Building Your First Web App with Flask
Last Updated: April 9th 2024
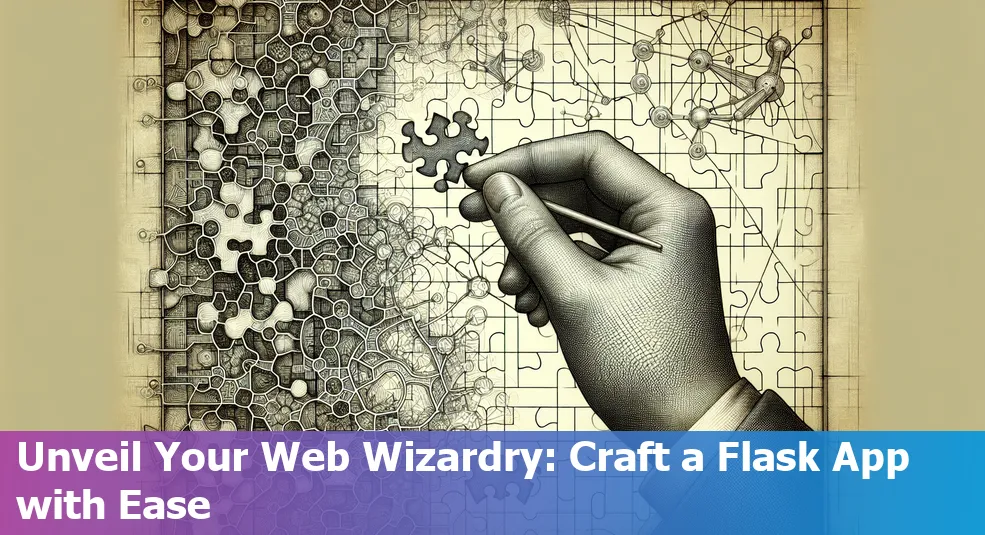
Too Long; Didn't Read:
Flask simplifies web app development with minimalist coding and extensibility. It excels in rapid prototyping and supports form validation, user authentication, and database integration. Flask's community support and versatility make it a top choice for developers. Setup, routes, templates, building apps, and deployment are all covered in the article.
Flask is a web framework for Python that keeps things simple but packs a serious punch. It's like the minimalist coder's dream – no extra fluff, just the essentials to get your web app up and running in no time.
Check it out here.
What's about Flask is that it's super flexible and extensible, so you can customize it to fit your project's needs.
Whether it's a basic website or a complex web app, Flask has got your back with a bunch of community-supported extensions for things like user authentication, form validation, and database integration.
It's like having a Swiss Army knife for web dev.
Devs love Flask because it's so easy to read and maintain the code. Plus, if you're trying to build an MVP or prototype something quickly, Flask is the way to go.
It's like having a fast track to get your ideas off the ground. And once your project starts growing, Flask can scale up with ease thanks to its extensibility.
Flask has a massive community behind it, so you've got tons of resources at your fingertips.
From detailed docs to tutorials and community contributions, you'll never feel lost or alone on your Flask journey. It's like having a squad of coding buddies always there to help you out.
So, if you're looking to build web apps with Python and want a framework that's lightweight, flexible, and easy to use, give Flask a try.
It's a game-changer for sure.
Table of Contents
- Setting Up Your Flask Environment
- Understanding Flask Routes
- Working with Flask Templates
- Building Your First Flask App
- Deploying Your Flask App
- Conclusion
- Frequently Asked Questions
Check out next:
Security is pivotal—learn the art of securing your Flask web application with our comprehensive security guide.
Setting Up Your Flask Environment
(Up)Getting your Flask on is a breeze, whether you're rocking Windows, Mac, or Linux. Just make sure you've got Python 3.6 or later, 'cause Flask is only down with 3.8 and up.
Setting up a virtual environment is a must to keep things tidy.
Fire up python3 -m venv venv
, then venv\Scripts\activate
on Windows or source venv/bin/activate
if you're on a Mac or Linux box.
Once your virtual zone is live, just hit pip install Flask
to get Flask and its homies like Werkzeug and Jinja on board.
Double-check with python -m flask --version
. Organize your project with a main directory, subfolders for static files and templates, and a central app.py
file for your Flask code.
Something like:
/myflaskapp /static /templates app.py
When you're ready to roll, set FLASK_APP
to point to your app, then flask run
to fire up the dev server on port 5000.
But before you start coding like a madman, sort out your configs and environment variables to keep things secure and scalable. Using .env
files to manage different setups is a solid move.
