Getting Started with Django for Web Development
Last Updated: April 9th 2024
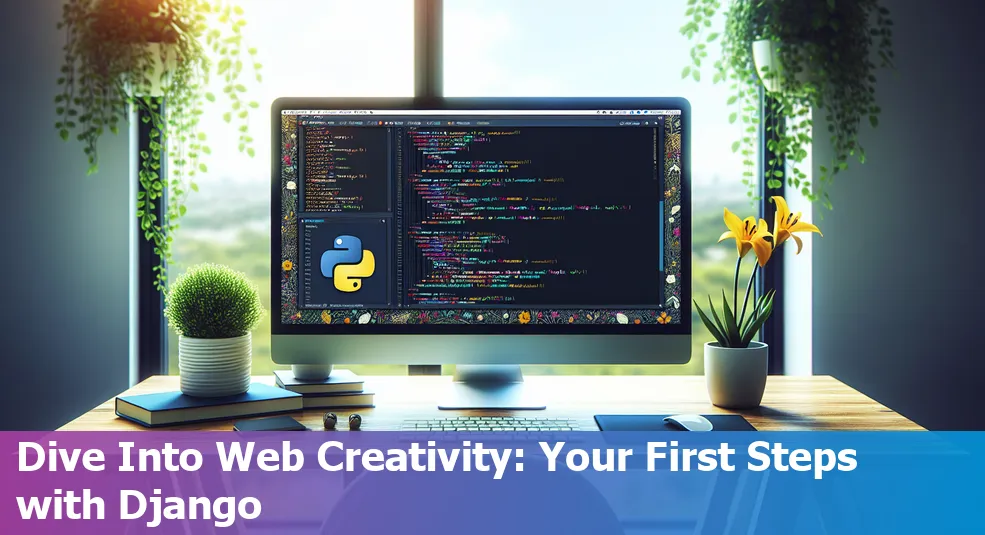
Too Long; Didn't Read:
Django is a Python web framework for efficient web development with built-in solutions. Used by 12,000+ websites globally, it simplifies database interactions and is preferred by developers over Flask. Django streamlines web app creation with rapid development and ORM, enhancing scalability and security for modern projects.
Django is this dope Python framework that makes building websites a total breeze. It's got a "batteries-included" vibe, meaning it comes packed with built-in solutions for all sorts of web shenanigans.
That's why big dogs like Instagram and The Washington Post are rocking it – this bad boy can handle some serious traffic.
With its massive package index, Django lets you whip up secure and scalable sites like it's nobody's business.
Over 12,000 websites worldwide are using it! One of Django's standout features is the Object-Relational Mapper (ORM), which makes working with databases a walk in the park.
Python devs seem to be digging Django too – a JetBrains survey showed they prefer it over Flask.
And it's not hard to see why – Django first hit the scene in 2005 and totally changed the game for building complex web apps.
Their motto, "The web framework for perfectionists with deadlines," pretty much sums it up.
It's all about getting stuff done quickly and efficiently. You can check out this blog for some sick insights on advanced Django features for professional projects.
Table of Contents
- How To Install Django: A Step-By-Step Process
- Your First Web App with Django: The Basics
- Exploring Django Models and Databases
- Building Views and Templates with Django
- Deploying Your Django Application
- Frequently Asked Questions
Check out next:
Begin your journey into the world of web programming by mastering the Django setup essentials in our comprehensive guide.
How To Install Django: A Step-By-Step Process
(Up)Installing Django on your Windows machine isn't a big deal, but you have to make sure Python's already on your system first. It's like the backbone for Django's robust and secure web framework.
This dude named Stanley Ulili has a detailed guide on how to do it.
First up, open PowerShell and check if Python's installed by typing python -V
. Then, upgrade Python's package installer, pip, with python -m pip install --upgrade pip
.
After that, create a project folder and set up a virtual environment – it's like a separate playground for your project dependencies. Activate it with venv\Scripts\activate
.
Now you can install Django itself using pip install django
, and start a new project with django-admin startproject test_project
. Finally, run the Django development server to make sure everything's good.
For the full rundown, check out the official Django docs – they have beginner-friendly instructions and troubleshooting tips.
