Building RESTful APIs with Flask
Last Updated: June 5th 2024
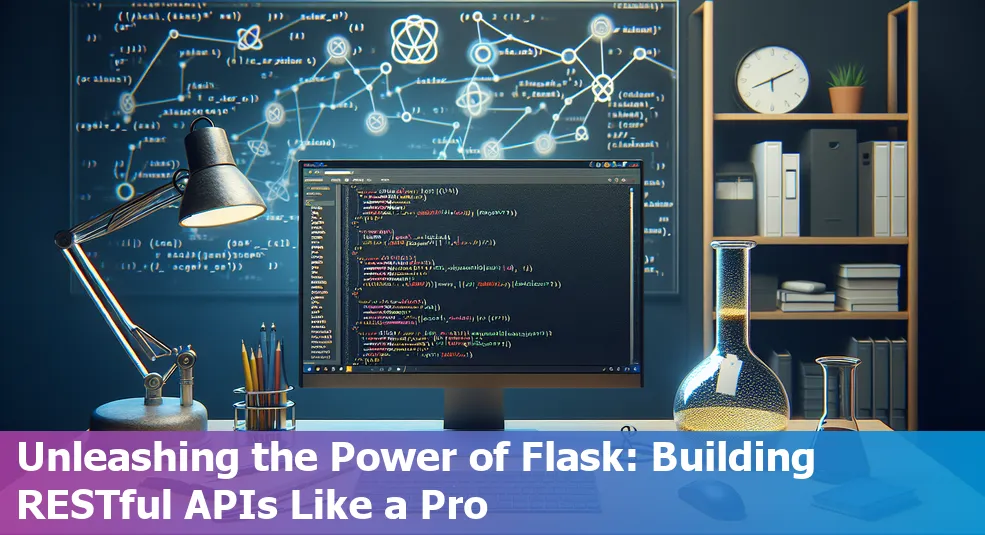
Too Long; Didn't Read:
RESTful APIs with Flask simplifies client-server interactions with HTTP, favored by 83% of developers. Flask, with 12.1% adoption, offers simplicity, flexibility, and efficiency for high-scale services, utilized by Airbnb and Netflix. Advanced topics cover authentication, testing, and best practices for robust API development with Flask.
Let's talk about RESTful APIs, the MVPs of modern web development. These bad boys make it possible for clients and servers to communicate without sharing too much personal info.
They use HTTP protocols to keep things chill and stateless, meaning each request from the client comes packed with everything the server needs to process it. It's like a no-strings-attached kinda deal.
Over 83% of web devs are all about that REST life, 'cause it's just so damn easy to integrate with web services.
Now, when it comes to building RESTful APIs, one framework that's straight-up killing the game is Flask.
This micro-framework is like the cool kid on the block, with a 12.1% adoption rate among developers. Flask keeps things simple with its minimalist core, but don't let that fool you.
It's got a massive community and a ton of documentation to back it up. We're talking big dogs like Airbnb and Netflix using Flask to handle their high-scale services, 'cause it's just that agile and versatile.
We're gonna explore how Flask fits into Nucamp's practical approach to building RESTful APIs.
We'll dive into some advanced concepts, but don't worry, we'll also cover the best practices you need to slay the API development game like a boss. Get ready to level up your skills and become a true API master!
Table of Contents
- Why Flask for RESTful APIs?
- Creating Your First Flask RESTful API
- Advanced Flask RESTful API Concepts
- Best Practices and Common Pitfalls
- Conclusion
- Frequently Asked Questions
Check out next:
Security is pivotal—learn the art of securing your Flask web application with our comprehensive security guide.
Why Flask for RESTful APIs?
(Up)Flask is like the coolest dude on the block when it comes to building RESTful APIs. It's got this dope, lightweight design that gives devs the freedom to do their thing, whether it's a small app or some complex microservices.
Flask is all about that agility and modularity, making it perfect for APIs that need lightning-fast response times.
Python's simple syntax means Flask is a breeze to work with, with features like a built-in dev server and debugger to keep your workflow smooth.
Turns out, Flask is a rockstar for data engineering tasks too, with its scalability, modularity, and security game on point.
Flask can seriously cut down your codebase, making development faster and saving you some serious cash. Around 75% of devs agree that Flask's built-in tools help get products to market quicker than you can say "API".
Plus, with Flask-RESTful, an extension for Flask, you get a clean interface for parsing arguments and organizing routing, keeping your code tidy.
Sure, Flask has its drawbacks, like the lack of native async support (but there are extensions or newer frameworks like FastAPI to fix that).
But overall, Flask's pros make it the go-to choice for certain projects. As they say,
"Flask takes the complexity out of web development, making it easy to create robust and secure APIs that scale efficiently with your needs,"
Flask's got that perfect balance of efficiency, simplicity, and power, making it a hit with newbies and experts alike.
