Flask for API Development: A Practical Approach
Last Updated: April 9th 2024
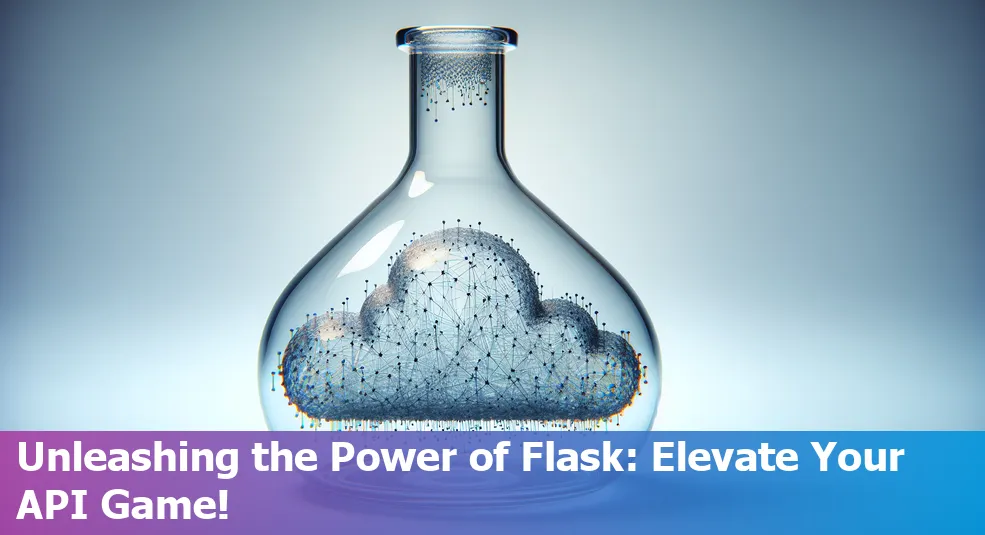
Too Long; Didn't Read:
Flask simplifies API development, emphasizing scalability and modularity. Its pluggable nature and key tools like SQL Alchemy elevate it for database interactions. Testing acumen and compatibility with Python libraries endorse its maturity. Industry trends indicate Flask as a practical ally for modern API development, balancing simplicity with flexibility and power.
Flask is this dope Python web framework that makes building APIs a breeze. It's all about keeping things simple and flexible, which is key for modern programming where scalability and modularity are da bomb.
This framework is a total game-changer for both microservices and monolithic systems. Its 'bare-bones' approach gives you just the right tools to quickly whip up web apps of any size, from small to massive.
What really sets Flask apart is its versatility.
With extensions and blueprints, you can customize it to your heart's content, unlike those rigid one-size-fits-all frameworks. Plus, it plays nice with heavy-hitters like SQL Alchemy for seamless database management and marshmallow for smooth data serialization.
The Programming Historian lesson breaks down how to make the most of these features.
Flask is also a pro when it comes to testing and works like a charm with all the popular Python libraries, which just goes to show how mature it is for building rock-solid, maintainable APIs.
The coding community is so hyped about creating extensions for it, as revealed in the JetBrains 2020 Python Developers Survey. That's why Flask is the second-most popular Python web framework out there.
Our Nucamp article gives you the lowdown on using Flask to build RESTful APIs, so be sure to check it out.
We'll be diving deeper into setting up the environment, API nitty-gritty, and how Flask can take your web services to the next level in future installments.
Table of Contents
- Setting Up Your Environment
- Building a Simple API with Flask
- Advanced Flask Concepts for APIs
- Real-World Applications of Flask APIs
- Conclusion: Why Choose Flask for API Development
- Frequently Asked Questions
Check out next:
Begin your journey by building your first web application with Flask, outlined in easy-to-follow steps.
Setting Up Your Environment
(Up)Setting up your sesh for Flask API development starts with the basics - installing Python. Almost half of all devs worldwide are rocking Python 'cause it's a straight-up breeze and has a ton of cool tools.
To get Flask running smoothly, you gotta create a virtual environment. All the pros recommend this move to keep your Python projects separate and avoid any dependency drama.
Here's the deal: Follow this guide to set up your environment and get Flask installed.
- Grab Python from the official site, making sure it plays nice with Flask (usually 3.6 or newer).