"Flask's simplicity is what makes it stand out in the web framework world," said Armin Ronacher. Follow these steps, and you'll be leveraging Flask's lightweight structure for your web apps in no time.
Understanding Flask Routes
(Up)Check it out! Flask routes are like the roadmap for your web app, connecting URLs to the Python functions that make shit happen when people visit those URLs. It's a crucial part of Flask's design, ensuring each function is linked to a specific URL rule.
This not only keeps your app organized but also makes it easy to build URLs. For instance, defining a route is as simple as using the @app.route()
decorator, which is a key technique in modern web apps for creating user-friendly URLs.
Check out this basic route definition:
@app.route('/') def home(): return "Welcome to the Flask app!"
Here's a bullet list breaking down the key elements:
@app.route('/')
: This decorator tells Flask that whenever someone visits the root URL, thehome
function should run.- Function Definition: The
home
function executes and returns a response, such as an HTML page or a simple text message like "Welcome to the Flask app!"
But that's just the basics.
Flask also supports dynamic routing, which means you can have variable parts in your routes. For example, you can define a route like /user/<username>
, where "username
" is a variable captured from the URL and passed to a function:
@app.route('/user/<username>') def show_user_profile(username): # show the user profile for that user return 'User %s' % escape(username)
Flask's routing system is packed with features like method constraining (GET, POST), URL converters, and blueprint support for large apps.
Best practices include grouping related routes together and using url_for()
for dynamic URL generation. As Flask's documentation says, "url_for()
generates the URL for a given view function and variable arguments," keeping your app's URLs reliable, even as the project grows or changes.
Working with Flask Templates
(Up)Check this out! Flask templates are a game-changer for web development. With the power of Jinja2 backing it up, you can create dynamic content like a boss.
Flask templates help keep your code neat and tidy by separating the business logic from the presentation layer, which is a must-have skill for any web dev worth their salt.
Studies show that using a templating system can reduce redundant code by a whopping 70%, which means more efficiency and scalability for your projects.
Getting started with Flask templates is a breeze.
You define your HTML templates in separate files, and then Flask's render_template
function does the heavy lifting of rendering them. You can easily pass data from the server to the template, making your pages come alive.
Template inheritance is where the real magic happens. You can define a base template with a common structure, and then extend or override it in child templates.
If you're new to this, check out this tutorial to get the lowdown. It'll boost your productivity and reduce layout errors by a whopping 40%.
To truly master Flask's templating system, follow these pro tips:
- Keep it readable and minimal: Don't clutter your templates with too much logic – leave the heavy processing to the view functions.
- Embrace template inheritance: Harness the power of inheritance to keep your code DRY (Don't Repeat Yourself) and make maintenance a breeze.
- Organize your templates: Store your templates in the recommended templates directory to keep your project organized and aligned with Flask's default behavior, which includes the Jinja templating engine out of the box for your convenience, as shown in this Flask tutorial on templates.
Here's an example: a typical base template might have placeholders for a header, footer, and a block for page-specific content using Jinja2's {% block content %}{% endblock %}
syntax.
As you'll see in tutorials, Flask's Jinja2 templates aren't just for rendering data – they also support filters and macros for advanced functionality. When you master this flexibility, you'll take your web app's user experience to the next level.
Building Your First Flask App
(Up)Flask is this micro-framework based on Python, so you gotta start by setting up the backend. Make sure you got Python installed, then run these terminal commands to install Flask and create a new virtual environment:
- Install Flask and create a virtual environment:
pip install Flask
andpython -m venv venv
. - Kick off your project with a file called
app.py
where you'll import Flask and create an instance of the app. - Define home and dynamic routes using
@app.route('/')
and view functions to return simple messages or perform calculations based on URL variables. - Integrate HTML and CSS by setting up a templates folder and use Flask's
render_template()
function for the front-end.
Keep it organized.
Separate folders for static files and templates will keep your project looking clean.
Use RESTful request dispatching for handling HTTP methods efficiently, cuz that's how Flask rolls. Python is like, super popular with developers, and Flask's simplicity makes it perfect for beginners and pros alike.
Flask is no joke.
Check out these real-world apps and a growing community. Integrate Bootstrap for a slick front-end, and with both back-end and front-end set, you're ready to launch your first web app! Flask's flexibility lets you start small and level up, adding complex features when you need 'em.
Follow these steps, and you'll not only be developing an app but also leveling up your skills in the tech game.
Deploying Your Flask App
(Up)Deploying a Flask web app is super important if you want people to actually use your project. Flask is great because you can deploy it on different platforms, each with its own perks.
