Python for Data Structures: An In-Depth Guide
Last Updated: April 9th 2024
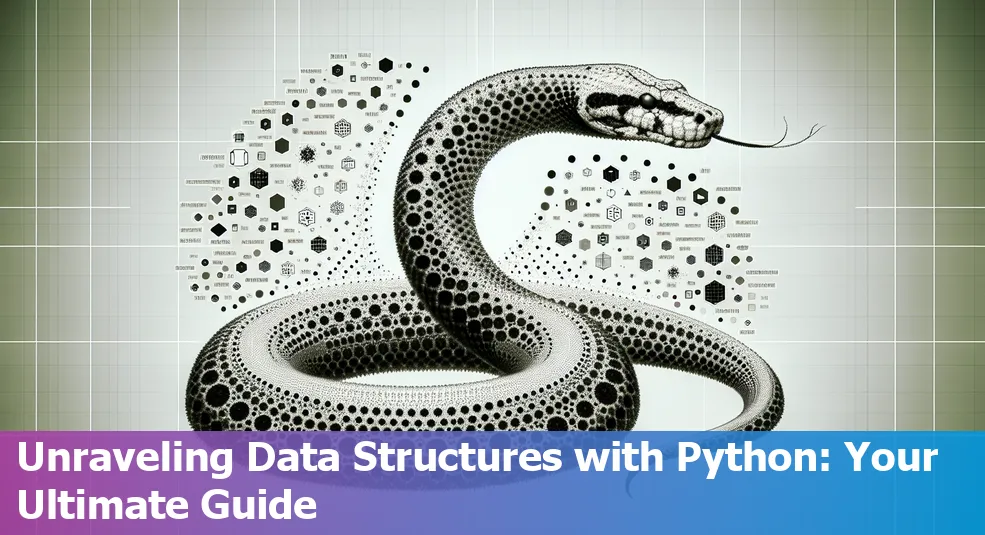
Too Long; Didn't Read:
Python's simplicity vs. complexity debate for data structures is explored. Python's dynamic typing aids beginners, but might obscure complex principles. Still, Python's libraries like NumPy shine. Python leads in education and TIOBE Index. The article delves into data structures, Python's built-ins, and real-world applications.
Python is like the universal language for working with data, but not everyone agrees it's the best way to learn data structures. Some people on sites like Quora argue that Python's simplicity makes it too easy, and you might miss out on some of the deeper concepts that more complex languages like Java can teach you with their stricter typing rules.
But on the other hand, Python's flexibility and interpreted nature let you jump right in and quickly play around with basic data structures like lists and dictionaries without getting bogged down in complex setup.
Regardless of the debate, Python's clean syntax and powerful libraries like NumPy and Pandas make it a go-to language for working with data efficiently.
Its versatility shines through in our Nucamp articles, where we explore how Python can streamline everything from network programming to big data analysis.
Plus, Python's widespread use in schools and universities worldwide shows how important it is for learning data structures.
Its consistent top ranking in the TIOBE Index also proves that developers recognize Python's value for both learning and practical applications.
Table of Contents
- Understanding Data Structures
- Python and its in-built Data Structures
- Implementing Data Structures in Python
- Real-World Applications of Python Data Structures
- Conclusion
- Frequently Asked Questions
Check out next:
Step into the future of technology by learning how to leverage Python and machine learning to create intelligent systems.
Understanding Data Structures
(Up)Data structures are like the building blocks of programming. They're all about organizing and storing data in a way that makes your code run smoothly and handle complex tasks with ease.
Understanding data structures is crucial if you want to be a pro developer, because they determine how fast and efficient your apps run. Algorithms for sorting and searching can have drastically different runtimes depending on the data structure used, and companies like Amazon and Microsoft love to test your knowledge of data structures in interviews to see if you can write code that scales well.
There are tons of data structures out there, but some popular ones that every programmer should know include:
- Arrays - These are like fixed-size containers that are great for storing and accessing data using indexes, especially when you're dealing with the same data types.
- Linked Lists - Made up of nodes, these are super flexible and allow you to insert and delete data without worrying about physical memory constraints.
- Hash Tables - These give you lightning-fast access to data using unique keys, perfect for creating database indexes and other speedy lookups.
- Stacks - Following the Last In, First Out (LIFO) rule, stacks are the go-to choice for backtracking operations like undo functions in software.
- Queues - Working on the First In, First Out (FIFO) principle, queues are essential for managing sequences, from task scheduling to buffering data streams.
