Database Integration in Flask: A How-To Guide
Last Updated: April 9th 2024
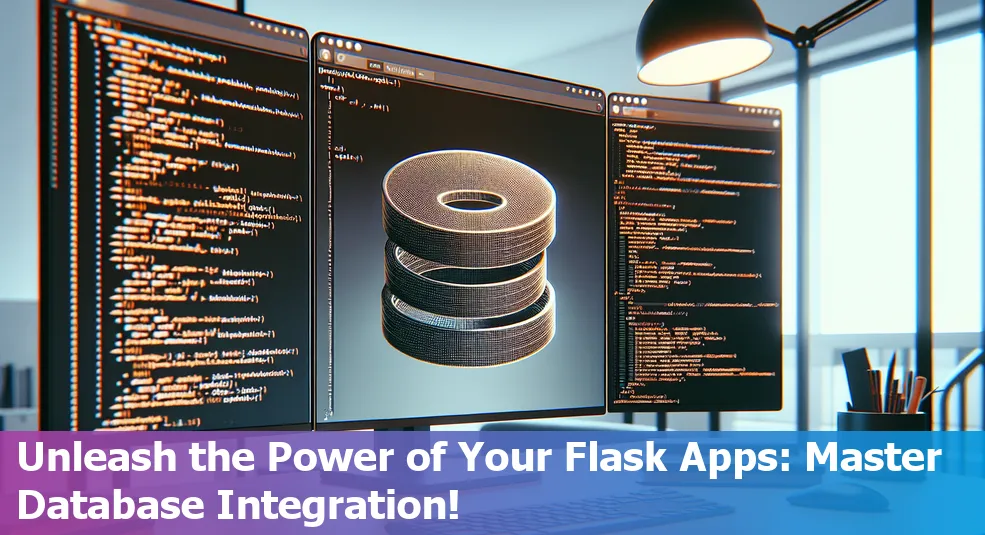
Too Long; Didn't Read:
Flask empowers seamless database integration with various DBMS like SQLite, PostgreSQL, MySQL, and NoSQL options such as MongoDB, boosting app efficiency, scalability, and security. Extensions like flask-sqlalchemy simplify data interactions, while best practices ensure smooth implementation in Flask applications for optimal performance.
Flask, that badass Python framework, is all about flexibility and control when it comes to web apps. But what really makes it dope is its ability to seamlessly integrate with databases.
Databases are like the backbone for storing and managing data, which is crucial for making your web app dynamic and interactive.
With Flask, you can easily hook up to various Database Management Systems (DBMS) like SQLite, PostgreSQL, MySQL, or even NoSQL monsters like MongoDB. This integration means your Flask app can scale like a beast and handle increasing amounts of data and user traffic without breaking a sweat.
By using extensions like flask-sqlalchemy, you can add extra functionality like a database abstraction layer, making it super easy to work with data.
This just amplifies Flask's versatility, allowing it to adapt to different project needs.
Integrating databases with Flask brings some serious benefits, such as:
- Boosting your app's efficiency with streamlined data operations.
- Expanding its capability to handle growing demands for data and user traffic.
- Advancing data protection with robust database protocols.
- Selecting the perfect database system to meet your project's specific goals.
In the upcoming sections, we'll dive deeper into the nitty-gritty of database integration, practical tips for smooth implementation, common challenges you might face, and best practices to unleash the full potential of databases in your Flask apps.
If you want to level up your Python and database game, check out Nucamp's comprehensive guide.
And for those who want to master the art of database-driven web apps, make sure to explore the deeper aspects of Flask routes in our specialized coverage.
Table of Contents
- Understanding Database Integration
- How Flask Interacts with Databases
- Steps to Database Integration in Flask
- Common Pitfalls and Their Solutions
- Conclusion and Best Practices
- Frequently Asked Questions
Check out next:
Dive into the world of mastering web development with our latest comprehensive guide on the Flask framework.
Understanding Database Integration
(Up)Database integration in Flask is like the foundation of your entire web app. Database integration means connecting your Flask app with a database to store, manipulate, and retrieve data.
Most devs use databases like MySQL, PostgreSQL, and MongoDB to keep their data safe and manage it efficiently. The type of database you choose and how you integrate it can seriously impact how your app functions and how smooth the user experience is.
Stack Overflow's survey shows that database integrations are super common, so it's a must-have for any serious web app.
- Persistent Data Storage: Databases are way more reliable than temporary storage, keeping your data secure even if the server restarts or the app reloads.
- Efficient Data Management: With database management systems (DBMS), devs can take advantage of SQL and other tools to handle data operations like a pro.
- Scalability: Your Flask app's growth potential depends on how scalable your database is, which is crucial in today's data-saturated world.