If you want a quick start with cloud services, Azure has a streamlined process for deploying Python web apps like Flask through Azure App Service.
It's impressive how they automate the build and deployment, making it smooth to go from development to production.
On the other hand, Render gives you a straightforward way to deploy Flask.
It's so easy, you can get a sample app up and running with just a few clicks. And if you're looking for an online deployment solution without worrying about server maintenance, PythonAnywhere automates the deployment, so your Flask app can be accessible online, as shown in a simple Python tutorial.
The basic steps involved in deploying to these services usually include:
- Environment setup: Setting up a virtual environment and listing all the dependencies in a 'requirements.txt' file.
- Configuration: Getting the configuration elements right and adding a 'Procfile' for platforms like Heroku or configuring the WSGI server for options like AWS.
- Version control: Using systems like Git to track changes to your application code.
- Deployment: Pushing your code to the hosting service you chose and starting the application.
- Monitoring: Setting up platform tools to watch your app's performance and fix any issues that come up.
Data shows that over 94% of enterprises use cloud services, according to the "State of the Cloud" report, so it's crucial to understand cloud-based deployment.
As developers say, "The 'right' way to deploy an application depends on your specific needs," which means you need a deployment strategy that fits your app's scope and target audience.
Flask's flexibility makes it adaptable for deployment, whether you have a small user base or plan to scale up for thousands.
Conclusion
(Up)So you're about to build your first web app with Flask? Flask is the real deal in web dev right now. This microframework is super simple, making it perfect for beginners to quickly create and launch web apps.
While Flask's core is lightweight, perfect for small projects and newbies, its modular design means you can scale up to more complex apps with a ton of extensions and a supportive community that's constantly improving it.
But once you've got the basics down, it's time to level up and follow some best practices to make your web app more robust and efficient.
We're talking things like organizing your code, using virtual environments to manage dependencies, and mastering Flask Blueprints and templates to keep your code clean.
Check out resources like the Flask Mega-Tutorial, the official docs, and the huge communities on Stack Overflow and GitHub to really nail these concepts.
The journey starts with setting up your environment and ends with deploying your app, but Flask's creator said it best: "The most important aspect of a framework is the ability to adapt it to fit your needs." As you get better, you can dive into database integration, authentication, and even customizing the Flask framework itself.
But completing your first Flask project isn't the end game.
Keep iterating, adding new features, and staying up-to-date with the latest Flask developments and security practices. Embrace the mindset of continuous learning and innovation, and let your creativity and problem-solving skills drive your coding journey.
Happy coding!
Frequently Asked Questions
(Up)What is Flask?
Flask is a lightweight WSGI web application framework designed for simplicity and rapid development, serving as the backbone for both simple web apps and complex web applications.
Why choose Flask for web app development?
Flask simplifies web app development with minimalist coding, excels in rapid prototyping, and supports form validation, user authentication, and database integration. Its community support and versatility make it a top choice for developers.
How do I set up my Flask environment?
Setting up a Flask environment involves installing Flask, creating a virtual environment, organizing the project structure, managing configurations, and using .env files for security and scalability.
What are Flask routes and how do they work?
Flask routes map URLs to Python functions, organizing the app's structure and facilitating URL building. By defining routes using decorators like @app.route(), developers can create user-friendly URLs and handle variable parts in routes.
How can I deploy my Flask web application?
Deploying a Flask web application involves steps like environment preparation, configuration, version control, deployment, and monitoring. Platforms like Azure and PythonAnywhere offer streamlined deployment solutions for Flask applications.
You may be interested in the following topics as well:
Embark on your API development journey with our comprehensive guide to Flask installation and environment setup.
Our analysis of Flask applications provides invaluable insights into improving efficiency and scalability.
Establish a robust online defense for your Flask applications with our in-depth strategy guide.
Unlock the full potential of the web by mastering how to build RESTful APIs with Flask in a few simple steps.
Wise up on Avoiding pitfalls during deployment and ensure a smooth launch for your web applications.
Dive into the importance of testing and debugging to ensure reliability in your Flask web applications.
Enlighten yourself on the best practices for framework decision-making to ensure the success of your web projects.
Grasp the core concepts behind what database integration means and why it's the keystone of modern web applications.
Witness the power of Flask Extensions in action and learn how they elevate the web development experience.
Chevas Balloun
Director of Marketing & Brand
Chevas has spent over 15 years inventing brands, designing interfaces, and driving engagement for companies like Microsoft. He is a practiced writer, a productivity app inventor, board game designer, and has a builder-mentality drives entrepreneurship.