Automated Testing in Django: A Beginner's Guide
Last Updated: June 5th 2024
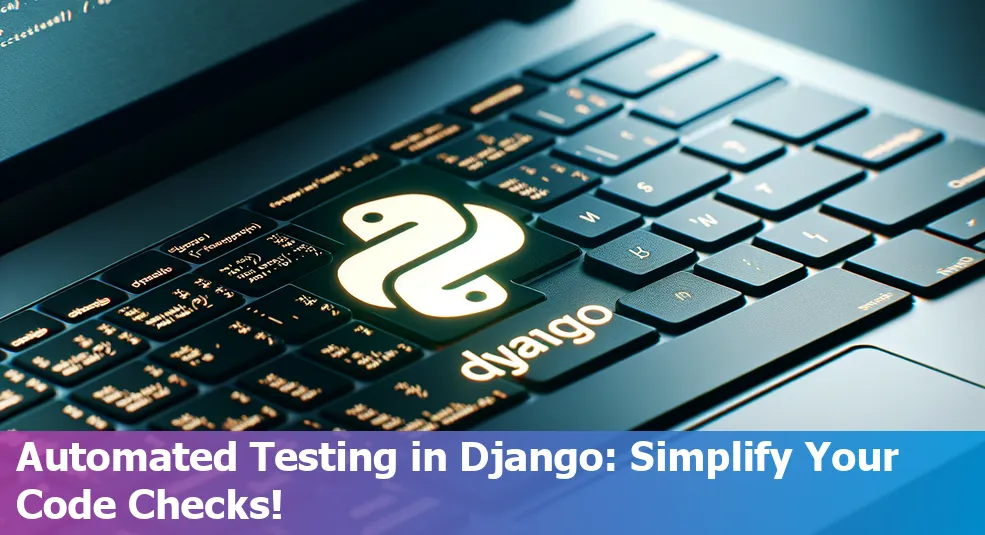
Too Long; Didn't Read:
Django offers a robust testing framework for automated testing, minimizing manual intervention. Embrace automated testing to ensure code quality, prevent regressions, and streamline development. Beginners find Django's testing tools accessible. Automated testing reduces costs, improves defect detection, and is essential for web app quality and efficiency.
Let me break it down for you about this Django thing. It's a Python web framework that makes building websites and web apps a breeze. The creators are industry vets, so you know it's legit.
Django takes care of all the tedious stuff, allowing you to focus on coding your app effortlessly and with less lines of code. Best part? It's free and open-source, with a massive community backing it up.
When it comes to testing your code automatically to catch any bugs or issues, Django has a solid testing framework built right in.
It seamlessly integrates with your projects, making it a smooth process. As those Simplilearn folks point out, Django helps you maintain high code quality and keep things organized, thanks to its robust testing tools.
These tools let you check if your code is working as intended, prevent any regressions (when new code breaks existing functionality), document how your code should behave, and promote better software design overall.
If you're new to this, Django's testing setup is ready to go, so you don't have to jump through hoops to set it up.
Automating your tests is a game-changer, according to Capgemini's report. It cuts testing costs and helps catch more defects before they become major issues. Embracing automated testing is crucial for ensuring your Django web apps are top-notch.
Table of Contents
- Why Automated Testing is Important for Django Projects
- Understanding Django's Built-in Test Framework
- Writing Your First Automated Test in Django
- Common Mistakes in Django Automated Testing and How to Avoid Them
- Advanced Django Test Techniques
- Conclusion: Embrace Automated Testing in Your Django Projects
- Frequently Asked Questions
Check out next:
Secure user data and streamline access controls by learning about Django's authentication mechanisms in our definitive guide.
Why Automated Testing is Important for Django Projects
(Up)Check it out! Automated testing in Django projects isn't just some fancy feature, it's the real deal for keeping your web apps solid and reliable. This guy Jeff Knupp explains how simple and powerful Django's unittest framework is, and how it's essential for keeping your code intact.