The key is to pick the right data structure for the job.
Arrays are great for fast access, but linked lists can handle dynamic sizes better. An intro tutorial like Great Learning's beginner guide can show you when to use each one, like how a stack is perfect for parsing expressions or how a priority queue can manage processor time for an operating system scheduler.
Bottom line, learning data structures is crucial if you want to create software that's efficient, reliable, and can handle complex tasks without breaking a sweat.
With the right data structure, you can minimize memory usage, boost data processing speeds, and make sure your code can handle whatever you throw at it.
Python and its in-built Data Structures
(Up)Python is all about keeping things simple yet powerful. It comes packed with dope data structures that can handle pretty much any coding challenge you throw at it.
The main players are lists, tuples, sets, and dictionaries.
Lists are like flexible squads that can change up their members over time, and they're perfect for list comprehensions, which make manipulating and looping through them a breeze.
Tuples, on the other hand, are like locked-down crews that can't be messed with.
They're ideal when you need to keep your data secure and untampered. Dictionaries work with hash tables, which means they can retrieve data like lightning with a time complexity of O(1)
, as explained in this overview of Python data structures.
This speed is clutch when dealing with big data and analytics, where hashing can seriously speed up the process. Sets are all about keeping things unique and unordered, making set comprehensions and operations like union and intersection super powerful tools.
When it comes to comparing these sturdy structures, it's clear:
- With their ability to manipulate and handle conditions and loops like bosses, lists and sets offer mad versatility.
- Tuples are the solid choice for fixed collections, emphasizing immutability.
- For tasks involving mapping key-value pairs, dictionaries rule with their efficiency.
The criteria determine which data structure to pick in Python.
Lists are the go-to for mutable, ordered grouping, while tuples are perfect for constant records, and dictionaries are unbeatable for associations requiring lightning-fast access.
Dictionaries are so useful that nearly 75% of Python users rely on them—a stat backed by Python's core philosophy.
Whether you're into data analysis, machine learning, or network programming, Python's data structures are the backbone, making the language a powerhouse across diverse domains.
Implementing Data Structures in Python
(Up)Let me lay it down for you about data structures in Python. It's a whole vibe, trust me. First up, we got stacks, and they're like the OG data structure.
You can make one just by using Python's built-in list
, with append()
and pop()
acting as your push and pop operations. It's all about that LIFO life (Last In, First Out), feel me? Runestone Academy breaks it down, showing that append()
and pop()
are both O(1), meaning they're mad efficient.
And if you need more stack goodness, Codecademy has your back with tips on checking the stack size with len()
and adding a peek()
function.
Plus, Steve Grice on Medium gives you the deets on making node-based stacks for different use cases.
Now, when it comes to more complex structures like trees, you gotta go with the class-based approach.
A binary tree, for example, involves creating a class with attributes for the root node and its left and right children. The tutorials show that Python's object-oriented nature makes it easy to represent nodes and sub-trees, and you can use recursive functions to visualize them.
Python's dynamic typing and memory management also make the process smooth as butter. For linked lists, Python's pointer-like references between objects let you create nodes that link to other nodes, forming singly or doubly linked lists.
Python's adaptability is on another level, handling advanced Python techniques for data analysis, scripting, and network programming like a boss, as Nucamp Coding Bootcamp's curriculum shows.
It's no wonder graphs and heaps are also a breeze in Python with third-party libraries like NetworkX and heapq.
Graphs use Python dictionaries and lists to store vertices and edges, making it easy to represent and traverse them. Heaps are perfect for priority queues, and the heapq module turns a regular list into a heap structure, where the smallest element can always be quickly popped.
Python's skills are off the charts, even handling big data analytics and web development with Flask.
The heapq operations have an O(log n) time complexity, proving that Python can handle both basic and complex algorithmic challenges across different sectors like a champ.
Real-World Applications of Python Data Structures
(Up)This Python thing is hella dope, dawg. It's like the secret weapon for all the coolest apps and tech out there. Imagine Netflix or Spotify without Python's data structures – those personalized recommendations you get would be whack.
So, what're these data structures, you ask? They're like containers that let you organize and manipulate data in different ways.
You got lists, which are like numbered boxes for storing stuff. Dictionaries are key-value pairs, perfect for matching things up. Sets are unique collections, no duplicates allowed.
And tuples are immutable, meaning you can't change 'em once they're set.
