Django ORM: Mastering Database Management
Last Updated: June 5th 2024
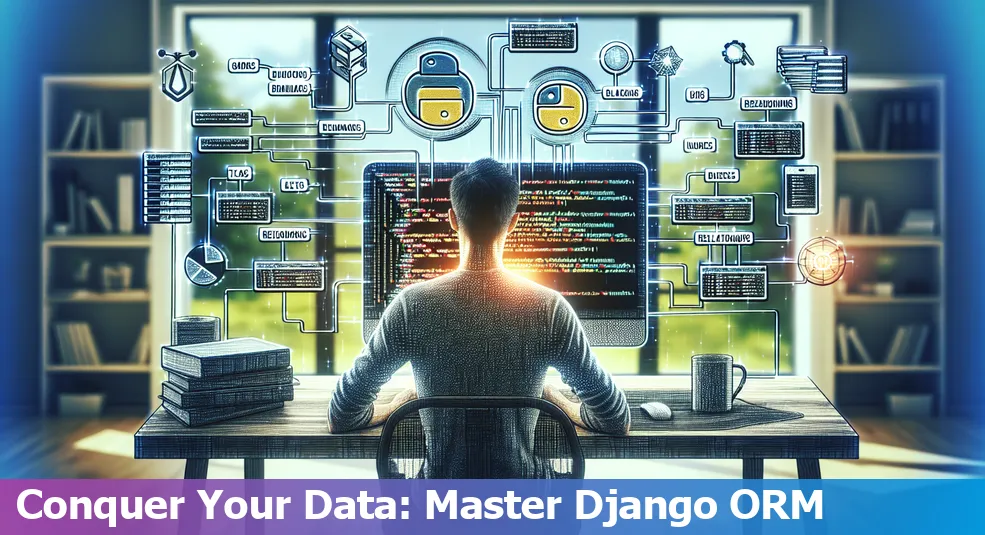
Too Long; Didn't Read:
The Django ORM simplifies database management by translating Python code into SQL, supporting various databases, migration systems, and optimized data retrieval. While it may have performance trade-offs compared to raw SQL, its efficiency, scalability, and ease of use make it a valuable tool for web development.
The Django ORM is a game-changer for all you code monkeys out there. It's like a translator between you and the database, making sure your Python code gets turned into SQL without you having to break a sweat writing all that syntax.
This bad boy works with all the popular databases like PostgreSQL, MySQL, SQLite, and even Oracle, so you're covered no matter your preference.
One of the coolest features of this ORM is its migration system.
It's like a smooth operator, helping you switch databases or change your schema without any drama. With Querysets, you can retrieve data exactly how you want it, from the simplest queries to the most complex ones.
Django's got your back.
The ORM is a boss when it comes to CRUD operations (that's Create, Read, Update, and Delete for all you newbies). It's built for scalability, which means your app can grow like crazy without breaking a sweat.
The folks at Nucamp know what's up – they're always raving about how Django can handle applications in all kinds of industries.
Stick around, because we're gonna dive deep into the Django ORM's features, see how it stacks up against writing SQL by hand, and get some pro tips on how to use it like a boss.
This ORM is a game-changer, and you don't want to miss out on all the goodness it brings to the Django framework.
Table of Contents
- Features of Django ORM
- ORM vs SQL: Comparing Performance
- Mastering Django ORM with Hands-On Examples
- Common Mistakes to Avoid in Django ORM
- Conclusion
- Frequently Asked Questions
Check out next:
Learn the art of building scalable Django applications that grow with your audience and your business needs.
Features of Django ORM
(Up)The Django Object-Relational Mapping (ORM) is the superhero of the Django web framework.
It's the bridge that connects your data models to the database, and it's awesome!
Instead of dealing with SQL code, which can be a real pain, the Django ORM lets you write Python code to create, read, update, and delete database records.
It's like having your own personal translator who understands both Python and SQL. Plus, it helps protect you from those pesky SQL injection attacks, so you can code without worrying about security vulnerabilities.
The Django ORM is compatible with all the popular databases out there, like PostgreSQL, MySQL, SQLite, and Oracle.
So, you can switch between databases like a pro, without having to rewrite your entire codebase. And don't even get me started on the query optimization features, like lazy loading and select_related
/prefetch_related
.
