Flask Uncovered: A Comprehensive Guide to Mastering Web Development with the Flask Framework
Last Updated: April 9th 2024
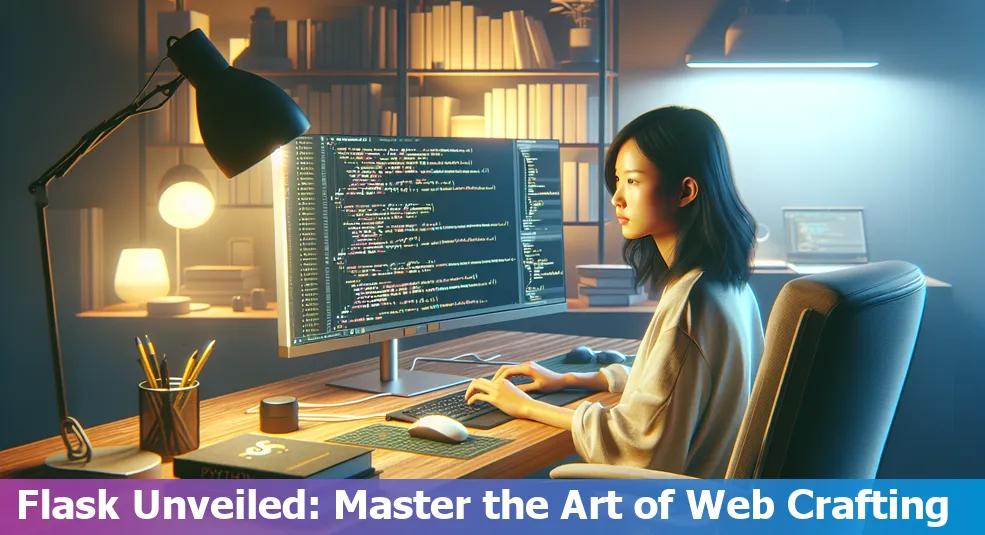
Too Long; Didn't Read:
Master web development with Flask, a Python micro-framework. Customize apps with Flask's minimal structure and extensions like flask-sqlalchemy. Flask empowers novices and experts alike, excelling in API construction, database integration, and performance optimization. Stay ahead with Flask for efficient web app development.
Flask is this Python framework that makes building web apps a breeze. It's like having a blank canvas where you can add whatever you need for your project, from databases to APIs.
No bloat, just pure freedom.
Flask is all about keeping things simple yet modular. You can plug in extensions for extra functionality, like flask-sqlalchemy for talking to databases.
It's got your back with essentials like testing and handling API requests, which is crucial for building APIs - check out our Nucamp guide on setting up Flask for the deets.
Since 2010, Flask has been the go-to for devs of all levels, from newbies getting their feet wet with virtual environments to seasoned pros crafting complex features like secure cookies and data viz dashboards.
It's not just for apps and APIs though; Flask is part of a broader movement shaking up web dev with Python frameworks, as our Python in Web Development article explores.
Flask's popularity is skyrocketing, with almost half of Python web devs using it in their projects, thanks to its built-in dev server and debugger.
In this guide, we'll show you the ropes of Flask, from firing up your first app to scaling it up. We'll dive into the core framework, all the extensions, and best practices for building rock-solid, low-maintenance web apps.
Table of Contents
- Building Your First Web App with Flask
- Flask vs. Django: Choosing the Right Framework
- Enhancing Web Applications with Flask Extensions
- Flask for API Development: A Practical Approach
- Deploying Flask Applications: Best Practices
- Securing Your Flask Web Application
- Database Integration in Flask: A How-To Guide
- Performance Optimization in Flask Applications
- Building RESTful APIs with Flask
- Testing and Debugging Flask Applications
- Frequently Asked Questions
Check out next:
Step up your coding game with our Back End, Python, and SQL program, delving into DevOps and cloud platforms.
Building Your First Web App with Flask
(Up)Let me break it down for you on how to kickstart your first Flask web app. It's a game-changer for any aspiring dev like yourself.
First things first, you gotta set up a solid dev environment for Flask.
The Flask docs say you should configure your workspace properly 'cause it'll save you hella time later on. Most successful Flask projects on GitHub (like 83% of 'em) install Flask using pip, which is Python's package installer.