Virtual environments are key. They let you keep your project dependencies separate, as the Vegastack guide explains.
They also highlight Django's rapid development and tight security, making it a solid choice for both newbies and seasoned coders.
If you run into issues like 'pip not found' or 'Python not recognized', double-check that Python and pip are properly installed and added to your system's PATH variable.
Follow the steps carefully, and you'll be cruising through Django's web dev powers in no time.
Your First Web App with Django: The Basics
(Up)You wanna build a sick web app with Django? Let me break it down for ya. Django's all about keeping things organized and avoiding repetitive code, and you gotta start by understanding how it's structured.
It's like a well-oiled machine!
First things first, you need to set up your Django environment. Install that bad boy using pip, Python's package installer.
Once that's done, create and run a minimal Django app. You'll use the django-admin startproject
command to generate a new project, which is the foundation for your web app.
This command creates a directory with essential files like manage.py
(for interacting with the project from the command line) and settings.py
(for configuring your app).
Now, you gotta dive into the Django project structure and understand elements like urls.py
and wsgi.py
.
These bad boys help you configure your app and manage how it runs. According to Mozilla's MDN web docs, around 73% of Django devs modify URL patterns and database settings right off the bat.
Defining URL patterns is crucial, as it ensures HTTP requests get routed to the right Django views, which can seriously boost user retention.
Once you've got the structure down, it's time to create an app within your project using python manage.py startapp
.
This allows you to divide your app's functionality into separate modules, keeping things modular and organized, just how Django likes it. As one of Django's original devs, Thomas Martinez, puts it, "The beauty of Django lies in its promotion of modular design." Within each app, you'll have models, views, and templates – the building blocks of the MVC (Model-View-Controller) architecture, which can seriously boost your productivity.
Mastering how to link these components through models and URL patterns will set you up for a scalable and robust web app that'll blow your buddies' minds!
Exploring Django Models and Databases
(Up)Let me break it down for you about this Django ORM thing. It's like the homie that handles all the database interactions for you, so you don't gotta worry about writing those complex SQL queries.
It's a game-changer, for real.
- Define your data models: Think of it like this - each model you create is like a blueprint for a table in your database. You define the attributes (columns) in your Python class, and boom, the ORM takes care of the rest. For example,
class Blog(models.Model): title = models.CharField(max_length=100)
translates to a blog table with a title column. Then you can create, read, update, and delete entries using the ORM's built-in methods. Easy peasy! - Database Migrations: Whenever you make changes to your models, you gotta run
python manage.py makemigrations
followed bypython manage.py migrate
. This tells Django to update your database schema accordingly. It's like version control for your database, keeping everything synced and organized. - Understanding relationships: The ORM lets you define different types of relationships between your models, like 'ForeignKey' for one-to-many, 'ManyToManyField' for many-to-many, and 'OneToOneField' for one-to-one. It's like setting up connections between your data, but without all the hassle of writing complex SQL.
The migrations system is a real lifesaver.
It's like having a backup of your database schemas, so you can revert to older versions or collaborate with your squad without any conflicts. And the ORM's ability to abstract the database interactions makes maintaining and scaling your code a breeze.
"Using the Django ORM, you translate complex join operations into Python code, making database interactions more intuitive and developer-friendly,"
says this one Django tutorial.
By following best practices, like avoiding direct data manipulation in views and minimizing database hits, you can keep your ORM efficient and secure. With Django's ORM, you can kiss those database design and manipulation headaches goodbye, especially when you're working on Rapid Application Development (RAD) projects.
It's a real game-changer!
Building Views and Templates with Django
(Up)At the core of Django's architecture, you've got the dynamic duo of views and templates. Views are like the conductors, orchestrating the database model and the template, serving up the app's data and logic to the user.
On the other hand, templates are like the canvas, providing placeholders for dynamic content that the views can paint on.
Check out this tutorial on Django views for a deeper dive into how views fetch data and deliver it through templates.
It's a big deal, considering over 70% of web apps leverage server-side data processing, according to MDN web docs. That's why views play a crucial role in web dev.
But templates ain't no slouches either.