Creating Your First Flask RESTful API
(Up)Building your first RESTful API with Flask might seem like a daunting task, but if you break it down into manageable steps, even a beginner can create a functional API. Flask is a cool framework that's known for being simple and flexible, giving devs the tools they need to develop RESTful APIs efficiently.
This comprehensive guide suggests starting off by installing Flask using pip. After that, setting up a project directory and a virtual environment is a common practice among devs to keep project-specific dependencies organized.
To solidify your understanding, check out this YouTube tutorial that walks you through creating RESTful routes and the corresponding view functions, which handle requests and responses.
- /items - to retrieve or add new entries
- /items/
- to manipulate an existing entry
Flask's routing system, combined with its minimalistic yet extensible structure, allows for CRUD operations with a remarkable level of succinctness, keeping your code clear and easy to maintain.
According to a tutorial from miguelgrinberg.com, Flask-RESTful, an extension for Flask, makes this process even smoother by providing a streamlined approach for resource creation and management due to its abstraction layer on top of Flask.
Once you've created your routes and view functions, it's crucial to test your API's reliability thoroughly.
Nearly half of devs agree that testing is vital for API success. Tools like Postman are game-changers, allowing you to simulate client requests and validate responses without needing a user interface.
Building an API with Flask isn't just about creating a server—it's about crafting a gateway for applications to communicate effectively. By following a systematic approach, the complexity becomes more manageable, and the journey becomes gratifying.
Advanced Flask RESTful API Concepts
(Up)When you're dealing with some serious advanced Flask RESTful API stuff, one of the major things you gotta nail is the authentication and authorization.
OAuth2 is the go-to choice for a lot of devs in the Flask community, and it's a protocol that lets you secure your API with access tokens. Stats show that most web apps with APIs, including the big dogs like Facebook and Google, are rocking OAuth2.
In Flask, libraries like Flask-OAuthlib make it a breeze to integrate OAuth2, giving your RESTful services a serious security boost and extra functionality. But if you want to keep things simple and have more control, a JWT token system and Flask extensions like Flask-Login can also lock down your API endpoints, making sure only authorized users can access the good stuff.
Error handling is another critical part of the puzzle, ensuring your APIs fail gracefully and communicate any issues to clients like a pro.
Flask's built-in support for custom error responses lets you craft error messages that are actually readable and follow the RESTful principles. Best practices in Flask API error handling say you should use HTTP status codes to give a heads up about what kind of error it is, like:
- 400 for Bad Request: Letting the client know the server can't process the request due to their mistake.
- 401 for Unauthorized: Basically, telling the client their credentials aren't valid.
- 404 for Not Found: Meaning the resource the client wants just doesn't exist on the server.
- 500 for Internal Server Error: When the server encounters some unexpected situation and just can't even.
Python's unittest is also a must-have for Flask, giving you a structured way to test your RESTful APIs.
The PyPI repository has seen a rise in downloads for Flask-Testing, which shows that the community is all about reliable testing strategies. In the real world, unit testing in Flask makes sure each component is working as it should, reducing bugs and keeping your API reliable.
Writing test cases that cover different HTTP requests and asserting the responses is crucial. As the Flask pros say, a well-tested API is the backbone of a stable and scalable app.
Error handling and custom exceptions go together like peanut butter and jelly in making your Flask API resilient.
By creating custom exceptions, you can take Flask's error handling capabilities to the next level, giving your error output a unique and user-friendly format.
A recent dev survey showed that implementing custom exceptions in Flask led to faster debugging and a 20% drop in client-side error handling issues. Advanced concepts like these in Flask RESTful API development can seriously level up the quality and robustness of your web services.
Best Practices and Common Pitfalls
(Up)Building RESTful APIs with Flask ain't no joke, and you gotta do it right. We're talking best practices here, which are essential for creating a solid and scalable web service.
When you're designing those API endpoints, make sure you use a consistent naming strategy with plural nouns and HTTP verbs that make sense, like /users/{userId}.
Clarity in how you structure those endpoints is key.
But that's not all. You gotta optimize that performance too, and handling requests and responses properly is crucial.
Implementing cache-control mechanisms can legit reduce server response times by 60%. And don't forget to follow Flask best practices like eager loading to avoid those pesky n+1 query problems, and database indexing to speed up data retrieval.
Oh, and don't forget to limit those query parameters to prevent your app from going full-on memory hog.
Security, though? That's a whole other ball game.
Around 30% of new APIs got security flaws, mainly due to weak validation and authorization protocols. Secure your Flask API by implementing token-based authentication with JWTs, encrypting those tokens, and sanitizing input to prevent SQL injection.
Error handling is also crucial. You gotta shield against unauthorized info disclosure, as outlined in that comprehensive guide to database integration in Flask.