Python's data structures are the backbone of machine learning, which is like teaching computers to learn on their own.
Around 58% of data scientists use Python regularly, according to some survey. In finance, over 70% of quant analysts rely on Python for trading and analysis. Even big dogs like JPMorgan Chase use Python in their trading platforms.
Web development wouldn't be the same without Python's dictionaries managing HTTP requests and user sessions.
Instagram uses 'em to handle millions of users. And in science, Python structures experimental data for libraries like NumPy and SciPy. Even the European Space Agency uses Python to automate their satellite systems, which is hella dope.
Network security giants like Cisco script their automation tools with Python's data structures, proving how versatile this language is.
One dev said Python is more than just a language – it's a tool for solving problems, and that's straight facts. Python's data structures are woven into modern tech like a boss, making them essential across all kinds of applications.
Conclusion
(Up)Python's the real MVP when it comes to handling all kinds of data structures, and that's why it's been the go-to tool for data management and analysis tasks. Check out Pandas, a killer library that makes working with labeled data structures a breeze, just like R's data.frame objects.
As we roll into 2023, Python's popularity with data structures is only going up for some solid reasons:
- Its clean syntax and readability make it a breeze to manage complex data structures, saving you time and reducing errors.
- Python's got a ton of awesome libraries like NumPy and Pandas, making it a powerhouse for data analysis and letting you get creative.
- The active community behind Python is constantly updating and adding new modules and frameworks, making it even easier to work with data structures.
When it comes to data management, Python's momentum is unstoppable.
It's been rocking the top spot on the TIOBE Index for March 2023, proving its edge when dealing with complex data structures.
"Python's the real deal for data-intensive applications, thanks to its simplicity, efficiency, and sick libraries." - Data Science Industry Expert
With the rise of big data and machine learning, Python skills are in high demand.
Job postings requiring Python expertise for data handling saw a 17% surge from 2022 to 2023. Python's constant evolution, adaptability, community support, and integration into apps like Excel cement its reign as the king of data structures.
Frequently Asked Questions
(Up)What are some common data structures in programming?
Common data structures in programming include arrays, linked lists, hash tables, stacks, and queues. These structures are chosen based on the specific needs of an application.
How does Python handle data structures?
Python provides built-in data structures like lists, tuples, dictionaries, and sets. Each structure has its own characteristics and advantages, allowing developers to efficiently manage and manipulate data.
How can data structures be implemented in Python?
Data structures can be implemented in Python using built-in structures like lists or with a class-based approach for more complex structures like trees. Python's dynamic typing and memory management simplify the implementation process.
What are some real-world applications of Python data structures?
Python data structures are used in various real-world applications such as machine learning, finance, web development, network security, and scientific research. Python's versatility and efficiency make it a preferred choice for handling diverse datasets.
Why is Python preferred for data structures?
Python's simplicity, readability, and extensive library support make it a popular choice for working with data structures. Its clean syntax reduces development time and error rates, promoting innovation and efficiency in data analysis.
You may be interested in the following topics as well:
Uncover the myriad of benefits of Python and database integration that can streamline your development process.
Learn about the robust Python ecosystem that supports both beginners and experienced developers with tools and libraries.
Start your Machine Learning journey by setting up the environment with Python and familiarizing yourself with its extensive ecosystem.
Unlock the powers of robust error handling to elevate the quality of your Python projects.
Get expert guidance on Choosing Flask or Django for your next web development project.
Unpack the full potential of Python's role in data science, an indispensable tool for modern analysis techniques.
Explore the Essential Python Libraries that are revolutionizing Network Programming, one script at a time.
Learn why Python for automation is the go-to choice for developers seeking efficiency and scalability.
Learn how to speed up your Python applications with practical optimization strategies.
Ludo Fourrage
Founder and CEO
Ludovic (Ludo) Fourrage is an education industry veteran, named in 2017 as a Learning Technology Leader by Training Magazine. Before founding Nucamp, Ludo spent 18 years at Microsoft where he led innovation in the learning space. As the Senior Director of Digital Learning at this same company, Ludo led the development of the first of its kind 'YouTube for the Enterprise'. More recently, he delivered one of the most successful Corporate MOOC programs in partnership with top business schools and consulting organizations, i.e. INSEAD, Wharton, London Business School, and Accenture, to name a few. With the belief that the right education for everyone is an achievable goal, Ludo leads the nucamp team in the quest to make quality education accessible