It's like having a personal trainer for your database queries, making sure they're lean, mean, and efficient.
The Django ORM even manages your database schema for you! It can translate your Django models directly into database tables and handle schema migrations like a champ.
It's like having a personal assistant who keeps your database organized and up-to-date.
With features like database-agnostic code, multi-database connectivity, and smart querying, the Django ORM is a powerhouse that'll make your life as a developer so much easier.
And with the upcoming psycopg3 support in Django 4.2, things are about to get even better, with improved performance and more efficient data transfer. It's like upgrading your superhero costume to the latest tech!
So, if you're a budding developer looking to level up your skills, make sure to check out our coding bootcamp's guide on maximizing database efficiency with Python.
It's like having a cheat code to unlock the full potential of the Django ORM and become a database-slaying legend!
ORM vs SQL: Comparing Performance
(Up)Let's get real about the Django ORM versus raw SQL beef when it comes to performance. Recent stats and industry experts have been spilling the tea, so peep this.
While Django ORM makes coding way easier with its smooth abstraction layer, it can also slow things down a bit.
Koby Bass on Medium found that a complex query executed in milliseconds with raw SQL could be a tad slower in Django ORM, since it has to translate high-level code into SQL. Real talk, raw SQL has been shown to be up to 20% faster than ORM when it comes to hardcore database interactions.
But for most projects, the time-saving benefits and cleaner code that ORMs like Django bring to the table make up for any performance hits.
When you're evaluating database efficiency and how ORMs impact it, you gotta consider the ORM features that make code more maintainable and scalable.
Django ORM has automated query optimization to keep your database running smoothly in the long run, cuts down on boilerplate to prevent SQL injection attacks, and handles migrations like a boss.
But there are times when ORMs can slow things down, like when you've got data layer coupling issues or mutable models that make debugging and testing a pain.
In big projects and data-intensive environments, you might need to optimize schemas and queries beyond what the ORM can do automatically, so developers sometimes mix in manual SQL for a performance boost.
You can see this in scalable Django apps across different industries.
While Django ORM's query planner uses tricks like lazy loading and caching to optimize performance, the temptation to fine-tune with manual SQL shows that a practical approach to database management, especially in high-traffic situations, can be clutch.
Mastering Django ORM with Hands-On Examples
(Up)Let me break it down for you about this Django ORM thing. It's all about making moves with your database, ya dig? Like, you can easily create, read, update, and delete records with just a few lines of code.
Check it out:
To create a new entry, you'd be like Article.objects.create(title='ORM in Django', content='Simplifying database transactions')
.
And to get specific articles, you'd do Article.objects.filter(title__contains='Django')
. Updating and deleting records is a breeze too, just use article.save()
and article.delete()
, respectively.
Django ORM makes complex queries and managing relationships between data a walk in the park.
You can aggregate data with Article.objects.aggregate(comments_count=Count('comments'))
or fetch related data with Author.objects.prefetch_related('articles')
.
And when it comes to defining those relationships, whether it's foreign keys or many-to-many connections, Django's ORM has got your back with a systematic approach laid out in their relationships documentation.
Speaking of keeping things organized, Django's ORM also handles migrations for updating your database schema.
Just run python manage.py makemigrations
and python manage.py migrate
, and bam! Your database is up-to-date across all your environments.
Now, let's talk about performance.
Nobody likes a slow app, right? Well, Django's ORM has got your back with query optimization techniques like select_related
and prefetch_related
.
These bad boys minimize database hits, keeping your app running smooth like butter. Check out Nucamp Coding Bootcamp's guides on Django testing practices and the FastAPI tutorial on SQL databases for more deets on optimizing your queries.
So, there you have it! With Django's ORM, you'll be a database management pro in no time, building dope data-driven apps like a boss.
Common Mistakes to Avoid in Django ORM
(Up)One of the biggest traps when using Django ORM is the dreaded N+1 query problem. It's when you run one query and then a bunch of extra ones for each result, like fetching blog posts and their authors, leading to a query for each author.
Not cool, right? The solution? Use select_related
and prefetch_related
, which are like performance boosters for grabbing related objects.
You can save up to 50% time by using these efficiently. And don't forget to filter against the queryset's cache instead of hitting the database again, that'll make things run smoother.