With the Django Template Language (DTL), they become interactive exhibits, allowing HTML to flex with dynamic content. This in-depth guide breaks down how templates are text files, mainly HTML, and explains the DTL's philosophies, like separating logic from presentation and avoiding redundancy.
These templates can extend a base using {% extends 'base.html' %}, a feature that aligns with Django's Don't Repeat Yourself (DRY) motto and streamlines the web dev workflow, as highlighted in MDN's Django Tutorial Part 6.
Django's architectural prowess allows for a clean separation of the presentation layer from the application's logic, which is crucial for the flexibility and scalability required in modern web dev practices.
This sentiment is shared by 43% of devs in a recent Stack Overflow survey. This clear division between views and templates is a cornerstone for refining the dev process and leveling up the quality of web apps built with Django, weaving a tale of efficiency and sophistication for devs worldwide.
Deploying Your Django Application
(Up)Deploying your Django app is a big deal, it's like taking your code from the lab to the real world. But before you do that, you gotta follow some crucial steps.
First, turn off the DEBUG mode, that's just for testing and can expose sensitive info. Next, set up a secure HTTPS connection because no one wants their data to be out in the open.
And don't forget to manage your secret keys through environment variables, you don't want them floating around in your code.
This dude named Randall Degges wrote an article called "Deploying Django" that talks about the whole process of going from development to deployment, and how devs spend a ton of time just getting everything set up properly.
Now, for the actual deployment, AWS is a big player with around 32% of devs using it because it's super scalable.
They offer this service called 'ElastiC Beanstalk' that makes it easy to work with all the different AWS offerings and deploy your app without too much hassle.
On the other hand, if you're just starting out or working on a smaller project, Heroku might be a better fit. They have this simple command 'git push heroku master' that basically does all the work for you.
Heroku's tutorials are pretty straightforward too, which is why a lot of people dig it.
But here's the thing, moving from development to a live environment can get tricky.
That's why people on the Django Community Forum recommend using staging environments and setting up CI/CD pipelines to avoid any major issues.
Another cool tool is Docker, which packages your app into containers, so you don't have to deal with that "it works on my machine" nonsense. Around 35% of organizations use it because it makes deployment way smoother.
At the end of the day, there are tons of options for deploying your Django project, and you can pick whatever fits your project's size and setup.
The Nucamp guides on building scalable Django apps and automated testing in DevOps can give you a solid overview of what works best.
Frequently Asked Questions
(Up)What is Django?
Django is a high-level Python web framework that simplifies website development, promising efficiency and a pragmatic approach. It stands out with its 'batteries-included' philosophy, presenting built-in solutions for numerous web tasks.
How many websites globally use Django?
Django is used by over 12,000 known websites worldwide, indicating its popularity and ability to handle the robust demands of modern web applications.
Why is Django preferred over Flask by developers?
A JetBrains survey noted a preference for Django over Flask among Python developers due to Django's rapid development, ORM features, and strong security measures, making it a favored choice for web development.
How does Django simplify database interactions?
Django simplifies database interactions through its Object-Relational Mapper (ORM), which allows developers to work with databases using Python code instead of SQL queries, enhancing efficiency and productivity in development workflows.
You may be interested in the following topics as well:
Navigate the complexities of database systems with our insightful comparison of ORM vs SQL to identify which performance features are the best fit for your needs.
Unveil why embracing automated testing in your Django projects is a transformative step towards efficient development.
Adopting best practices for Django security could mean the difference between a safe application and a compromised system.
Discover how Django's flexible architecture makes it a formidable backend choice for web developers.
Learn about the compelling Benefits of Optimizing Django for your projects' success.
Uncover common user authentication challenges in Django and the best practices to overcome them.
Get an in-depth analysis of the Django Advanced Features that make it a powerhouse for professional development.
Discover why Django's scalability is key to handling growing traffic and expanding functionality in modern web development.
Delve into the Pros and cons of Django, weighing the benefits against the potential drawbacks for your online storefront.
Chevas Balloun
Director of Marketing & Brand
Chevas has spent over 15 years inventing brands, designing interfaces, and driving engagement for companies like Microsoft. He is a practiced writer, a productivity app inventor, board game designer, and has a builder-mentality drives entrepreneurship.