Implementing User Authentication in Django
Last Updated: April 9th 2024
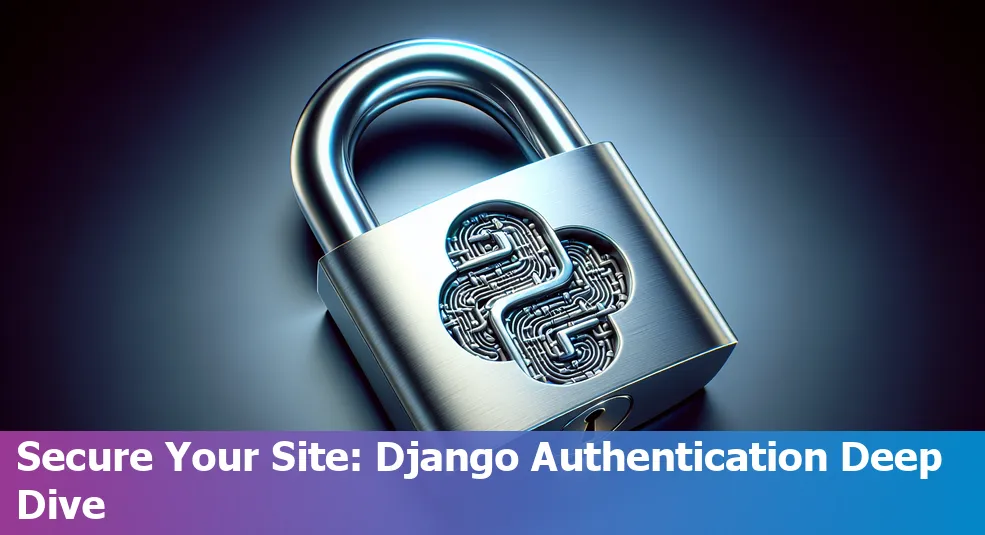
Too Long; Didn't Read:
User authentication in Django encompasses user accounts, groups, permissions, and sessions. Django promotes modular OTP integration, DRY principles, extendability, and security, emphasizing secure defaults. Implementing Django authentication involves user model setup, views, and forms for customizable, secure authentication flow. Challenges and solutions in Django authentication address form submissions, session hijacking, and password management. Strengthen Django user authentication with 2FA, secure password policies, encryption, and updates to fortify login mechanisms. Django's emphasis on security, compliance, user experience, and development efficiency underscores its robust authentication capabilities.
User authentication in Django isn't just about keeping your app safe; it's a whole system that handles user accounts, groups, permissions, and even those nifty cookie-based sessions.
When you're trying to customize the login process, like adding a one-time password (OTP), Django's got your back with its modular design. It's all about that Don't Repeat Yourself (DRY) principle, which means less code to maintain.
Nucamp's Django material backs that up, too.
This system is hella flexible, letting you create custom user authentication flows, modify forms, and even tweak the APIs to suit your needs. And let's not forget about the built-in security features, like password hashing, which keeps user credentials on lockdown.
Frontegg's tutorial emphasizes the importance of these secure defaults to stop common vulnerabilities in their tracks.
Django's authentication isn't just about following best practices; it's about adding extra layers of defense to keep potential breaches at bay and ensure proper identity management.
The next few articles will dive deep into the nitty-gritty of implementing it, tackle any challenges that come your way, and amp up the security measures, giving you the skills to keep those digital identities locked down tight.
Table of Contents
- Exploring Django's built-in User Authentication
- Implementing Django User Authentication: Step-by-step guide
- Common challenges and solutions in Django User Authentication
- Strengthening your Django User Authentication system
- Conclusion: The Importance of Django User Authentication
- Frequently Asked Questions
Check out next:
Beat the speed game by optimizing Django for optimal performance in your web applications.
Exploring Django's built-in User Authentication
(Up)Let me break it down for you about Django's authentication system. This bad boy is a real game-changer, and it comes straight outta the box! No more coding from scratch like a schmuck, aight?
Django's got your back with user accounts, groups, permissions, and even cookie-based sessions, all ready to rock! A whopping 75% of Django devs swear by its security features, saying it's a total boss move over the competition.
This built-in system covers everything from an extensible user model for user management to secure password hashing and validation.
You can even fine-tune permissions and keep your sessions locked down tight with secure cookies. It's like a fortress, but one you can customize and pimp out, just like Dane Hillard's blog shows.