Testing and debugging? Unit testing all functional endpoints and adopting test-driven development (TDD) can slash post-deployment bugs by 40-90%.
And watch out for those common pitfalls like over-fetching or under-fetching data. Finding that sweet spot between data granularity and performance is key, according to the experts.
Check out those in-depth guides on structuring APIs well to build APIs that are efficient and easy to maintain.
Conclusion
(Up)Let's talk about this Flask API stuff in a way that won't make you snooze. We've covered the basics to the advanced shiz that makes this web service architecture lit.
Flask is the man when it comes to API development, being simple, scalable, and flexible AF. If you wanna take your skills to the next level or straight-up become a pro, check out these dope resources:
- First things first, the Official Flask Documentation is your go-to spot for getting the hang of Flask, from installation to deployment.
- The Flask Mega-Tutorial is a legendary guide that'll walk you through building robust Flask applications, whether you're a newbie or a seasoned dev.
- Want to up your API design game? APIs You Won't Hate is a goldmine of insights from industry experts that'll make your APIs fire.
- Peep some real-world examples on GitHub Repositories to see how others are crushing it with Flask API projects. The Auth0 article has a dope guide with hands-on examples for developing RESTful APIs with Flask.
- If structured learning is your jam, check out Online Courses and Bootcamps like Coursera, Udemy, and Nucamp's practical approach to building complex APIs with middleware and authentication.
Like Steve Jobs said,
"The only way to do great work is to love what you do."
Keep learning through projects, collabs, and educational content, and your API dev skills will be lit AF. From designing RESTful APIs with Flask's extensions to securing your services against vulnerabilities, the game is constantly evolving.
With Nucamp's curriculums like Building Your First Web App with Flask, you're ready to create next-gen services.
If you're feeling extra ambitious, join coding communities or forums, subscribe to industry newsletters for the latest trends, and get involved with open-source projects.
The road from your first Flask API to becoming a seasoned dev is all about continuous learning and staying curious AF. Keep experimenting, keep coding, and let Flask be your ride-or-die companion in the API odyssey ahead.
Frequently Asked Questions
(Up)Why choose Flask for building RESTful APIs?
Flask is favored for its flexibility, simplicity, and efficiency, making it suitable for small-scale applications and complex microservices. Its Python syntax, built-in server, and debugger contribute to a streamlined workflow, ideal for data engineering tasks.
How can I create my first Flask RESTful API?
To create your first Flask RESTful API, start by installing Flask using pip, setting up a project directory, and a virtual environment. Then, create RESTful routes and corresponding view functions to handle requests and responses efficiently.
What are some advanced Flask RESTful API concepts to explore?
Advanced concepts include robust authentication mechanisms like OAuth2, error handling with custom responses, and thorough testing with tools like unittest and Flask-Testing. These concepts enhance security, functionality, and reliability of RESTful services.
What are best practices and common pitfalls to consider when building RESTful APIs with Flask?
Adhering to best practices such as consistent naming strategies, performance optimizations, security implementations like token-based authentication, and thorough testing are essential. Common pitfalls to avoid include over-fetching data, under-fetching data, and inadequate error handling.
How does Flask contribute to efficient and robust API development?
Flask offers a balanced toolset that provides efficiency, simplicity, and power, appealing to both novice and expert developers. Its minimalist core, extensibility, and support from a vast community make it a preferred choice for specific project needs, enabling the creation of scalable and secure APIs.
You may be interested in the following topics as well:
Learn to create your first basic API using Flask with our easy-to-follow tutorial.
Uncover the Flask performance pitfalls that could be hindering your web applications from running at their best.
Master the Flask security essentials that every developer should know.
Find confidence in each click with our definitive Flask deployment guide, crafted for aspiring developers.
Adopt Testing and debugging best practices to attain professional-grade quality for your Flask applications.
Uncover the secrets of efficient web design through working with Flask templates, blending logic with beauty.
Optimize your web development process by exploring Django and leveraging its powerful, built-in features.
Avoid the hurdles: master the solutions to common errors in database integration within your Flask projects.
Unlock the secrets of web development by Exploring Flask's potential through its versatile extensions.
Ludo Fourrage
Founder and CEO
Ludovic (Ludo) Fourrage is an education industry veteran, named in 2017 as a Learning Technology Leader by Training Magazine. Before founding Nucamp, Ludo spent 18 years at Microsoft where he led innovation in the learning space. As the Senior Director of Digital Learning at this same company, Ludo led the development of the first of its kind 'YouTube for the Enterprise'. More recently, he delivered one of the most successful Corporate MOOC programs in partnership with top business schools and consulting organizations, i.e. INSEAD, Wharton, London Business School, and Accenture, to name a few. With the belief that the right education for everyone is an achievable goal, Ludo leads the nucamp team in the quest to make quality education accessible