The Django Software Foundation themselves recommend automated testing, saying it leads to more stable releases and supports agile development.
Running a full suite of tests before and after you make code changes, as Quintagroup suggests, is crucial because it catches bugs early, saving you time and money, and preventing software issues.
- Higher Quality Code: Django's testing tools give you a clear path to identify and prevent mistakes in your code, ensuring thorough coverage and helping you deliver higher-quality web apps.
- Efficient Refactoring: With automated testing, developers can confidently refactor and optimize, knowing the safety net of tests will catch any flaws they introduce.
- Time Savings: Once you set up tests, you can rerun them super fast, way faster than manual efforts—yep, automated tests can be much faster, as Mozilla's developer network points out.
- Improved Collaboration: By serving as functional documentation, automated tests enhance teamwork by providing a shared understanding of the system's expectations.
Not only that, but the integration of automated testing in Django web development fits perfectly with the framework's 'Don't repeat yourself' (DRY) philosophy, streamlining processes by reusing test cases.
Automated tests are a key part of Agile methodologies, complementing Django's approach to allow for consistent delivery of value to clients. It's pretty much essential for staying ahead in today's fast-paced software development game.
Understanding Django's Built-in Test Framework
(Up)Let me break it down for you in a way that makes sense. Django's testing tools are a game-changer for developers, making it way easier to write and run tests for your web apps.
It's like having a built-in safety net that ensures your code doesn't mess up your live data.
Here's the deal:
- Custom assertion methods let you check if your code is doing what it's supposed to do, down to the smallest detail.
- The 'client class' simulates real users interacting with your app, allowing you to test how it responds to different requests and URLs.
- Signal tracking helps you test asynchronous events, like when something happens in the background.
- You can integrate other testing libraries like 'pytest' or 'unittest.mock' to supercharge your testing capabilities.
Imagine you're testing a view in your app.
You'd use the test client to simulate a user request, then use assertion methods to verify that the response matches what you expect. But here's the catch: you'll choose between 'TestCase' and 'SimpleTestCase' based on whether your test involves the database or not.
TestCase | SimpleTestCase |
---|---|
For testing stuff that interacts with the database | For testing stuff that doesn't touch the database |
Slower since it's dealing with database activity | Faster since there's no database involved |
PATRICIA HERNANDEZ, one of Django's founders, put it best: "The goal of Django's testing framework is to make it painless to write, run, and maintain tests for your applications." They want to make testing as easy as pie, so you can focus on building awesome web apps that work flawlessly.
If you want to level up your Django skills for professional projects, check out the resources on Mastering Django.
Writing Your First Automated Test in Django
(Up)Writing your first automated test in Django is a big deal, like a coming-of-age moment for any aspiring dev. First things first, create a 'tests.py' file in your Django app—that's where your tests are gonna chill.
Check out the official Django testing guidelines to get started on the right foot. You'll need to import unittest and the models you wanna validate.
Start with the basics – make sure your model's str method is on point. Here's a quick rundown:
- Bring in the necessary libraries and models with
from django.test import TestCase
andfrom .models import YourModel
. Get the testing frameworks and models in place to set up the automated testing. - Create a new class ModelTests that extends TestCase. Set up a testing suite to hold your test cases.
- Inside ModelTests, write a function called
test_str_representation
. Add test functions to check if your model works as expected. - Create a model instance and fill in the fields with some data. Make instances to test how your model methods work.
- Use assertEqual to compare the expected string with
YourModel.__str__()
. Assert your expectations and see if the instance outputs match. - Wrap it up with a clean-up step
def tearDown(self): YourModel.objects.all().delete()
. Keep things tidy with a teardown procedure.
To run your masterpiece, just type python manage.py test
in the terminal.
If everything passes, your code is solid; if not, you know what needs some tweaking. As the pros say, tests are the backbone of fearless coding; they let you make changes and improve your software design without breaking everything.