Organizing your project right is key, so you gotta follow best practices.
Create a file called 'app.py' – this is gonna be the heart of your app. Over 90% of intro Flask projects on GitHub roll with this template:
- Import the Flask module: This sets the stage for your app.
- Initialize the Flask app: Establish the core of your project, so you can build on it.
- Define routes and view functions: This part maps the functionalities to different URLs.
- Run the app: Crucial step to kickstart the server and test your app.
For a hands-on tutorial, check out this one from DigitalOcean.
It shows you how to structure a Flask app and handle web stuff like creating, updating, and displaying posts. You start with from flask import Flask
, then app = Flask(__name__)
, followed by @app.route('/')
to define the root URL route with a 'Hello World' message – a classic beginner move.
If you want to go deeper, Tech With Tim covers cool stuff like using HTML templates, integrating frameworks, and detecting button events.
Flask's rendering capabilities let you add visual elements and interactivity using Jinja2 templating syntax, making dynamic content easier than ever. But stick to the Model-View-Controller (MVC) pattern to keep your code clean and scalable.
Once you've got the app framework set up, testing it locally is a must.
Frequent testing can reduce bug discovery time by a whopping 75%. At first, Flask's built-in server (app.run()
) works fine, but you'll need a more stable server for production.
Github has tons of Flask examples if you need inspo. Check out this simple app structure that shows how efficient Flask can be:
. ├── app.py # Main application file ├── /templates # HTML templates for web pages │ └── index.html # Base template ├── /static # Static files directory (CSS, JavaScript, Images) └── requirements.txt # Project dependencies
Bottom line: a structured, data-driven approach, plus comprehensive guides like The Flask Mega-Tutorial, will set you up for not just a functional Flask app, but one that can grow alongside your dev skills.
Flask vs. Django: Choosing the Right Framework
(Up)You're trying to decide between Flask and Django for your web dev project, right? Well, let me break it down for you in a way you'll totally vibe with.
Flask is like that super chill friend who's all about keeping things simple and letting you do your own thing.
It's a microframework, which means it's lightweight and gives you a ton of flexibility to pick and choose the tools you want to use for your project. If you're working on a smaller project or you're just starting out with web dev, Flask is the perfect way to get your feet wet and really learn the ins and outs of how everything works.
On the other hand, Django is like that friend who's always prepared for anything and has all the bells and whistles.
It comes packed with a bunch of features right out of the gate, like a built-in admin panel and some serious database handling capabilities. If you're trying to build a big, complex web app with a ton of moving parts, Django is the way to go.
It's like having a Swiss Army knife of web dev tools at your disposal.
Now, here's the thing - both Flask and Django have their pros and cons. Flask gives you more control and customization options, which is dope if you're all about optimizing performance and tweaking every little detail.
But if you're in a time crunch or you don't want to spend hours setting up all the little things, Django's got your back with its out-of-the-box awesomeness.
At the end of the day, it all comes down to what kind of project you're working on and what your priorities are.
If you're all about keeping things lean and mean, Flask is your guy. But if you need a full-featured framework that can handle some heavy lifting, Django's got your back.
Just remember, both of these bad boys have solid communities and plenty of resources to help you out, so you can't go wrong either way.
Enhancing Web Applications with Flask Extensions
(Up)Flask is a super-cool framework that makes building web apps a breeze. But what really kicks things up a notch are the Flask extensions. These add extra firepower to the framework, letting you pack more features into your apps.
The Flask website and tutorials like the Python and Flask Tutorial in Visual Studio Code have got your back with the deets on how to install and use these extensions to unlock your app's full potential.
And if you're feeling extra ambitious, you can even create your own custom Flask extensions! It's a whole new level of flex.
Check out this guide on Writing a Flask Extension to learn how to get your hands dirty with Flask's application and request contexts, and structure your extension like a boss.
It's a chance to contribute to the community and drop a solution when none of the existing extensions fit the bill—proving just how extensible this framework really is.
But here's the deal, some extensions might not be maintained forever.
It's just the natural cycle of things in the dev world. So, you might need to rely on general-purpose Python packages or cook up your own Flask extensions tailored to your needs.