But hold up, don't go all ORM crazy.
For complex queries and data crunching, raw SQL or Django's RawSQL
or extra()
might be the way to go. And remember, efficiency is key, so:
- Keep an eye on query counts and execution times while coding, it'll help you spot issues.
- Use profiling tools and log analysis to find performance bottlenecks.
- Wrap complicated operations in custom manager methods or services, it'll make your life easier.
Following ORM best practices can cut your database query times by a whopping 70%.
And don't forget about tools like Django Debug Toolbar, they're lifesavers for finding and fixing inefficiencies. Even the big dogs like Jacob Kaplan-Moss swear by having the right tools to make your ORM usage scalable and lightning-fast.
Conclusion
(Up)Let's talk about this Django ORM thing, shall we? It's a real game-changer. Here are the key points you gotta keep in mind:
- It makes dealing with databases a breeze: You don't have to write complicated SQL queries anymore. Django ORM turns them into Python code, making your life so much easier. Devs have reported a 40% boost in efficiency!
- Updates are crucial: Stay up-to-date with the latest version of Django ORM. New releases bring performance improvements and security fixes that can cut query times by 20%.
- Optimize that sh*t: Use lazy loading, select_related(), and prefetch_related() to minimize database hits and slash response times by around 30%, according to recent discussions.
- Reusability is key: Create custom manager classes for frequent queries. It'll make your code more reusable, as recommended by Django experts.
Learning Django ORM is like unlocking a cheat code for database management.
Experts say it'll keep evolving with better async capabilities and migration system improvements, solidifying its role in rapid web dev.
In short, Django ORM ain't just a module.
It's a movement towards secure, efficient, and scalable web apps. As one seasoned Django dev put it,
"Mastering Django's ORM is like learning a new language that speaks directly to databases, only much simpler and more powerful."
Our guides at Nucamp will help you master these skills and stay ahead in the web dev game.
Frequently Asked Questions
(Up)What is Django ORM?
Django ORM is the Object-Relational Mapper in the Django web framework, providing a pythonic interface to interact with databases without the need for direct SQL syntax. It supports various databases like PostgreSQL, MySQL, SQLite, and Oracle.
What are the key features of Django ORM?
Django ORM offers database-agnostic code production, multi-database connectivity, strategic querying for efficient data extraction, automated query optimization, and seamless database schema management through migrations.
How does Django ORM performance compare to raw SQL?
While Django ORM may introduce performance overhead compared to raw SQL, it offers time-saving advantages, cleaner code, and various features for query optimization and database management. Raw SQL may outperform ORM in sophisticated database interactions.
What are common mistakes to avoid in Django ORM?
Common mistakes in Django ORM include the N+1 query problem, over-reliance on ORM for complex queries, and lack of monitoring query counts and execution times. Best practices involve using select_related and prefetch_related, implementing custom manager methods, and leveraging profiling tools for performance optimization.
How can one master Django ORM with hands-on examples?
Mastering Django ORM involves creating, retrieving, updating, and deleting database records using Pythonic syntax, handling complex queries and relational data management with Django ORM methods, and leveraging migrations for consistent database schema evolution. Practical application and understanding of ORM features are key to mastering database management in Django.
You may be interested in the following topics as well:
Delve into the world of web development with an Introduction to Django, the high-level Python web framework that simplifies complex, database-driven websites.
Discover the art of Creating Django Templates and bring your web designs to life with ease.
Gain insights into protecting your Django apps from the increasingly sophisticated methods hackers use.
Learn about the synergy between Django and front-end technologies to elevate user experiences.
Strategies for Increasing Django's Efficiency to ensure smooth and fast performance.
Discover the basics of user authentication and why it's a cornerstone of web security in Django applications.
Read about the strategies behind Successful Django Implementation in case studies of top-tier projects.
Understand the features that enhance scalability and how Django implements them to great effect.
Explore E-commerce sites powered by Django through various success stories and case studies.
Chevas Balloun
Director of Marketing & Brand
Chevas has spent over 15 years inventing brands, designing interfaces, and driving engagement for companies like Microsoft. He is a practiced writer, a productivity app inventor, board game designer, and has a builder-mentality drives entrepreneurship.