- Improved Performance: Integrating your database the right way can optimize server loads and speed up response times.
- Security: A solid database guards your app against vulnerabilities.
"Integrating databases seamlessly in Flask doesn't just future-proof your app, but it also makes the user experience way better," says Joseph Martinez, a top industry expert.
Whether you're a startup or a massive enterprise, this foundational step is key for data-driven decision-making and building robust web apps.
With over 90% of current global data emerging recently, skipping database integration can make your Flask app obsolete in this data-centric era. Integrating a database with Flask isn't just recommended; it's a game-changer for technological success.
How Flask Interacts with Databases
(Up)Check it out! Flask is this dope framework that packs a mean punch for web apps. It's like a lean, mean, coding machine that really shines when it comes to database integration.
That's where Flask-SQLAlchemy steps in – it's like your personal translator between Python and SQL databases. With this tool, you can hook up to all sorts of databases like SQLite, PostgreSQL, and MySQL, using a slick syntax that's almost like SQL, but not quite.
- SQLite Integration: Perfect for small-time projects, SQLite is an embedded database that's easy to set up.
- Postgres Power: If you're looking for some serious muscle, the PostgreSQL extension has got your back with a robust and scalable database solution.
- MySQL Madness: MySQL is a free and open-source database that's a popular choice for web apps.
- Oracle Optimization: Oracle brings high-availability and enterprise-level features to the table.
- Microsoft Mania: Microsoft SQL Server is the go-to database for .NET environments.
Flask-Migrate is like your personal database migration wizard.
Powered by Alembic, it automates the process of managing schema changes, keeping your database up-to-date with your code. It supports multiple databases with the Flask-SQLAlchemy "binds" feature, so you can switch things up whenever you need to.
And for all you NoSQL fanatics out there, Flask-MongoEngine has got your back with MongoDB integration, even though it's not a default configuration.
- Flask-SQLAlchemy: The tool that makes SQL database integration a breeze for Flask apps.
- Flask-MongoEngine: The extension that brings the power of MongoDB to your Flask app.
- Flask-Redis: An optional add-on for caching and session management, giving your database features an extra boost.
As your app grows and becomes more complex, Flask's database-agnostic engine allows you to mix and match the best technologies for your needs.
Just make sure to follow best practices like connection pooling, query optimization, and the Twelve-Factor app methodology for managing database credentials. As
Armin Ronacher put it, Flask doesn't force any decisions on you, but with its extensions, it makes database interactions feel almost intuitive.
Steps to Database Integration in Flask
(Up)Integrating a database into your Flask app is a game-changer, and it's not as complicated as it may seem. The key is to choose the right database, like MongoDB Atlas for NoSQL or PostgreSQL if you want something robust.
Then, you gotta use SQLAlchemy for its Object-Relational Mapping (ORM) feature, which lets you interact with databases through Python classes in Flask. It's a whole journey, but here's how you do it:
- Start by installing Flask-SQLAlchemy using
pip
. This gives you the ORM capabilities to connect Python with databases like a boss. Install ORM capabilities to bridge Python with databases. - In your Flask app configs, set up your database URI correctly. This includes choosing the right database driver and specifying the connection details. Precisely establish database URI to ensure correct connections.
- Structure your database models by subclassing
db.Model
. This way, your database schema is reflected in your Flask app, making everything clear as day. Structure database models to mirror your schema. - Implement Flask-Migrate to handle database changes like a pro. Start by running
flask db init
, then generate new migrations withflask db migrate -m "initial migration"
, and apply them withflask db upgrade
. Implement Flask-Migrate for smooth database alterations. - Manage your database operations within your Flask routes or business logic layers. Use ORM methods to create, read, update, or delete records, following best practices like data normalization, foreign key relationships, and indexing for search optimization. Orchestrate database operations using ORM for CRUD functionality.
To really level up your app, follow best practices in designing database schemas.
Normalizing your data reduces redundancy and boosts performance, as pointed out by the International Journal of Database Management Systems. Plus, a case study from Database Journal shows how crucial meticulous data migration is for keeping everything running smoothly.
Flask-Migrate's incremental migration method ensures a smooth transition for database changes, so your app stays lit without interruptions.
Common Pitfalls and Their Solutions
(Up)When you're coding with Flask and trying to hook it up with a database, things can get pretty messy if you're not careful. One of the most common issues you'll run into is having the wrong config strings, which basically means your app can't connect to the database 'cause the credentials or syntax are all jacked up.
Another pain in the butt is managing database sessions in Flask-SQLAlchemy. If you don't handle those properly, you could end up with database locks or delayed writes, and that's just a headache waiting to happen.