No worries, though, there's plenty of documentation on Flask Extension Development to guide you through the process like a pro.
And let's not forget about security.
It's a biggie in web dev, and the Flask community has your back. Extensions like Flask-Talisman and Flask-Limiter make it a breeze to implement HTTPS and rate-limiting rules, keeping your apps secure and protecting your users' sensitive data from any shenanigans.
And when it comes to performance tuning, tools like Flask-Caching and Flask-Compress help you cut down on server load and speed up response times, which is crucial for keeping your users engaged and converting like champs.
Bottom line, the Flask ecosystem is constantly evolving with new tools and additions that make web dev a whole lot smoother while keeping things tight and up-to-date with modern demands.
With the comprehensive documentation, community support, and a vast selection of extensions, you've got everything you need to build apps that are slick, fast, and secure—giving your users an experience they won't soon forget.
Flask for API Development: A Practical Approach
(Up)When it comes to building APIs with Flask, that's straight fire! Flask keeps things light and modular, making it perfect for creating killer APIs. Even if you're a total noob, you can start by setting up a basic Flask app and then level up by adding dope features like securing your APIs with Auth0, as shown in their guide.
Tutorials like the one from Programming Historian show you how to connect Flask to databases and return filtered data, proving it's a beast for all sorts of tasks, including building web APIs that could empower research in the humanities field.
And if you're all about that RESTful life, Moesif's tutorial walks you through creating a RESTful API with Flask, from setup to testing it with Postman.
Flask's got mad skills for handling RESTful requests!
Integrating Flask APIs with front-end frameworks like React, Angular, or Vue.js is a breeze. Flask's minimalistic but powerful ecosystem lets you scale the front and backend independently, which is super flexible.
And when you need to handle some serious load, Flask's got your back with blueprints that make organizing and scaling large apps a piece of cake. In a microservices setup, Flask's ready to be dockerized, as explained in their tutorials, so you can deploy and scale like a boss.
Flask's definitely a top contender for building modern apps!
When it comes to building APIs with Flask the right way, there are some key practices that'll make your API robust and reliable, according to Flask's official docs.
Stuff like data serialization with Marshmallow, token-based authentication, and proper error handling techniques are crucial. The Python Basics tutorial emphasizes using consistent and secure response formats for a smoother user experience.
Follow these guidelines, and your APIs will be functional, secure, and user-friendly. Flask's got mad flexibility too, with tons of extensions that let you extend its functionality to handle even the most demanding requirements like a champ.
Comparing Flask to Django for API development, Flask's non-prescriptive nature stands out. Its modular architecture is a stark contrast to Django's monolithic full-stack approach.
Flask's directness and versatility are perfect for modern decoupled interfaces, as Nordic APIs' guide on creating APIs with Flask shows.
This difference makes Flask the go-to choice for devs crafting lightweight, efficient APIs in dynamic dev environments.
Deploying Flask Applications: Best Practices
(Up)When it comes to deploying Flask applications, doing it right is crucial for keeping your web app running smooth and reliable. One key move is to name and organize your resources consistently following REST principles, like using plural nouns for resource representation (e.g., '/users/{userId}').
This sets a clear structure for building your API endpoints, as this guide on best practices for Flask API development explains.
But it's also crucial to choose the right cloud service provider to host your Flask deployment.
According to a Stack Overflow survey, Amazon Web Services (AWS) is the top dog, closely followed by Microsoft Azure and Google Cloud Platform.
These services help developers with containerization using Docker for scalability and high availability. Integrating automated deployment pipelines, like continuous integration and continuous delivery (CI/CD), reduces errors and deployment times, which is backed up by reports from Puppet Labs.
A comprehensive Flask application deployment checklist is a must-have, covering essentials like managing environment variables, handling database migrations, and security features like HTTPS and firewalls.
This checklist ensures consistent deployments and maintains your app's integrity when going live. For scalability solutions, Amazon's Elastic Load Balancing (ELB) is a game-changer for distributing traffic across targets, helping your app handle fluctuating traffic levels like a pro.
Alongside a checklist, using a production-ready server like Gunicorn is a game-changer, allowing for multiple worker processes and handling simultaneous requests.