By following these steps, you're not just writing a test, you're making your app more resilient. And if you wanna level up your testing game, check out the MDN Web Docs tutorial on Django testing to learn about different types of tests.
With tests on your side, you're not just a dev, you're a coding superhero!
Common Mistakes in Django Automated Testing and How to Avoid Them
(Up)When it comes to automated testing in Django, you gotta watch out for some common pitfalls to keep your code running smooth. Like, one rookie mistake is writing tests without really knowing what you're trying to achieve.
To avoid that, make sure you have clear testing goals and a solid understanding of what your app needs to do. Also, don't sleep on edge cases - those sneaky little scenarios that can mess up a big chunk of your production code if you're not careful.
Use a checklist to cover all the usual edge case suspects.
Everyone agrees that clean code is the way to go - 90% of developers get that. But not everyone takes the time to keep their tests spick and span.
Doing that helps you avoid code duplication and makes your tests easier to read. Another common trip-up is not keeping your tests isolated. When tests aren't independent, you end up with flaky results.
Use Django's @isolate
decorator to keep your tests running separately. It's kinda wild that only 37% of developers are taking advantage of Django's testing tools like Client
and RequestFactory
.
Those are super handy for writing solid tests.
You might run into weird errors like the "Module Not Found" thing. That's usually just a matter of following Django's naming conventions and folder structure for tests.
Testing shouldn't be an afterthought - it's a crucial part of the development process. When you get good at it, your tests not only catch regressions but also make your code more resilient and less prone to breaking.
To sum it up, here's how you can dodge common testing pitfalls:
- Start with clear testing goals so you know what you're aiming for.
- Use checklists for edge cases to cover all your bases and avoid silly mistakes.
- Keep your tests clean and tidy to avoid duplication and keep things readable.
- Keep tests independent to avoid flaky results and use
@isolate
when needed. - Leverage all of Django's testing tools to write better tests.
Follow these tips, and your automated tests will not only catch regressions but also keep your code solid as a rock.
Advanced Django Test Techniques
(Up)Let me break it down for you real quick. As you start building more complex web apps with Django, testing becomes a game-changer. This beginner's guide, "Django Testing for Beginners," makes it easy to understand why writing tests early on can save you from a ton of headaches and bugs down the line.
But as your app gets more intricate, you gotta level up your testing game too.
Integration tests are crucial for making sure all the different parts of your Django app play nice together. Check out "Advanced Django Features You Need to Know" to see how Django's testing capabilities can handle complex scenarios like middleware, signals, and Class-Based Views.
One advanced technique you'll want to master is using mock objects and patching.
This lets you test individual components without worrying about all the real-world complexities. Django's unittest.mock
module is your best friend here, and it can save you a ton of testing time.
And if you're worried about performance, Django's TestCase
classes got you covered for load testing and making sure your app can handle the traffic.
As your app grows, you'll need to adopt some best practices.
Split your tests into smaller units for easier debugging, and run them in parallel (Django supports that). Continuous integration (CI) is a must – check out "Optimizing Development" by Nucamp Coding Bootcamp for more deets.
And don't forget to refactor your code regularly and use Django's override_settings
decorator to switch settings during tests.
"Incorporating advanced testing techniques sets apart proficient Django developers, ensuring applications are not just functional but resilient and scalable."
The more you level up your testing skills, the better equipped you'll be to tackle the challenges of modern web development.
Conclusion: Embrace Automated Testing in Your Django Projects
(Up)Let me break it down for you about automated testing in Django - that's a total game-changer! According to Merixstudio's blog, it's like having a friend who's always got your back.
It keeps things agile, gives you a solid return on your investment, and lets you run tests 24/7, so you can keep pumping out quality code like a pro.
Industry studies show that automated testing in Django can cut down your development time by like 15-20%.
And with Nucamp's suite of tools, you can automate that testing game and make sure your deployments are on point.