- Use Python's built-in
venv
module by typingpython3 -m venv flask_env
in your terminal to create your virtual environment calledflask_env
. - Activate the environment with
source flask_env/bin/activate
(Mac/Linux) orflask_env\Scripts\activate
(Windows). - Once it's active, install Flask by running
pip install Flask
, which is a must-have package manager as explained in these Flask setup instructions from Twilio.
Setting up your Flask dev environment the right way is clutch for a smooth workflow.
Rock Visual Studio Code as shown in this tutorial from Microsoft to take advantage of its terminal, editor, and debugger tailored for Flask.
As Flask veteran Patricia Anderson says:
"Set your FLASK_ENV to development to enable all development features including debugger and auto-reload."
Flask's lightweight and modular design makes it perfect for building scalable APIs.
For full backend power, your Flask environment should handle JSON through flask.json
and parse requests with Flask-RESTful
.
Flask's extensibility with tools like Flask-SQLAlchemy
for database integration and Flask-Migrate
makes managing database schema changes a breeze, proving it's a solid choice for full-fledged API projects.
Building a Simple API with Flask
(Up)Creating a basic API with Flask is all about setting up your dev environment.
Flask is a Python framework that makes building web apps a breeze, and it powers a ton of Python-based APIs out there. The first step is to define your routes using the @app.route()
decorator, which tells Flask what URLs to listen for and what HTTP methods (like GET or POST) to accept.
These days, structuring your Flask API endpoints and using Flask's jsonify
to turn Python dicts into JSON responses is the way to go.
If you're just starting out, here's the basic steps:
- Installation: Install Flask by running
pip install Flask
in your terminal. - Initialization: Import Flask and create a Flask app instance.
- Routing: Define your routes with functions that return data in JSON format.
- JSON Conversion: Convert your data to JSON using Flask's
jsonify
to make it web-friendly. - Server Execution: Start your Flask app on a local server with
app.run()
, as the Moesif Blog explains.
Implementing CRUD operations is a must, and Flask makes it easy.
For example, to CREATE something, you'd set up a POST route to let clients submit data. Structuring your Flask API according to best practices, like using Blueprints for scalability, parsing requests with Flask-RESTful, and separating your MVC components, is the way to go.
Flask gives developers the tools to innovate in the backend, which is what Charles Miller was all about – taking risks and pushing technology forward.
Even a basic Flask API for simple data retrieval will use some of Flask's features to get the job done for small apps.
But as your requirements grow, scaling Flask means adding more complex stuff like handling JSON parameters and database integrations, which are crucial for larger systems.
Advanced Flask Concepts for APIs
(Up)As you dive into building complex APIs with Flask, you'll realize how crucial it is to master some advanced concepts to create APIs that are solid and secure.
Middleware in Flask, like Werkzeug for handling requests and responses, is a game-changer when it comes to tackling cross-origin resource sharing (CORS) issues—a nightmare for many full-stack devs.
And let's not forget about user authentication; over 75% of organizations in Postman's API development survey agree that it's a must-have for API security. Flask extensions like Flask-HTTPAuth and Flask-JWT are your best friends, locking down those API endpoints from unauthorized access.
- Isolating test cases, making it easier to maintain and reduce dependencies,
- Using Python's unittest or pytest frameworks to build a solid testing suite,
- Integrating CI/CD pipelines that automatically run tests, a approach that has boosted development efficiency by 60% for businesses that adopted it.
Delivering clear error messages in Flask is a surefire way to elevate the user experience, and 92% of users in industry surveys vouch for its trustworthiness.
Flask's errorhandler()
and register_error_handler()
functions are your go-to tools for crafting custom error responses. Building sophisticated RESTful APIs with Flask involves all these techniques, ensuring your APIs are secure, user-friendly, and fully testable.
As one expert puts it,
"Implementing Flask's advanced features for API construction not only streamlines your workflows but also significantly enhances the quality of your web services, aligning with the high standards for web application effectiveness that are expected nowadays."
These practices reflect a commitment to top-notch web development, especially since APIs are becoming the backbone of modern software infrastructures.
Real-World Applications of Flask APIs
(Up)Flask is the shit when it comes to building web apps and APIs. Major tech giants like Netflix and Pinterest are all over it, using it to power their security and scale their features.
If you wanna get on their level, check out this guide on creating your first Flask RESTful API. It's a solid starting point for getting into the backend game.