To avoid these kinds of problems, make sure you configure your database URIs correctly and double-check your settings.
Using context managers or the 'flask_sqlalchemy.SQLAlchemy.session' scopes can help you keep those session-related issues at bay.
Another thing that can really screw you over is underestimating database migrations.
Like, around 25% of Flask devs have had issues with this after deploying their apps. If you don't thoroughly test your new migrations, you might end up with database connection problems, and that's just a nightmare.
Using something like Flask-Migrate can automate the whole schema evolution process, which can help you avoid migration conflicts and keep your database structure consistent.
According to a DigitalOcean tutorial, setting up these kinds of tools from the get-go can save you a ton of trouble down the line when it comes to database integration issues.
And here's another pro tip: don't skimp on error handling.
If you don't have a solid way to catch and handle database errors, things can get really messy really fast. Implementing try-except blocks can help you deal with those errors gracefully, like they show in this DigitalOcean tutorial on error handling in Flask.
Combine that with the built-in error handling in Flask and extensions like SQLAlchemy, and you'll significantly reduce the frequency and impact of database-related errors.
Conclusion and Best Practices
(Up)If you're building a Flask app, you gotta have your database game on point. It's like the backbone of your whole project, and if you don't get it right, your app's gonna be as useful as a chocolate teapot.
Here's the lowdown on nailing that database integration:
- Get cozy with SQLAlchemy: This ORM is your best friend for interacting with databases in Flask. It turns those gnarly SQL commands into Python code that's way more readable, making your life a whole lot easier. Check it out here.
- Store data like a pro: Design your database schema properly, and you'll be retrieving and storing data like a boss. Normalization is key to avoiding redundant data, so don't slack on that.
- Protect your data: Use tools like Flask-Migrate to keep your data safe when updating your schema. Nucamp's Python-database integration guide has the deets on that.
- Keep it flexible: Separate your database access layer from your business logic. That way, you can test and modify things without everything going haywire. Check out our PostgreSQL optimization tips for more on that.
- Scale like a boss: Use database connection pools to handle varying loads without your app crapping out. This can seriously boost your Flask app's performance, which is crucial according to best practices for Flask API development.
Don't just take our word for it – stats show that apps with proper database schemas can see up to a 30% boost in query performance.
And using SQLAlchemy can save devs up to 40% of their time on database tasks, letting them focus on other parts of the app.
Bottom line: nail your database integration, and your Flask app will be unstoppable, handling user demands and scaling like a champ.
Industry experts agree that "the right database integration approach can make or break your Flask application's success." Use this as your checklist, and you'll be golden.
Frequently Asked Questions
(Up)What Database Management Systems (DBMS) can be integrated with Flask?
Flask can seamlessly integrate with various DBMS like SQLite, PostgreSQL, MySQL, and NoSQL options such as MongoDB.
What are the advantages of integrating a database with Flask?
Advantages include boosting application efficiency, expanding scalability, advancing data protection, and selecting suitable database systems for specific project objectives.
Which extensions can simplify data interactions in Flask applications?
Extensions like flask-sqlalchemy can simplify data interactions by providing a database abstraction layer, enhancing Flask's versatility in different project contexts.
What steps are involved in database integration in Flask?
Steps include leveraging SQLAlchemy for ORM capabilities, establishing database URI in app configurations, structuring database models, implementing Flask-Migrate for migrations, and orchestrating database operations following best practices.
What are some common pitfalls in database integration with Flask and their solutions?
Common pitfalls include incorrect configuration strings, improper handling of database sessions, underestimation of database migrations, and lack of proper error handling. Solutions involve ensuring correct settings, using context managers, testing migrations thoroughly, and implementing error handling practices.
You may be interested in the following topics as well:
Gain insights from case studies on Flask and understand why it's a go-to for professionals.
Learn about sustainable coding practices that ensure your Flask applications remain optimized over time.
Explore cybersecurity strategies for Flask to safeguard your user's data and trust.
Unlock the full potential of the web by mastering how to build RESTful APIs with Flask in a few simple steps.
Before you dive into the world of web applications, ensure you understand the essential Prerequisites for Flask deployment.
Empower your web development skills by learning to apply testing and debugging methodologies effectively in Flask.
Navigate the digital landscape with ease by learning about virtual paths in Flask, the key to connecting users to your content.
Get the full picture by examining the pros and cons of Django before diving into your next web application.
Discover the importance of Flask Extensions in modern web application development and see how they can streamline your projects.
Chevas Balloun
Director of Marketing & Brand
Chevas has spent over 15 years inventing brands, designing interfaces, and driving engagement for companies like Microsoft. He is a practiced writer, a productivity app inventor, board game designer, and has a builder-mentality drives entrepreneurship.