These tools, plus the skills to rock Django, will help you catch errors faster than you can say "bug" and take the pressure off your QA team.
Adopting automated testing in Django is a total power move for your project's success.
It'll keep things consistent by cutting out human error and spotting issues early, just like Testim.io's analysis says.
And with over 70% of devs worried about refactoring, automated testing lets you optimize your code with confidence. Plus, Django's test environment mimics the real deal, so you can deploy with the peace of mind that automated testing has your back.
But it's not just about preventing fails.
Studies from BrowserStack show that teams using automated testing seriously reduce downtime caused by bugs.
That's the kind of value you can't ignore. So don't sleep on automated testing in Django - it'll take your web apps to new levels of reliability and efficiency, guaranteed.
Let it be the wind beneath your project's wings, and watch it soar!
Frequently Asked Questions
(Up)Why is Automated Testing Important for Django Projects?
Automated testing in Django projects ensures robustness and reliability of web applications. It leads to higher quality code, efficient refactoring, time savings, and improved collaboration. Additionally, automated testing aligns with Django's 'Don't repeat yourself' (DRY) philosophy, streamlining processes and supporting Agile methodologies.
What are Common Mistakes in Django Automated Testing and How to Avoid Them?
Common mistakes in Django automated testing include lacking clear testing goals, overlooking edge cases, neglecting code cleanliness, and not ensuring test case independence. To avoid these, developers should clarify testing intentions, use edge case checklists, refine tests to eliminate redundancy, ensure test case independence, and utilize Django's testing facilities effectively.
How can Developers Use Advanced Django Test Techniques?
Developers can utilize advanced Django test techniques by incorporating integration tests, mock objects, patching, performance testing, and best practices such as breaking down tests into smaller units, running tests in parallel, practicing continuous integration, and using Django's override_settings decorator for flexible settings changes during test runs.
How to Write Your First Automated Test in Django?
To write your first automated test in Django, create a 'tests.py' file in your Django app. Integrate necessary libraries and models, define a testing suite, write test functions to verify model behavior, create model instances to test methodology, use assertEqual to compare expected outputs, and ensure a clean state through teardown procedures. Run the tests using 'python manage.py test' in the terminal.
Why Should Developers Embrace Automated Testing in Django Projects?
Developers should embrace automated testing in Django projects to ensure consistent results, reduce human error, identify issues early, optimize code safely, enhance code quality, and streamline development and deployment processes. Automated testing brings tangible value by mitigating downtime related to faults, sustaining high-quality standards, and hastening the development and deployment of web applications.
You may be interested in the following topics as well:
Learn how Django ORM Streamlines backend development, allowing you to focus more on your application's functionality.
Dive deep into how Django Models and Databases work together to manage your app's data effortlessly.
Stay ahead of cybersecurity threats by learning how attackers target Django applications.
Explore what the future of full-stack development looks like with Django leading the charge.
Experience a significant Speed Boost for Django Applications with simple yet effective optimization techniques.
Explore advanced security practices to take your Django app's authentication to the next level.
Gain insights into Django's Professional Edge and see how businesses succeed with these robust tools.
Unlock the potential of Django's full-stack capabilities for a comprehensive approach to scalable web applications.
Explore E-commerce sites powered by Django through various success stories and case studies.
Ludo Fourrage
Founder and CEO
Ludovic (Ludo) Fourrage is an education industry veteran, named in 2017 as a Learning Technology Leader by Training Magazine. Before founding Nucamp, Ludo spent 18 years at Microsoft where he led innovation in the learning space. As the Senior Director of Digital Learning at this same company, Ludo led the development of the first of its kind 'YouTube for the Enterprise'. More recently, he delivered one of the most successful Corporate MOOC programs in partnership with top business schools and consulting organizations, i.e. INSEAD, Wharton, London Business School, and Accenture, to name a few. With the belief that the right education for everyone is an achievable goal, Ludo leads the nucamp team in the quest to make quality education accessible