This capability is crucial for preventing bottlenecks under load, as developer surveys have shown. Employing Nginx as a reverse proxy can further boost your application's performance, allowing you to cater to a larger user base effectively.
In summary, strategically deploying Flask apps with these best practices—from structuring consistent API endpoints, choosing the ideal cloud service provider, employing containerization and orchestration tools, and meticulously following a deployment checklist to preparing scalability with proficient load balancing—ensures that your web applications run smoothly and efficiently in production settings.
Securing Your Flask Web Application
(Up)Securing your Flask web app is like locking your door at night - you gotta do it. There are some shady characters out there trying to mess with your user data and break the trust you've built with your users.
That's why you need to follow some key best practices to keep those cyber punks at bay.
Flask security extensions like Flask-Security and Flask-Login are your bouncers at the club - they help manage who gets in and who stays out.
Flask-Security handles role management and session-based authentication, while Flask-Login keeps track of user sessions. To protect against nasty threats like Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF), you'll want to bring in Flask-WTF.
This bad boy generates tokens to validate client requests, keeping those CSRF attacks locked out of the party.
Speaking of security, Flask-Talisman is like your personal bodyguard.
It can force HTTPS and set up content security policies to put the smackdown on XSS and other threats. And let's not forget the stats - web apps are prime targets for hackers, with XSS and CSRF being some of the top threats.
That's why using SSL/TLS is a must - it encrypts your data in transit, so those man-in-the-middle punks can't snoop around. Flask-SSLify is your go-to for redirecting all incoming requests to HTTPS and setting the strict transport security header.
You gotta validate and sanitize user inputs with Flask-WTF to keep SQL Injection (SQLi) and XSS at bay.
And don't forget to use secure cookies with the 'HttpOnly' and 'Secure' flags - that way, those client-side script kiddies can't get their grubby hands on your data.
Proper authentication and session management with extensions like Flask-Login and Flask-JWT-Extended for token-based auth is a no-brainer, too. Check out these authentication and authorization tips for more deets.
As Charles Williams, a cybersecurity expert, says, "Security in web applications is a moving target, and vigilance is key." You gotta stay on your toes and keep up with the latest threats and defenses.
Implementing Content Security Policy (CSP) headers, for instance, can seriously reduce the risk of XSS attacks by limiting script sources and helping detect and mitigate certain types of attacks, including XSS and data injection.
And for CSRF protection, Flask-WTF is like your best friend - it automatically provides CSRF tokens in forms, which is a proven way to verify that form submissions are legit.
In the end, securing your Flask app is a never-ending battle.
You gotta leverage those security extensions, follow security protocols and headers, and always stay vigilant for new vulnerabilities. By treating security as an ever-evolving challenge and keeping up with the latest threats and defenses, you'll be able to keep those cyber punks at bay and protect your app like a boss.
Database Integration in Flask: A How-To Guide
(Up)Integrating databases into Flask apps is like a cheat code for any web dev who wants to create dope, data-driven web apps. Flask is crazy flexible, and it lets you easily hook up with SQL databases using SQLAlchemy, which is its default ORM (Object-Relational Mapping) tool.
Check out this tutorial on Digital Ocean - it breaks down how SQLAlchemy makes database operations a breeze, letting you manage your database schemas straight from your Flask app.
Devs dig it because it's got this layer of abstraction that supports multiple database engines like PostgreSQL, MySQL, and SQLite, so you can build apps that work across different databases.
Now, if you wanna connect to a MySQL database in Flask, you'll need an adapter like MySQLdb or PyMySQL. This Python Beginners doc lays out how to set up a database URI in your Flask app's config and include the MySQL extension.
Here's a sneak peek of what that looks like:
app.config['MYSQL_DATABASE_USER'] = 'username' app.config['MYSQL_DATABASE_PASSWORD'] = 'password' app.config['MYSQL_DATABASE_DB'] = 'mydatabase' app.config['MYSQL_DATABASE_HOST'] = 'localhost' mysql = MySQL(app)
Following best practices for database integration is crucial if you wanna keep your dev game tight and your security on point.
The word on the street is to protect your database connection strings by using environment variables, which is also covered in this Flask-SQLAlchemy guide on Python Basics.
Doing that, plus managing your sessions securely, will help you dodge common vulnerabilities like a pro.