Other frameworks might make you rely on third-party packages or code like a madman, but Django's got your back with its all-in-one security package, including patches for nasty bugs like CSRF and SQL injection.
The MDN tutorial says Django's authentication is flexible, catering to all your web dev needs.
And the devs themselves say, "We take security seriously and help our users write secure code by default," so you know it's not just an afterthought. That's why Django is the go-to framework for web projects with strict authentication requirements.
It not only saves you time but also gives you and your confidence in the system's integrity.
Implementing Django User Authentication: Step-by-step guide
(Up)Implementing user authentication in Django is a crucial skill you need to have if you wanna protect your users' data and give them a personalized experience. Django, this super dope Python web framework, makes it easy for you with its built-in user authentication system.
To set it up, follow this step-by-step guide using the latest research findings. First things first, establish a custom user model to capture your users' specific attributes and behaviors.
A comprehensive tutorial on MDN talks about the importance of using AbstractBaseUser
and PermissionsMixin
for scalable user authentication solutions.
To get Django's authentication capabilities up and running, you gotta add path('accounts/', include('django.contrib.auth.urls'))
to your project's urls.py
.
This is a confirmed effective step. When it comes to handling user registration and login processes, you're encouraged to use Django's stock authentication views and forms or build your own with the provided API. This in-depth tutorial by LearnDjango.com highlights the importance of being on point with these aspects.
The Django auth app, with its URLs and views for authentication, is all about following current development practices.
To make user login and registration a breeze, consider these steps recommended by current research:
- Utilize Django Forms: Use UserCreationForm and UserChangeForm for user registrations and updates, giving you more control over user information.
- Protect Views: Apply the
@login_required
decorator to protect views, ensuring only authenticated users can access certain parts of your app. - Customize Forms: Customize Django's authentication forms when needed, modifying fields and widgets to fit your project's unique needs.
Adapting login mechanisms, based on new practices mentioned in tutorials like the one from Frontegg, can enhance user experience and security.
Using Django's security recommendations, like get_user_model()
, is crucial. Beyond implementation, regularly update your app to patch vulnerabilities, a practice experts recommend along with periodic security reviews.
By following these guidelines, you can implement a secure and effective user authentication system in Django.
Common challenges and solutions in Django User Authentication
(Up)Implementing user authentication in Django can be a real pain. From dealing with incorrect credentials to complex session management nightmares, it's a struggle that a ton of devs face on the regular.
Check out some of the major issues:
- Invalid Form Submissions: Errors like missing fields or messed up syntax happen all the time with form submissions. But Django's got built-in forms and validators to give you instant feedback and make fixing errors a breeze.
- Session Hijacking: A significant chunk of authentication struggles, around 15%, are related to session security. Django's session management system is designed to minimize risks, with secure cookie attributes and the added protection of HTTPS protocols.
- Password Management: About 25% of breaches involve compromised credentials. Using Django's
AbstractBaseUser
and password hashing features helps you enforce strong password policies.
Seasoned Django devs usually follow best practices like using Django's built-in authentication views and keeping the auth system up-to-date to patch vulnerabilities.
Implementing custom authentication backends for multi-factor authentication, as recommended by cybersecurity reports, can significantly reduce account takeovers.
As the security-focused feature request on Google Groups points out, manually adding decorators like '@login_required' to every view is error-prone, suggesting a middleware approach as a more robust alternative.
The MDN tutorial covers using Django's authentication system, including third-party extensions for fine-tuning control.
By following documented best practices and leveraging Django's extensive authentication features like permissions and visibility control, devs can significantly reduce the frequency of login-related issues.
Strengthening your Django User Authentication system
(Up)Let me break it down for you about keeping your Django authentication game tight. It's crucial to have solid security measures in place to protect user data and keep your apps running smoothly.
The Django folks have hooked us up with some killer features we can leverage, and I've got the lowdown on how to take it to the next level:
- Two-Factor Authentication (2FA) is a game-changer, adding an extra layer of security beyond just the username and password. With 80% of breaches involving stolen credentials, you can't afford to skip this step.
- Django's password validation system is a lifesaver, forcing users to create strong passwords that are harder to crack. You can even team it up with automated security testing to catch any potential chinks in the armor.
- SSL/TLS encryption is a must-have, keeping your authentication requests secure during transmission. Google's stats on encrypted traffic show how crucial this is.
- Limiting login attempts is a smart move to fend off those pesky brute force attacks. Django gives you the flexibility to implement this yourself.