Flask is the go-to framework for startups and big corps alike.
Lyft uses it to handle high-traffic communication between their services, and Zalando switched to microservices powered by Flask's flexibility and speed. Even biotech companies like Ginkgo Bioworks are using Flask APIs to interface with complex lab equipment, showing its versatility beyond just tech.
The numbers don't lie – reports from Jetbrains and GitHub's dependency graph prove Flask's dominance in the web framework space.
You can even use it to deploy machine learning models as APIs, which is just crazy.
With a massive community and tons of support, Flask is the real deal for building APIs and putting your skills to work in the real world.
Conclusion: Why Choose Flask for API Development
(Up)Flask is the bomb when it comes to building APIs. It's lightweight and easy to use, but it still packs a punch. You know how it is, some people love it for how simple it is to get started, while others think it's not fast enough for big apps.
But hey, check out this comparison between Flask and FastAPI, it breaks it all down.
Flask's got a ton of cool extensions that make it super easy to add stuff like database support and create RESTful APIs. It's popular too, just look at what DEV Community says about how much control you get and how easy it is to test your code.
But they also warn about scaling issues and messy code if you're not careful. Flask is perfect for building microservices and serverless apps, which is like the future of web development, right? Around 30% of developers are already into that game, according to this article.
Flask lets you build complex back-end services for those kinds of apps. Even Nucamp has a guide on creating your first Flask RESTful API. So, in a nutshell, Flask is a solid choice for building APIs right now, and it's gonna keep being awesome as tech keeps evolving.
It's popular with startups and big companies alike, and it's just really versatile and efficient for web development.
Frequently Asked Questions
(Up)What is Flask known for in API development?
Flask is acclaimed for simplifying API development with its emphasis on scalability, modularity, and pluggable nature. It is widely used for its flexibility and ability to rapidly deploy small to sizable web applications.
How does Flask interact with databases in API development?
Flask synergizes with tools like SQL Alchemy for seamless database interactions, making it a prime choice for data serialization and manipulation in API projects.
Why is testing acumen important in Flask for API development?
Flask's testing acumen and compatibility with Python libraries showcase its maturity in building maintainable APIs. Testing ensures quality and reliability of API functionalities.
What are some advanced concepts for Flask API development?
Advanced concepts for Flask API development include leveraging tools like Werkzeug for handling requests, ensuring strong user authentication with extensions like Flask-HTTPAuth and Flask-JWT, and implementing CI/CD pipelines for automated testing.
What real-world applications demonstrate the effectiveness of Flask APIs?
Flask is utilized by tech entities like Netflix, Pinterest, Lyft, and Zalando for various applications, showcasing its efficiency in scaling, feature development, inter-service communications, and interfacing with advanced infrastructure beyond conventional tech boundaries.
You may be interested in the following topics as well:
Implementing crucial performance upgrades in your Flask apps can significantly enhance user satisfaction.
Discover the importance of web application security, a foundational aspect of any online service, especially when using Flask.
Continue your learning beyond this article with additional Flask API resources, curated for aspiring developers.
Keep your corner of the web fresh with insights into efficient Flask App Updating post-deployment.
Master the art of troubleshooting with the Flask Debug mode, a convenient feature to detect and solve issues swiftly.
Step into the world of professional web development by deploying your Flask app for the world to see and use.
Make an informed decision with our detailed Flask and Django comparison, and pick the perfect tool for your next project.
Learn how the Flask framework interfaces with databases to power dynamic, data-driven websites.
Navigate through the complexities of overcoming Flask Extension challenges with confidence in your next project.
Ludo Fourrage
Founder and CEO
Ludovic (Ludo) Fourrage is an education industry veteran, named in 2017 as a Learning Technology Leader by Training Magazine. Before founding Nucamp, Ludo spent 18 years at Microsoft where he led innovation in the learning space. As the Senior Director of Digital Learning at this same company, Ludo led the development of the first of its kind 'YouTube for the Enterprise'. More recently, he delivered one of the most successful Corporate MOOC programs in partnership with top business schools and consulting organizations, i.e. INSEAD, Wharton, London Business School, and Accenture, to name a few. With the belief that the right education for everyone is an achievable goal, Ludo leads the nucamp team in the quest to make quality education accessible