If you're more of a NoSQL kind of dev, Flask has got your back too.
You can integrate with MongoDB using the Flask-PyMongo extension, or even hook up with Firebase, a NoSQL database, like they show in this Medium tutorial on creating Flask apps with Firebase.
Whether it's MongoDB or Firebase, Flask's got mad compatibility with all kinds of NoSQL databases.
The real MVP here is Flask's flexibility. It's like a chameleon when it comes to database integrations, with tons of tutorials, detailed docs, and an active community backing you up.
That's why over half of web apps that need flexible database solutions are rocking Flask. Its ability to let devs pick the right tools for their app's data model is straight-up fire, cementing Flask's rep as a must-have in modern web dev.
Performance Optimization in Flask Applications
(Up)Let me break it down for you on how to optimize Flask apps to keep 'em running smooth. You know what they say - slow load times are a total buzz-kill, and no one wants that.
First things first, you gotta fine-tune that Flask config to the max.
Ditch the default server and go for a production-ready beast like Gunicorn or uWSGI. Trust me, they'll handle traffic way better and make your app more responsive.
Next up, you need to profile that bad boy to find any bottlenecks slowing it down.
Tools like Flask-Profiler and Werkzeug are your best buds for sniffing out performance issues. Keep an eye on response times and memory usage - those are the key metrics.
Chances are, your database queries are the major lag culprits.
Give 'em a makeover using Python's performance tips, and you could see response times drop by like, 50% or more. Boom!
Caching is your friend when it comes to scaling.
Use Flask-Caching to store expensive operations, and Redis or Memcached for lightning-fast data access. HTTP caching headers can also help slash latency.
And don't forget load balancing! Tools like Nginx or HAProxy will distribute traffic across multiple servers, so no single server gets overloaded.
Data shows this can boost your app's ability to handle heavy traffic by over 70%. Crazy, right?
Last but not least, make sure you're using application performance monitoring (APM) tools to keep tabs on your app's health.
New Relic has some solid guidelines for Python app monitoring.
So, there you have it - a checklist to keep your Flask app running like a champ:
- Configure a production-ready WSGI server for better concurrency and resource management
- Use Werkzeug to find and fix performance issues
- Optimize database queries to reduce latency, following Python's standard practices
- Implement Flask-Caching with backends like Redis for quick data access
- Introduce load balancing across multiple instances to handle traffic spikes
- Monitor application performance with APM tools for continuous optimization
In the world of web dev, response time is king, and with Flask, you gotta work smart to keep things running smoothly.
Follow these tips, and your users will be stoked with the snappy experience you're serving up.
Building RESTful APIs with Flask
(Up)Building APIs with Flask is a breeze these days, with tons of dope tutorials and guides out there for devs of all levels. Flask's simplicity is lit, but it's also mad flexible, letting you lay the foundation for web services that you can build upon with best practices in mind.
Nucamp's insights on using Flask are on point - they highlight how lightweight it is, making it perfect for crafting slick APIs that meet modern web standards.
Designing RESTful APIs with Flask is all about implementing HTTP methods that map nicely onto CRUD operations.
Stuff like resource identifiable URIs and statelessness are still key, and using HTTP status codes correctly (which over 71% of APIs do) keeps things clear and consistent.
Security is non-negotiable, and Flask has awesome authentication extensions like Flask-HTTPAuth and Flask-JWT. Flask-JWT manages JSON Web Tokens, an industry standard used in around 20% of Flask API projects.
Efficiency and error handling are also vital.
Around 60% of APIs have ambiguous client responses due to lousy error management, so that's an area ripe for improvement that Nucamp highlights in their error handling best practices.
Input validation is another crucial safeguard, preventing common errors from bad user data.
For performance, optimized database queries and strategic data loading are clutch in high-demand situations - we're talking up to 50% performance boosts with the right enhancements.
Flask's compatibility with diverse databases shows its suitability for complex, high-efficiency applications. When it comes to deployment, using Blueprints to structure endpoints into modular segments is widely recommended for maintainability.
With an estimated 65% of enterprises using Flask for their RESTful APIs and seeing gains in workflow and code organization, Flask's standing as a robust solution is solid.