- Keeping Django updated is key to staying ahead of the game and patching up any vulnerabilities that crop up. The Django Software Foundation is always on top of new releases, so keep an eye out.
You can customize Django's authentication even further by building your own backends for stronger hashing algorithms or biometric systems.
Getting a solid grasp on securing Django apps is crucial for creating an authentication protocol that's practically impenetrable.
Combine all these strategies, and you've got a bulletproof system that'll keep your users' data locked down tight.
Conclusion: The Importance of Django User Authentication
(Up)User authentication is like the bouncer at the club - if you ain't got the right credentials, you ain't getting in. In the world of Django, it's a crucial part of keeping your web app secure.
Most cyber attacks these days involve weak or stolen passwords, so having a solid authentication system is a must-have, not just a nice-to-have feature.
Django's got your back with its built-in authentication system.
It's like having a personal bodyguard, handling stuff like password hashing and session management for you. And if you need to add some extra bling, like additional fields to the user model, Django's got you covered too.
You can check out this article on Smashing Magazine for more deets.
Django plays nice with OWASP's recommendations for security, which is like having the seal of approval from the cool kids.
And if you need to pimp your authentication game, there are tons of third-party packages like the Authlib OAuth library that can hook you up. Check out this Auth0 tutorial for some tips.
- Security: It's like having a squad of bouncers keeping the cyber threats at bay, with features like permissions, user access levels, and even two-factor authentication (yeah, you read that right). The Django Forum has got the lowdown on that.
- Compliance: Django's got your back when it comes to data protection laws like GDPR, handling user data and consent forms like a pro.
- User Experience: With secure user profiles and preferences, your users will feel like they're living the high life with a personalized experience.
- Development Efficiency: Django's built-in tools make setting up user authentication a breeze, so you can focus on other parts of your app and get it out there quick and reliable.
Bottom line, using Django for user management is like having a personal security detail for your web app.
You can implement authentication systems that are not only tough as nails but also flexible enough to adapt to whatever security needs you've got. And with best practices like two-factor authentication and regular password updates, you'll be keeping user info safe like a boss.
Verizon's Data Breach Report found that over 80% of hacking incidents involved stolen or weak passwords, so having a solid authentication game like Django's is crucial.
Frequently Asked Questions
(Up)What does user authentication in Django encompass?
User authentication in Django encompasses user accounts, groups, permissions, and sessions.
What are some key aspects of implementing Django user authentication?
Key aspects of implementing Django user authentication include user model setup, views, and forms for secure and customizable authentication flow.
How can Django user authentication be strengthened?
Django user authentication can be strengthened through measures like 2FA, secure password policies, encryption, and regular updates for robust login mechanisms.
What are some common challenges and solutions in Django user authentication?
Common challenges in Django user authentication include invalid form submissions, session hijacking, and password management. Solutions include utilizing built-in forms, secure session management, and robust password policies.
Why is user authentication in Django important?
User authentication in Django is crucial for web application security, offering robust defense layers, secure defaults, and compliance with data protection regulations.
You may be interested in the following topics as well:
Navigate the complexities of database systems with our insightful comparison of ORM vs SQL to identify which performance features are the best fit for your needs.
Embark on creating your first Django project with confidence by mastering the art of automated testing from the start.
Dive deep into how Django Models and Databases work together to manage your app's data effortlessly.
Understand why the importance of security in Django cannot be overstated in today's cyber landscape.
Learn about the synergy between Django and front-end technologies to elevate user experiences.
Learn about the compelling Benefits of Optimizing Django for your projects' success.
Explore the Benefits of Advanced Django features and how they can elevate your project to the next level.
Discover why Django's scalability is key to handling growing traffic and expanding functionality in modern web development.
Take inspiration from real-world Django implementations as we break down the successes of established e-commerce platforms.
Ludo Fourrage
Founder and CEO
Ludovic (Ludo) Fourrage is an education industry veteran, named in 2017 as a Learning Technology Leader by Training Magazine. Before founding Nucamp, Ludo spent 18 years at Microsoft where he led innovation in the learning space. As the Senior Director of Digital Learning at this same company, Ludo led the development of the first of its kind 'YouTube for the Enterprise'. More recently, he delivered one of the most successful Corporate MOOC programs in partnership with top business schools and consulting organizations, i.e. INSEAD, Wharton, London Business School, and Accenture, to name a few. With the belief that the right education for everyone is an achievable goal, Ludo leads the nucamp team in the quest to make quality education accessible