that's easy to maintain and scale.
As 'The Zen of Python' says, "Readability counts," reminding us that maintainability is a critical form of optimization in itself. So, sticking to the guidelines for crafting Python code that's user-friendly and efficient lays the groundwork for software that's built to last, scale up, and stay robust.
Frequently Asked Questions
(Up)Why is optimizing Python code important?
Optimizing Python code is essential for enhancing performance and speed, especially since Python is known for slower execution due to its interpreted nature. Efficient code leads to faster running applications, reduced memory usage, better scalability, and enhanced user satisfaction.
What are some strategies for writing efficient Python code?
Some strategies for writing efficient Python code include utilizing built-in data structures, implementing list comprehensions and generator expressions, leveraging parallelism, profiling code, minimizing global variable access, and focusing on algorithm selection and optimization.
How can Python IO operations and looping constructs be optimized for better performance?
Python IO operations and looping constructs can be optimized by using buffered I/O methods, efficient file handling practices, employing built-in functions over explicit loops, eliminating duplicate computations, and adopting tools like PyPy for module-level optimizations.
What built-in tools does Python offer for code optimization?
Python offers built-in tools like cProfile for profiling code, timeit for benchmarking, and functools.lru_cache for caching. These tools help developers analyze and optimize their code for better performance.
Which external tools can be used to boost Python performance?
External tools like Cython for transforming Python to C code, PyPy for just-in-time compilation, performance profilers like Yappi and structured logging practices can be used to boost Python performance significantly.
You may be interested in the following topics as well:
Learn strategies for maximizing database efficiency with Python integration from our expert-developed guide.
Discover how Python's popularity is growing not just among coders, but across various industries worldwide.
Start your Machine Learning journey by setting up the environment with Python and familiarizing yourself with its extensive ecosystem.
Discover how an emphasis on efficient coding through proper error handling can be a game changer for developers.
Unpack the unique Features of Flask and understand why developers praise its simplicity and flexibility.
Discover the power of Lambdas in Python to streamline your code and make data analysis more efficient.
Discover why Python's popularity in data structures has skyrocketed among developers.
Unpacking the crucial concepts in Python that underpin effective network programming strategies.
Master the technique of writing efficient scripts to fulfill a variety of automation needs.
Chevas Balloun
Director of Marketing & Brand
Chevas has spent over 15 years inventing brands, designing interfaces, and driving engagement for companies like Microsoft. He is a practiced writer, a productivity app inventor, board game designer, and has a builder-mentality drives entrepreneurship.