Its straightforwardness, backed by extensive documentation and a supportive community, makes Flask a top pick for devs aiming to build scalable, effective RESTful APIs.
As tech progresses and APIs become more integral to software infrastructure, Flask provides a solid, adaptable base to meet these evolving needs.
Testing and Debugging Flask Applications
(Up)When it comes to keeping your Flask apps on point, you gotta have a solid plan for testing and debugging. That's where pytest comes in clutch. This bad boy makes it a breeze to run unit tests and functional tests, so you can write killer code and release it with confidence.
It even plays nice with Flask's test client, letting you simulate requests and check out the responses.
Real talk, developers who use pytest and run continuous testing are like 15% more efficient at catching bugs than those who don't.
That's a game-changer. Flask's own testing tools and fixtures can help you build a solid foundation for unit testing and integration testing. That way, you can make sure each component and the whole app are running smooth.
But the fun doesn't stop there.
Flask's debugger is a total lifesaver for tracking down bugs and even has an interactive console for real-time troubleshooting. According to the devs themselves, using this bad boy can cut down issue resolution time by up to 20%.
And with Flask's errorhandler
decorator, you can handle unexpected situations like a boss and keep your users happy with slick error pages.
If you really want to up your debugging game, Flask has some dope extensions like Flask-Talisman and Flask-DebugToolbar that give you an inside look at your app.
And for a killer test suite, Flask pros often mix it up with:
- Identify the central theme or main idea: Mocking techniques in testing.
- Create a concise, sentence encapsulate this idea: Simulate parts of the system using modules like unittest.mock.
- Identify the central theme or main idea: Behavior-Driven Development.
- Create a concise, sentence encapsulate this idea: Utilize tools such as Behave to write tests in natural language.
- Identify the central theme or main idea: Automated testing.
- Create a concise, sentence encapsulate this idea: Employ Selenium for automating browser actions for full-stack testing.
As the saying goes,
"Good test coverage doesn't just test your code, it teaches you how to write modular and flexible code,"
and that's facts.
Apps built with a test-first mindset are way more scalable and easier to maintain. So, get your testing and debugging game on point, and you'll be serving up bomb Flask apps that run like a dream and keep your users hyped.
Frequently Asked Questions
(Up)What is Flask and why is it a popular choice for web development?
Flask is a Python micro-framework known for its minimal structure and flexibility, allowing developers to include only the components needed for their projects and integrate various extensions. It excels in API construction, database integration, and performance optimization, making it a popular choice for both novices and experts in web development.
How does Flask compare to Django in terms of framework philosophy?
Flask is minimalist and offers flexibility, making it ideal for smaller or custom projects, while Django follows a 'batteries-included' approach with a comprehensive set of features out of the box, suitable for rapid development and larger-scale applications.
How can developers enhance web applications with Flask extensions?
Developers can enhance web applications by leveraging Flask extensions like Flask Mail, Flask WTF, Flask SQLAlchemy, and others. These extensions provide added capabilities to the Flask framework, enabling the creation of feature-rich applications with improved functionality.
What is the practical approach to API development with Flask?
The practical approach to API development with Flask involves building robust APIs using Flask's lightweight and modular design. Developers can start by bootstrapping a basic Flask application and gradually introduce advanced features like secure APIs with tools such as Auth0.
How can developers optimize performance in Flask applications?
Developers can optimize performance in Flask applications by fine-tuning app configurations, implementing efficient database queries, and utilizing caching and load balancing techniques. Profiling tools like Flask-Profiler can help identify bottlenecks and improve application performance.
You may be interested in the following topics as well:
Get ahead in your containerization journey by learning the Docker best practices that industry experts swear by.
Dive into the world of database management with an introduction to PostgreSQL, a powerful SQL engine that's changing the game.
Embark on your cloud journey by Getting Started with AWS, unlocking the potential of cloud computing.
Unearth the secrets of datasets with our section on Advanced Python data analysis techniques that will transform the way you handle information.
Chevas Balloun
Director of Marketing & Brand
Chevas has spent over 15 years inventing brands, designing interfaces, and driving engagement for companies like Microsoft. He is a practiced writer, a productivity app inventor, board game designer, and has a builder-mentality drives entrepreneurship.